readable-stream
Advanced tools
Comparing version 1.1.13 to 2.0.0
@@ -1,22 +0,1 @@ | ||
// Copyright Joyent, Inc. and other Node contributors. | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a | ||
// copy of this software and associated documentation files (the | ||
// "Software"), to deal in the Software without restriction, including | ||
// without limitation the rights to use, copy, modify, merge, publish, | ||
// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
// persons to whom the Software is furnished to do so, subject to the | ||
// following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included | ||
// in all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
// a duplex stream is just a stream that is both readable and writable. | ||
@@ -27,5 +6,12 @@ // Since JS doesn't have multiple prototypal inheritance, this class | ||
'use strict'; | ||
module.exports = Duplex; | ||
/*<replacement>*/ | ||
var processNextTick = require('process-nextick-args'); | ||
/*</replacement>*/ | ||
/*<replacement>*/ | ||
var objectKeys = Object.keys || function (obj) { | ||
@@ -39,2 +25,3 @@ var keys = []; | ||
/*<replacement>*/ | ||
@@ -50,6 +37,8 @@ var util = require('core-util-is'); | ||
forEach(objectKeys(Writable.prototype), function(method) { | ||
var keys = objectKeys(Writable.prototype); | ||
for (var v = 0; v < keys.length; v++) { | ||
var method = keys[v]; | ||
if (!Duplex.prototype[method]) | ||
Duplex.prototype[method] = Writable.prototype[method]; | ||
}); | ||
} | ||
@@ -85,5 +74,9 @@ function Duplex(options) { | ||
// But allow more writes to happen in this tick. | ||
process.nextTick(this.end.bind(this)); | ||
processNextTick(onEndNT, this); | ||
} | ||
function onEndNT(self) { | ||
self.end(); | ||
} | ||
function forEach (xs, f) { | ||
@@ -90,0 +83,0 @@ for (var i = 0, l = xs.length; i < l; i++) { |
@@ -1,22 +0,1 @@ | ||
// Copyright Joyent, Inc. and other Node contributors. | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a | ||
// copy of this software and associated documentation files (the | ||
// "Software"), to deal in the Software without restriction, including | ||
// without limitation the rights to use, copy, modify, merge, publish, | ||
// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
// persons to whom the Software is furnished to do so, subject to the | ||
// following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included | ||
// in all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
// a passthrough stream. | ||
@@ -26,2 +5,4 @@ // basically just the most minimal sort of Transform stream. | ||
'use strict'; | ||
module.exports = PassThrough; | ||
@@ -28,0 +9,0 @@ |
@@ -1,21 +0,2 @@ | ||
// Copyright Joyent, Inc. and other Node contributors. | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a | ||
// copy of this software and associated documentation files (the | ||
// "Software"), to deal in the Software without restriction, including | ||
// without limitation the rights to use, copy, modify, merge, publish, | ||
// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
// persons to whom the Software is furnished to do so, subject to the | ||
// following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included | ||
// in all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
'use strict'; | ||
@@ -25,2 +6,7 @@ module.exports = Readable; | ||
/*<replacement>*/ | ||
var processNextTick = require('process-nextick-args'); | ||
/*</replacement>*/ | ||
/*<replacement>*/ | ||
