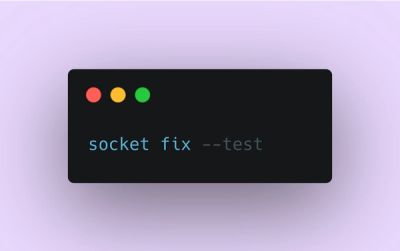
Product
Introducing Socket Fix for Safe, Automated Dependency Upgrades
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Reakit is a toolkit for building accessible web apps with React. It provides a set of low-level components and hooks that can be used to create custom, accessible UI components.
Accessible Dialog
This code demonstrates how to create an accessible dialog using Reakit. The `useDialogState` hook manages the state of the dialog, and the `Dialog` and `DialogDisclosure` components are used to render the dialog and the button that opens it.
import React from 'react';
import { useDialogState, Dialog, DialogDisclosure } from 'reakit/Dialog';
function App() {
const dialog = useDialogState();
return (
<>
<DialogDisclosure {...dialog}>Open Dialog</DialogDisclosure>
<Dialog {...dialog} aria-label="Welcome">
<p>Welcome to Reakit Dialog!</p>
<button onClick={dialog.hide}>Close</button>
</Dialog>
</>
);
}
export default App;
Accessible Menu
This code demonstrates how to create an accessible menu using Reakit. The `useMenuState` hook manages the state of the menu, and the `Menu`, `MenuItem`, and `MenuButton` components are used to render the menu and its items.
import React from 'react';
import { useMenuState, Menu, MenuItem, MenuButton } from 'reakit/Menu';
function App() {
const menu = useMenuState();
return (
<>
<MenuButton {...menu}>Menu</MenuButton>
<Menu {...menu} aria-label="Preferences">
<MenuItem {...menu}>Profile</MenuItem>
<MenuItem {...menu}>Settings</MenuItem>
<MenuItem {...menu}>Logout</MenuItem>
</Menu>
</>
);
}
export default App;
Accessible Tabs
This code demonstrates how to create accessible tabs using Reakit. The `useTabState` hook manages the state of the tabs, and the `Tab`, `TabList`, and `TabPanel` components are used to render the tabs and their content.
import React from 'react';
import { useTabState, Tab, TabList, TabPanel } from 'reakit/Tab';
function App() {
const tab = useTabState();
return (
<>
<TabList {...tab} aria-label="My tabs">
<Tab {...tab}>Tab 1</Tab>
<Tab {...tab}>Tab 2</Tab>
<Tab {...tab}>Tab 3</Tab>
</TabList>
<TabPanel {...tab}>Content 1</TabPanel>
<TabPanel {...tab}>Content 2</TabPanel>
<TabPanel {...tab}>Content 3</TabPanel>
</>
);
}
export default App;
React Aria is a library of React hooks that provides accessible UI primitives. It is part of Adobe's React Spectrum project. React Aria focuses on providing low-level hooks for building accessible components, similar to Reakit, but with a broader range of accessibility features.
Chakra UI is a simple, modular, and accessible component library that gives you the building blocks you need to build your React applications. It provides a set of high-level components with built-in accessibility, making it easier to build accessible UIs compared to Reakit's low-level approach.
Toolkit for building accessible rich web apps with React.
Explore website Β»
By donating $100 or more you become a sponsor and help in the development of this project. Thank you to all our sponsors! π
By donating $5 or more you become a backer and help in the development of this project. Thank you to all our backers! π
npm:
npm i reakit
Yarn:
yarn add reakit
Thanks to @nosebit for the package name on npm.
import React from "react";
import ReactDOM from "react-dom";
import { useDialogState, Dialog, DialogDisclosure } from "reakit";
function App() {
const dialog = useDialogState();
return (
<div>
<DialogDisclosure {...dialog}>Open dialog</DialogDisclosure>
<Dialog {...dialog} aria-label="Welcome">
Welcome to Reakit!
</Dialog>
</div>
);
}
ReactDOM.render(<App />, document.getElementById("root"));
Play with this on CodeSandbox and read the documentation to learn more.
This project exists thanks to all the people who contribute. Learn more on the contributing guide.
Logo by Leonardo Elias.
MIT Β© Diego Haz
FAQs
Toolkit for building accessible rich web apps with React
The npm package reakit receives a total of 107,833 weekly downloads. As such, reakit popularity was classified as popular.
We found that reakit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Β It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Automatically fix and test dependency updates with socket fixβa new CLI tool that turns CVE alerts into safe, automated upgrades.
Security News
CISA denies CVE funding issues amid backlash over a new CVE foundation formed by board members, raising concerns about transparency and program governance.
Product
Weβre excited to announce a powerful new capability in Socket: historical data and enhanced analytics.