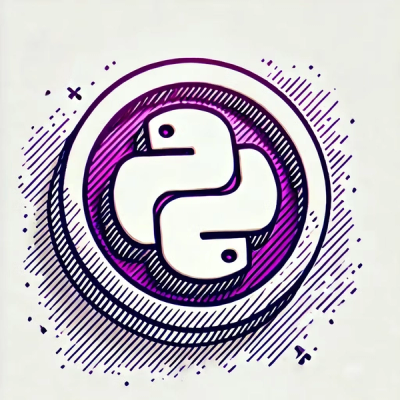
Product
Socket Now Supports pylock.toml Files
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
reddit-wrapper-v2
Advanced tools
Simple Reddit API framework for nodejs. Replacing the no longer updated reddit-snooper. Relying more on promises rather than callbacks. Nicely handles multiple reddit errors.
Reddit-Wrapper is designed to be a simple to user reddit API wrapper, while also providing robust error handling and retry capabilities. Every function returns a promise. Allowing the user to easily handle errors in the catch, and results in the then.
Now with TypeScript definitions! (Thanks @yliaho)
This wrapper also has a lot of error handling functionality. As Reddit sometimes errors out in strange ways (random 403's, "You are doing this too much...") handling it within this wrapper allows users to focus on non reddit functionality. Find below all the error handling strategies you can opt into. By default no error handling options are enabled except for automatic 403 retries.
Simple API usage:
reddit.api.get("/subreddits/mine/subscriber", {
limit: 2,
})
.then(function(response) {
let responseCode = response[0];
let responseData = response[1];
console.log("Received response (" + responseCode + "): ", responseData);
})
.catch(function(err) {
console.log("Reddit Error: ", err);
});
npm install reddit-wrapper-v2 --save
var RedditAPI = require('reddit-wrapper-v2');
var redditConn = new RedditAPI({
// Options for Reddit Wrapper
username: 'reddit_username',
password: 'reddit password',
app_id: 'reddit api app id',
api_secret: 'reddit api secret',
user_agent: 'user agent for your bot',
retry_on_wait: true,
retry_on_server_error: 5,
retry_delay: 1,
logs: true
});
Option Name | Option Default | Description | Required |
---|---|---|---|
username | null | The Username for the Reddit Account. | True |
password | null | The Password for the Reddit Account. | True |
app_id | null | The Reddit Application ID. | True |
api_secret | null | The Reddit Application Secret. | True |
logs | False | Display logs. | False |
user_agent | Reddit-Watcher-V2 | The User Agent for all Reddit API requests. | False |
retry_on_wait | False | If True and Reddit returns a "You are trying this too much" error, it will pause the process for the exact time needed, then retry the request. | False |
retry_on_server_error | 0 | If > 0 and Reddit returns a server error (responseCode >= 500 && responseCode <= 599) it will retry the request the number of times you specify + 1 automatically. | False |
retry_delay | 5 sec. | Specifies the retry delay for server error retries. (IE. if server error and you specify you want to retry before retrying it will delay for retry_delay seconds.) | False |
All you need to get up and running is obtain an api_id and an api_secret. Both can be created on the Reddit app console
The API component is an agnostic wrapper around Reddit's rest API that handles retries, and Reddit's different response codes.
In order to use the api head over to the Reddit API Documentation. All of the api methods use one of the 5 HTTP methods (GET, POST, PATCH, PUT, DELETE) which map to the 5 different redditAPI.api methods.
// endpoint: api endpoint ex: 'api/v1/me' or '/api/v1/me/karma/' (listed on api documentation)
// data: JSON data, dependent on the request which is specified in the docs
// NOTE: the function .get is used for api calls that use HTTP GET, you can find the method each api endpiont uses on (you guessed it) the reddit api docs
// HTTP GET
redditWrapper.api.get(endpoint, data)
.then(function(response) {
let responseCode = response[0];
let responseData = response[1];
console.log("Received response (" + responseCode + "): ", responseData);
})
.catch(function(err) {
return console.error("api request failed: " + err)
});
// HTTP POST
redditWrapper.api.post(endpoint, data)
.then(function(response) {})
.catch(function(err) {})
// HTTP PATCH
redditWrapper.api.patch(endpoint, data)
.then(function(response) {})
.catch(function(err) {})
// HTTP PUT
redditWrapper.api.put(endpoint, data)
.then(function(response) {})
.catch(function(err) {})
// HTTP DELETE
redditWrapper.api.delete(endpoint, data)
.then(function(response) {})
.catch(function(err) {})
// gets an api token
redditWrapper.api.get_token()
.then(function(token) {})
.catch(function(err) {})
Note: new accounts get little to no posting privileges (1 comment or post per 5 minutes or more) if you dont have any karma. If you just want to play around with the api I recommend using an active account.
Note: the response from any HTTP methods will be an array containing [responseCode, data].
Note: Any 403 that occurs during a retry process will cause a token refresh and a endpoint retry.
check how much karma your bot has
redditWrapper.api.get('api/v1/me/karma', {})
.then(function(resp) {
let responseCode = response[0];
let responseData = response[1];
console.log("I have " + responseData.karma + " karma")
})
.catch(function(err) {
console.log("Error getting karma: ", err);
})
post a comment
redditWrapper.api.post("/api/comment", {
api_type: "json",
text: "Hello World"
thing_id: comment.data.name
})
.then(function(resp) {
let responseCode = response[0];
let responseData = response[1];
console.log("Posted comment!");
})
.catch(function(err) {
console.log("Error posting comment: ", err);
})
FAQs
Simple Reddit API framework for nodejs. Replacing the no longer updated reddit-snooper. Relying more on promises rather than callbacks. Nicely handles multiple reddit errors.
The npm package reddit-wrapper-v2 receives a total of 8 weekly downloads. As such, reddit-wrapper-v2 popularity was classified as not popular.
We found that reddit-wrapper-v2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.
Research
Security News
Malicious Ruby gems typosquat Fastlane plugins to steal Telegram bot tokens, messages, and files, exploiting demand after Vietnam’s Telegram ban.