var isArray = require('isarray'); | ||
@@ -44,5 +30,13 @@ /*</replacement>*/ | ||
var Stream = require('stream'); | ||
/*<replacement>*/ | ||
var Stream; | ||
(function (){try{ | ||
Stream = require('st' + 'ream'); | ||
}catch(_){Stream = require('events').EventEmitter;}}()) | ||
/*</replacement>*/ | ||
/*<replacement>*/ | ||
var util = require('core-util-is'); | ||
@@ -52,3 +46,2 @@ util.inherits = require('inherits'); | ||
var StringDecoder; | ||
@@ -65,2 +58,3 @@ | ||
var StringDecoder; | ||
@@ -74,6 +68,13 @@ util.inherits(Readable, Stream); | ||
// object stream flag. Used to make read(n) ignore n and to | ||
// make all the buffer merging and length checks go away | ||
this.objectMode = !!options.objectMode; | ||
if (stream instanceof Duplex) | ||
this.objectMode = this.objectMode || !!options.readableObjectMode; | ||
// the point at which it stops calling _read() to fill the buffer | ||
// Note: 0 is a valid value, means "don't call _read preemptively ever" | ||
var hwm = options.highWaterMark; | ||
var defaultHwm = options.objectMode ? 16 : 16 * 1024; | ||
var defaultHwm = this.objectMode ? 16 : 16 * 1024; | ||
this.highWaterMark = (hwm || hwm === 0) ? hwm : defaultHwm; | ||
@@ -105,10 +106,2 @@ | ||
// object stream flag. Used to make read(n) ignore n and to | ||
// make all the buffer merging and length checks go away | ||
this.objectMode = !!options.objectMode; | ||
if (stream instanceof Duplex) | ||
this.objectMode = this.objectMode || !!options.readableObjectMode; | ||
// Crypto is kind of old and crusty. Historically, its default string | ||
@@ -150,2 +143,5 @@ // encoding is 'binary' so we have to make this configurable. | ||
if (options && typeof options.read === 'function') | ||
this._read = options.read; | ||
Stream.call(this); | ||
@@ -161,3 +157,3 @@ } | ||
if (util.isString(chunk) && !state.objectMode) { | ||
if (!state.objectMode && typeof chunk === 'string') { | ||
encoding = encoding || state.defaultEncoding; | ||
@@ -179,2 +175,6 @@ if (encoding !== state.encoding) { | ||
Readable.prototype.isPaused = function() { | ||
return this._readableState.flowing === false; | ||
}; | ||
function readableAddChunk(stream, state, chunk, encoding, addToFront) { | ||
@@ -184,6 +184,5 @@ var er = chunkInvalid(state, chunk); | ||
stream.emit('error', er); | ||
} else if (util.isNullOrUndefined(chunk)) { | ||
} else if (chunk === null) { | ||
state.reading = false; | ||
if (!state.ended) | ||
onEofChunk(stream, state); | ||
onEofChunk(stream, state); | ||
} else if (state.objectMode || chunk && chunk.length > 0) { | ||
@@ -274,3 +273,3 @@ if (state.ended && !addToFront) { | ||
if (isNaN(n) || util.isNull(n)) { | ||
if (n === null || isNaN(n)) { | ||
// only flow one buffer at a time | ||
@@ -298,4 +297,5 @@ if (state.flowing && state.buffer.length) | ||
return 0; | ||
} else | ||
} else { | ||
return state.length; | ||
} | ||
} | ||
@@ -312,3 +312,3 @@ | ||
if (!util.isNumber(n) || n > 0) | ||
if (typeof n !== 'number' || n > 0) | ||
state.emittedReadable = false; | ||
@@ -401,3 +401,3 @@ | ||
if (util.isNull(ret)) { | ||
if (ret === null) { | ||
state.needReadable = true; | ||
@@ -418,3 +418,3 @@ n = 0; | ||
if (!util.isNull(ret)) | ||
if (ret !== null) | ||
this.emit('data', ret); | ||
@@ -427,5 +427,6 @@ | ||
var er = null; | ||
if (!util.isBuffer(chunk) && | ||
!util.isString(chunk) && | ||
!util.isNullOrUndefined(chunk) && | ||
if (!(Buffer.isBuffer(chunk)) && | ||
typeof chunk !== 'string' && | ||
chunk !== null && | ||
chunk !== undefined && | ||
