regexp-manager
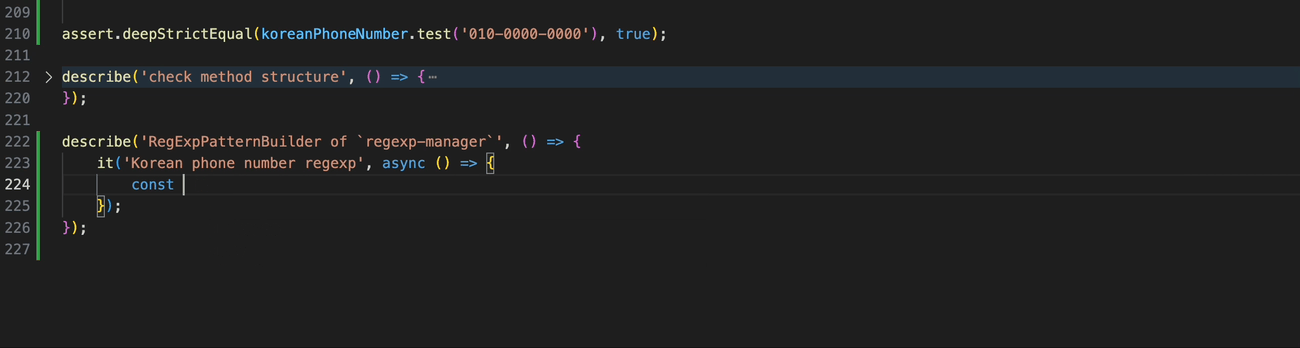
$ npm i regexp-manager
This library creates regular expressions in a form similar to query builders. The benefits of this library are three.
- To intuitively use various methods of regular expressions that developers do not usually deal with
- To increase the reuse of regular expressions
- To infer regular expressions at the type level and allow problems to be found at the time of compilation
Methods currently implemented
const leftOrRight = new RegExpPatternBuilder('left').or('right').expression;
const leftAndRight = new RegExpPatternBuilder('left').and('right').expression;
const capturingA = new RegExpPatternBuilder('').capturing('A').expression;
const lessThanTen = new RegExpPatternBuilder('a').lessThan(10).expression;
const lessThanOrEqualTen = new RegExpPatternBuilder('a').lessThanOrEqual(10).expression;
const moreThanThree = new RegExpPatternBuilder('a').moreThan(3).expression;
const moreThanOrEqualThree = new RegExpPatternBuilder('a').moreThanOrEqual(3).expression;
const lookhehind = new RegExpPatternBuilder('b').includes('LEFT', 'a');
const lookahead = new RegExpPatternBuilder('b').includes('RIGHT', 'a');
const nagativeLookbhind = new RegExpPatternBuilder('b').excludes('LEFT', 'a');
const negativeLookahead = new RegExpPatternBuilder('b').excludes('RIGHT', 'a');
How to use a builder
import { RegExpPatternBuilder } from 'regexp-manager';
const koreanPhoneNumber = new RegExpPatternBuilder('')
.capturing(() => new RegExpPatternBuilder('010').or('011'))
.and(() => new RegExpPatternBuilder('[0-9]').between(3, 4))
.and(() => new RegExpPatternBuilder('[0-9]').between(4, 4)).expression;
If you write a function that returns the string or additional builder according to the inferred type, you can check the result at the time of compilation.