!state.objectMode) { | ||
@@ -439,3 +440,4 @@ er = new TypeError('Invalid non-string/buffer chunk'); | ||
function onEofChunk(stream, state) { | ||
if (state.decoder && !state.ended) { | ||
if (state.ended) return; | ||
if (state.decoder) { | ||
var chunk = state.decoder.end(); | ||
@@ -463,5 +465,3 @@ if (chunk && chunk.length) { | ||
if (state.sync) | ||
process.nextTick(function() { | ||
emitReadable_(stream); | ||
}); | ||
processNextTick(emitReadable_, stream); | ||
else | ||
@@ -488,5 +488,3 @@ emitReadable_(stream); | ||
state.readingMore = true; | ||
process.nextTick(function() { | ||
maybeReadMore_(stream, state); | ||
}); | ||
processNextTick(maybeReadMore_, stream, state); | ||
} | ||
@@ -542,3 +540,3 @@ } | ||
if (state.endEmitted) | ||
process.nextTick(endFn); | ||
processNextTick(endFn); | ||
else | ||
@@ -738,7 +736,3 @@ src.once('end', endFn); | ||
if (!state.reading) { | ||
var self = this; | ||
process.nextTick(function() { | ||
debug('readable nexttick read 0'); | ||
self.read(0); | ||
}); | ||
processNextTick(nReadingNextTick, this); | ||
} else if (state.length) { | ||
@@ -754,2 +748,7 @@ emitReadable(this, state); | ||
function nReadingNextTick(self) { | ||
debug('readable nexttick read 0'); | ||
self.read(0); | ||
} | ||
// pause() and resume() are remnants of the legacy readable stream API | ||
@@ -762,6 +761,2 @@ // If the user uses them, then switch into old mode. | ||
state.flowing = true; | ||
if (!state.reading) { | ||
debug('resume read 0'); | ||
this.read(0); | ||
} | ||
resume(this, state); | ||
@@ -775,5 +770,3 @@ } | ||
state.resumeScheduled = true; | ||
process.nextTick(function() { | ||
resume_(stream, state); | ||
}); | ||
processNextTick(resume_, stream, state); | ||
} | ||
@@ -783,2 +776,7 @@ } | ||
function resume_(stream, state) { | ||
if (!state.reading) { | ||
debug('resume read 0'); | ||
stream.read(0); | ||
} | ||
state.resumeScheduled = false; | ||
@@ -834,4 +832,8 @@ stream.emit('resume'); | ||
chunk = state.decoder.write(chunk); | ||
if (!chunk || !state.objectMode && !chunk.length) | ||
// don't skip over falsy values in objectMode | ||
if (state.objectMode && (chunk === null || chunk === undefined)) | ||
return; | ||
else if (!state.objectMode && (!chunk || !chunk.length)) | ||
return; | ||
@@ -848,6 +850,6 @@ var ret = self.push(chunk); | ||
for (var i in stream) { | ||
if (util.isFunction(stream[i]) && util.isUndefined(this[i])) { | ||
if (this[i] === undefined && typeof stream[i] === 'function') { | ||
this[i] = function(method) { return function() { | ||
return stream[method].apply(stream, arguments); | ||
}}(i); | ||
}; }(i); | ||
} | ||
@@ -956,13 +958,15 @@ } | ||
state.ended = true; | ||
process.nextTick(function() { | ||
// Check that we didn't get one last unshift. | ||
if (!state.endEmitted && state.length === 0) { | ||
state.endEmitted = true; | ||
stream.readable = false; | ||
stream.emit('end'); | ||
} | ||
}); | ||
processNextTick(endReadableNT, state, stream); | ||
} | ||
} | ||
function endReadableNT(state, stream) { | ||
// Check that we didn't get one last unshift. | ||
if (!state.endEmitted && state.length === 0) { | ||
state.endEmitted = true; | ||
stream.readable = false; | ||
stream.emit('end'); | ||
} | ||
} | ||
function forEach (xs, f) { | ||
@@ -969,0 +973,0 @@ for (var i = 0, l = xs.length; i < l; i++) { |
@@ -1,23 +0,1 @@ | ||
// Copyright Joyent, Inc. and other Node contributors. | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a | ||
// copy of this software and associated documentation files (the | ||
// "Software"), to deal in the Software without restriction, including | ||
// without limitation the rights to use, copy, modify, merge, publish, | ||
// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
// persons to whom the Software is furnished to do so, subject to the | ||
// following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included | ||
// in all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
// a transform stream is a readable/writable stream where you do | ||
@@ -65,2 +43,4 @@ // something with the data. Sometimes it's called a "filter", | ||
'use strict'; | ||
module.exports = Transform; | ||
@@ -78,3 +58,3 @@ | ||
function TransformState(options, stream) { | ||
function TransformState(stream) { | ||
this.afterTransform = function(er, data) { | ||
@@ -102,3 +82,3 @@ return afterTransform(stream, er, data); | ||
if (!util.isNullOrUndefined(data)) | ||
if (data !== null && data !== undefined) | ||
stream.push(data); | ||
@@ -123,3 +103,3 @@ | ||
this._transformState = new TransformState(options, this); | ||
this._transformState = new TransformState(this); | ||
@@ -137,4 +117,12 @@ // when the writable side finishes, then flush out anything remaining. | ||
if (options) { | ||
if (typeof options.transform === 'function') | ||
this._transform = options.transform; | ||
if (typeof options.flush === 'function') | ||
this._flush = options.flush; | ||
} | ||
this.once('prefinish', function() { | ||
if (util.isFunction(this._flush)) | ||
if (typeof this._flush === 'function') | ||
this._flush(function(er) { | ||
@@ -187,3 +175,3 @@ done(stream, er); | ||
if (!util.isNull(ts.writechunk) && ts.writecb && !ts.transforming) { | ||
if (ts.writechunk !== null && ts.writecb && !ts.transforming) { | ||
ts.transforming = true; | ||
@@ -190,0 +178,0 @@ this._transform(ts.writechunk, ts.writeencoding, ts.afterTransform); |
@@ -1,22 +0,1 @@ | ||
// Copyright Joyent, Inc. and other Node contributors. | ||
// | ||
// Permission is hereby granted, free of charge, to any person obtaining a | ||
// copy of this software and associated documentation files (the | ||
// "Software"), to deal in the Software without restriction, including | ||
// without limitation the rights to use, copy, modify, merge, publish, | ||
// distribute, sublicense, and/or sell copies of the Software, and to permit | ||
// persons to whom the Software is furnished to do so, subject to the | ||
// following conditions: | ||
// | ||
// The above copyright notice and this permission notice shall be included | ||
// in all copies or substantial portions of the Software. | ||
// | ||
// THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS | ||
// OR IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF | ||
// MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN | ||
// NO EVENT SHALL THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, | ||
// DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR | ||
// OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE | ||
// USE OR OTHER DEALINGS IN THE SOFTWARE. | ||
// A bit simpler than readable streams. | ||
@@ -26,5 +5,12 @@ // Implement an async ._write(chunk, cb), and it'll handle all | ||
'use strict'; | ||
module.exports = Writable; | ||
/*<replacement>*/ | ||
var processNextTick = require('process-nextick-args'); | ||
/*</replacement>*/ | ||
/*<replacement>*/ | ||
var Buffer = require('buffer').Buffer; | ||
@@ -41,6 +27,16 @@ /*</replacement>*/ | ||
var Stream = require('stream'); | ||
/*<replacement>*/ | ||
var Stream; | ||
(function (){try{ | ||
Stream = require('st' + 'ream'); | ||
}catch(_){Stream = require('events').EventEmitter;}}()) | ||
/*</replacement>*/ | ||
util.inherits(Writable, Stream); | ||
function nop() {} | ||
function WriteReq(chunk, encoding, cb) { | ||
@@ -50,2 +46,3 @@ this.chunk = chunk; | ||
this.callback = cb; | ||
this.next = null; | ||
} | ||
@@ -58,9 +55,2 @@ | ||
// the point at which write() starts returning false | ||
// Note: 0 is a valid value, means that we always return false if | ||
// the entire buffer is not flushed immediately on write() | ||
var hwm = options.highWaterMark; | ||
var defaultHwm = options.objectMode ? 16 : 16 * 1024; | ||
this.highWaterMark = (hwm || hwm === 0) ? hwm : defaultHwm; | ||
// object stream flag to indicate whether or not this stream | ||
@@ -73,2 +63,9 @@ // contains buffers or objects. | ||
// the point at which write() starts returning false | ||
// Note: 0 is a valid value, means that we always return false if | ||
// the entire buffer is not flushed immediately on write() | ||
var hwm = options.highWaterMark; | ||
var defaultHwm = this.objectMode ? 16 : 16 * 1024; | ||
this.highWaterMark = (hwm || hwm === 0) ? hwm : defaultHwm; | ||
// cast to ints. | ||
@@ -129,3 +126,4 @@ this.highWaterMark = ~~this.highWaterMark; | ||
this.buffer = []; | ||
this.bufferedRequest = null; | ||
this.lastBufferedRequest = null; | ||
@@ -144,2 +142,19 @@ // number of pending user-supplied write callbacks | ||
WritableState.prototype.getBuffer = function writableStateGetBuffer() { | ||
var current = this.bufferedRequest; | ||
var out = []; | ||
while (current) { | ||
out.push(current); | ||
current = current.next; | ||
} | ||
return out; | ||
}; | ||
Object.defineProperty(WritableState.prototype, 'buffer', { | ||
get: require('util-deprecate')(function() { | ||
return this.getBuffer(); | ||
}, '_writableState.buffer is deprecated. Use ' + | ||
'_writableState.getBuffer() instead.') | ||
}); | ||
function Writable(options) { | ||
@@ -158,2 +173,10 @@ var Duplex = require('./_stream_duplex'); | ||
if (options) { | ||
if (typeof options.write === 'function') | ||
this._write = options.write; | ||
if (typeof options.writev === 'function') | ||
this._writev = options.writev; | ||
} | ||
Stream.call(this); | ||
@@ -168,9 +191,7 @@ } | ||
function writeAfterEnd(stream, state, cb) { | ||
function writeAfterEnd(stream, cb) { | ||
var er = new Error('write after end'); | ||
// TODO: defer error events consistently everywhere, not just the cb | ||
stream.emit('error', er); | ||
process.nextTick(function() { | ||
cb(er); | ||
}); | ||
processNextTick(cb, er); | ||
} | ||
@@ -185,11 +206,11 @@ | ||
var valid = true; | ||
if (!util.isBuffer(chunk) && | ||
!util.isString(chunk) && | ||
!util.isNullOrUndefined(chunk) && | ||
if (!(Buffer.isBuffer(chunk)) && | ||
typeof chunk !== 'string' && | ||
chunk !== null && | ||
chunk !== undefined && | ||
!state.objectMode) { | ||
var er = new TypeError('Invalid non-string/buffer chunk'); | ||
stream.emit('error', er); | ||
process.nextTick(function() { | ||
cb(er); | ||
}); | ||
processNextTick(cb, er); | ||
valid = false; | ||
@@ -204,3 +225,3 @@ } | ||
if (util.isFunction(encoding)) { | ||
if (typeof encoding === 'function') { | ||
cb = encoding; | ||
@@ -210,3 +231,3 @@ encoding = null; | ||
if (util.isBuffer(chunk)) | ||
if (chunk instanceof Buffer) | ||
encoding = 'buffer'; | ||
@@ -216,7 +237,7 @@ else if (!encoding) | ||
if (!util.isFunction(cb)) | ||
cb = function() {}; | ||
if (typeof cb !== 'function') | ||
cb = nop; | ||
if (state.ended) | ||
writeAfterEnd(this, state, cb); | ||
writeAfterEnd(this, cb); | ||
else if (validChunk(this, state, chunk, cb)) { | ||
@@ -246,3 +267,3 @@ state.pendingcb++; | ||
!state.bufferProcessing && | ||
state.buffer.length) | ||
state.bufferedRequest) | ||
clearBuffer(this, state); | ||
@@ -252,6 +273,17 @@ } | ||
Writable.prototype.setDefaultEncoding = function setDefaultEncoding(encoding) { | ||
// node::ParseEncoding() requires lower case. | ||
if (typeof encoding === 'string') | ||
encoding = encoding.toLowerCase(); | ||
if (!(['hex', 'utf8', 'utf-8', 'ascii', 'binary', 'base64', | ||
'ucs2', 'ucs-2','utf16le', 'utf-16le', 'raw'] | ||
.indexOf((encoding + '').toLowerCase()) > -1)) | ||
throw new TypeError('Unknown encoding: ' + encoding); | ||
this._writableState.defaultEncoding = encoding; | ||
}; | ||
function decodeChunk(state, chunk, encoding) { | ||
if (!state.objectMode && | ||
state.decodeStrings !== false && | ||
util.isString(chunk)) { | ||
typeof chunk === 'string') { | ||
chunk = new Buffer(chunk, encoding); | ||
@@ -267,3 +299,4 @@ } | ||
chunk = decodeChunk(state, chunk, encoding); | ||
if (util.isBuffer(chunk)) | ||
if (chunk instanceof Buffer) | ||
encoding = 'buffer'; | ||
@@ -279,6 +312,13 @@ var len = state.objectMode ? 1 : chunk.length; | ||
if (state.writing || state.corked) | ||
state.buffer.push(new WriteReq(chunk, encoding, cb)); | ||
else | ||
if (state.writing || state.corked) { | ||
var last = state.lastBufferedRequest; | ||
state.lastBufferedRequest = new WriteReq(chunk, encoding, cb); | ||
if (last) { | ||
last.next = state.lastBufferedRequest; | ||
} else { | ||
state.bufferedRequest = state.lastBufferedRequest; | ||
} | ||
} else { | ||
doWrite(stream, state, false, len, chunk, encoding, cb); | ||
} | ||
@@ -301,11 +341,7 @@ return ret; | ||
function onwriteError(stream, state, sync, er, cb) { | ||
--state.pendingcb; | ||
if (sync) | ||
process.nextTick(function() { | ||
state.pendingcb--; | ||
cb(er); | ||
}); | ||
else { | ||
state.pendingcb--; | ||
processNextTick(cb, er); | ||
else | ||
cb(er); | ||
} | ||
@@ -334,3 +370,3 @@ stream._writableState.errorEmitted = true; | ||
// Check if we're actually ready to finish, but don't emit yet | ||
var finished = needFinish(stream, state); | ||
var finished = needFinish(state); | ||
@@ -340,3 +376,3 @@ if (!finished && | ||
!state.bufferProcessing && | ||
state.buffer.length) { | ||
state.bufferedRequest) { | ||
clearBuffer(stream, state); | ||
@@ -346,5 +382,3 @@ } | ||
if (sync) { | ||
process.nextTick(function() { | ||
afterWrite(stream, state, finished, cb); | ||
}); | ||
processNextTick(afterWrite, stream, state, finished, cb); | ||
} else { | ||
@@ -378,8 +412,13 @@ afterWrite(stream, state, finished, cb); | ||
state.bufferProcessing = true; | ||
var entry = state.bufferedRequest; | ||
if (stream._writev && state.buffer.length > 1) { | ||
if (stream._writev && entry && entry.next) { | ||
// Fast case, write everything using _writev() | ||
var buffer = []; | ||
var cbs = []; | ||
for (var c = 0; c < state.buffer.length; c++) | ||
cbs.push(state.buffer[c].callback); | ||
while (entry) { | ||
cbs.push(entry.callback); | ||
buffer.push(entry); | ||
entry = entry.next; | ||
} | ||
@@ -389,3 +428,4 @@ // count the one we are adding, as well. | ||
state.pendingcb++; | ||
doWrite(stream, state, true, state.length, state.buffer, '', function(err) { | ||
state.lastBufferedRequest = null; | ||
doWrite(stream, state, true, state.length, buffer, '', function(err) { | ||
for (var i = 0; i < cbs.length; i++) { | ||
@@ -398,7 +438,5 @@ state.pendingcb--; | ||
// Clear buffer | ||
state.buffer = []; | ||
} else { | ||
// Slow case, write chunks one-by-one | ||
for (var c = 0; c < state.buffer.length; c++) { | ||
var entry = state.buffer[c]; | ||
while (entry) { | ||
var chunk = entry.chunk; | ||
@@ -410,3 +448,3 @@ var encoding = entry.encoding; | ||
doWrite(stream, state, false, len, chunk, encoding, cb); | ||
entry = entry.next; | ||
// if we didn't call the onwrite immediately, then | ||
@@ -417,3 +455,2 @@ // it means that we need to wait until it does. | ||
if (state.writing) { | ||
c++; | ||
break; | ||
@@ -423,8 +460,6 @@ } | ||
if (c < state.buffer.length) | ||
state.buffer = state.buffer.slice(c); | ||
else | ||
state.buffer.length = 0; | ||
if (entry === null) | ||
state.lastBufferedRequest = null; | ||
} | ||
state.bufferedRequest = entry; | ||
state.bufferProcessing = false; | ||
@@ -435,3 +470,2 @@ } | ||
cb(new Error('not implemented')); | ||
}; | ||
@@ -444,7 +478,7 @@ | ||
if (util.isFunction(chunk)) { | ||
if (typeof chunk === 'function') { | ||
cb = chunk; | ||
chunk = null; | ||
encoding = null; | ||
} else if (util.isFunction(encoding)) { | ||
} else if (typeof encoding === 'function') { | ||
cb = encoding; | ||
@@ -454,3 +488,3 @@ encoding = null; | ||
if (!util.isNullOrUndefined(chunk)) | ||
if (chunk !== null && chunk !== undefined) | ||
this.write(chunk, encoding); | ||
@@ -470,5 +504,6 @@ | ||
function needFinish(stream, state) { | ||
function needFinish(state) { | ||
return (state.ending && | ||
state.length === 0 && | ||
state.bufferedRequest === null && | ||
!state.finished && | ||
@@ -486,3 +521,3 @@ !state.writing); | ||
function finishMaybe(stream, state) { | ||
var need = needFinish(stream, state); | ||
var need = needFinish(state); | ||
if (need) { | ||
@@ -493,4 +528,5 @@ if (state.pendingcb === 0) { | ||
stream.emit('finish'); | ||
} else | ||
} else { | ||
prefinish(stream, state); | ||
} | ||
} | ||
@@ -505,3 +541,3 @@ return need; | ||
if (state.finished) | ||
process.nextTick(cb); | ||
processNextTick(cb); | ||
else | ||
@@ -508,0 +544,0 @@ stream.once('finish', cb); |
{ | ||
"name": "readable-stream", | ||
"version": "1.1.13", | ||
"description": "Streams3, a user-land copy of the stream library from Node.js v0.11.x", | ||
"version": "2.0.0", | ||
"description": "Streams3, a user-land copy of the stream library from iojs v2.x", | ||
"main": "readable.js", | ||
"dependencies": { | ||
"core-util-is": "~1.0.0", | ||
"process-nextick-args": "~1.0.0", | ||
"inherits": "~2.0.1", | ||
"isarray": "0.0.1", | ||
"string_decoder": "~0.10.x", | ||
"inherits": "~2.0.1" | ||
"util-deprecate": "~1.0.1" | ||
}, | ||
@@ -16,7 +18,7 @@ "devDependencies": { | ||
"scripts": { | ||
"test": "tap test/simple/*.js" | ||
"test": "tap test/parallel/*.js" | ||
}, | ||
"repository": { | ||
"type": "git", | ||
"url": "git://github.com/isaacs/readable-stream" | ||
"url": "git://github.com/nodejs/readable-stream" | ||
}, | ||
@@ -31,4 +33,3 @@ "keywords": [ | ||
}, | ||
"author": "Isaac Z. Schlueter <i@izs.me> (http://blog.izs.me/)", | ||
"license": "MIT" | ||
} |
@@ -0,3 +1,7 @@ | ||
(function (){ | ||
try { | ||
exports.Stream = require('st' + 'ream'); // hack to fix a circular dependency issue when used with browserify | ||
} catch(_){} | ||
}()); | ||
exports = module.exports = require('./lib/_stream_readable.js'); | ||
exports.Stream = require('stream'); | ||
exports.Readable = exports; | ||
@@ -4,0 +8,0 @@ exports.Writable = require('./lib/_stream_writable.js'); |
@@ -5,12 +5,31 @@ # readable-stream | ||
[](https://travis-ci.org/nodejs/readable-stream) | ||
[](https://nodei.co/npm/readable-stream/) | ||
[](https://nodei.co/npm/readable-stream/) | ||
[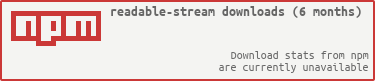](https://nodei.co/npm/readable-stream/) | ||
This package is a mirror of the Streams2 and Streams3 implementations in Node-core. | ||
```bash | ||
npm install --save readable-stream | ||
``` | ||
If you want to guarantee a stable streams base, regardless of what version of Node you, or the users of your libraries are using, use **readable-stream** *only* and avoid the *"stream"* module in Node-core. | ||
***Node-core streams for userland*** | ||
**readable-stream** comes in two major versions, v1.0.x and v1.1.x. The former tracks the Streams2 implementation in Node 0.10, including bug-fixes and minor improvements as they are added. The latter tracks Streams3 as it develops in Node 0.11; we will likely see a v1.2.x branch for Node 0.12. | ||
This package is a mirror of the Streams2 and Streams3 implementations in | ||
Node-core. | ||
**readable-stream** uses proper patch-level versioning so if you pin to `"~1.0.0"` you’ll get the latest Node 0.10 Streams2 implementation, including any fixes and minor non-breaking improvements. The patch-level versions of 1.0.x and 1.1.x should mirror the patch-level versions of Node-core releases. You should prefer the **1.0.x** releases for now and when you’re ready to start using Streams3, pin to `"~1.1.0"` | ||
If you want to guarantee a stable streams base, regardless of what version of | ||
Node you, or the users of your libraries are using, use **readable-stream** *only* and avoid the *"stream"* module in Node-core. | ||
As of version 2.0.0 **readable-stream** uses semantic versioning. | ||
# Streams WG Team Members | ||
* **Chris Dickinson** ([@chrisdickinson](https://github.com/chrisdickinson)) <christopher.s.dickinson@gmail.com> | ||
- Release GPG key: 9554F04D7259F04124DE6B476D5A82AC7E37093B | ||
* **Calvin Metcalf** ([@calvinmetcalf](https://github.com/calvinmetcalf)) $lt;calvin.metcalf@gmail.com@gt; | ||
- Release GPG key: F3EF5F62A87FC27A22E643F714CE4FF5015AA242 | ||
* **Rod Vagg** ([@rvagg](https://github.com/rvagg)) <rod@vagg.org> | ||
- Release GPG key: DD8F2338BAE7501E3DD5AC78C273792F7D83545D | ||
* **Sam Newman** ([@sonewman](https://github.com/sonewman)) <newmansam@outlook.com> | ||
* **Mathias Buus** ([@mafintosh](https://github.com/mafintosh)) <mathiasbuus@gmail.com> | ||
* **Domenic Denicola** ([@domenic](https://github.com/domenic)) <d@domenic.me> |
New author
Supply chain riskA new npm collaborator published a version of the package for the first time. New collaborators are usually benign additions to a project, but do indicate a change to the security surface area of a package.
Found 1 instance in 1 package
Dynamic require
Supply chain riskDynamic require can indicate the package is performing dangerous or unsafe dynamic code execution.
Found 1 instance in 1 package
No contributors or author data
MaintenancePackage does not specify a list of contributors or an author in package.json.
Found 1 instance in 1 package
16
35
54210
6
1465
2
3
1
+ Addedprocess-nextick-args@~1.0.0
+ Addedutil-deprecate@~1.0.1
+ Addedprocess-nextick-args@1.0.7(transitive)
+ Addedutil-deprecate@1.0.2(transitive)