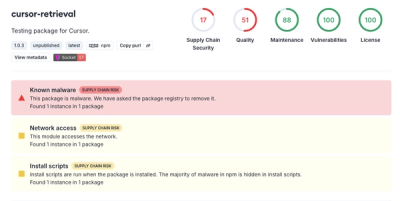
Security News
The Risks of Misguided Research in Supply Chain Security
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
responselike
Advanced tools
The `responselike` npm package is designed to create `Response` objects that mimic HTTP response objects. This can be particularly useful in testing scenarios where you want to simulate responses from web servers without making actual HTTP requests. It allows for the creation of response-like objects with properties and methods that resemble those of real HTTP responses.
Creating a response-like object
This feature allows for the creation of a response-like object with a status code, headers, body, and URL. It's useful for simulating HTTP responses in tests.
const Response = require('responselike');
const response = new Response(200, {}, 'body', 'http://example.com');
console.log(response.statusCode); // 200
Accessing response properties
Once a response-like object is created, you can access its properties such as headers, body, and URL, similar to how you would with a real HTTP response.
console.log(response.headers); // {}
console.log(response.body); // 'body'
console.log(response.url); // 'http://example.com'
Nock is a powerful HTTP server mocking and expectations library for Node.js. Unlike `responselike`, which is focused on creating response-like objects, nock allows you to intercept HTTP requests and provide predefined responses. It's more comprehensive for testing HTTP interactions.
This package provides a way to mock HTTP requests and responses in Node.js, similar to `responselike`. However, `node-mocks-http` is more focused on providing a complete set of tools for mocking the `http` module, including both request and response objects, making it more suitable for integration testing.
A response-like object for mocking a Node.js HTTP response stream
Returns a streamable response object similar to a Node.js HTTP response stream. Useful for formatting cached responses so they can be consumed by code expecting a real response.
npm install responselike
import Response from 'responselike';
const response = new Response({
statusCode: 200,
headers: {
foo: 'bar'
},
body: Buffer.from('Hi!'),
url: 'https://example.com'
});
response.statusCode;
// 200
response.headers;
// {foo: 'bar'}
response.body;
// <Buffer 48 69 21>
response.url;
// 'https://example.com'
response.pipe(process.stdout);
// 'Hi!'
Returns a streamable response object similar to a Node.js HTTP response stream.
Type: object
Type: number
The HTTP response status code.
Type: object
The HTTP headers. Keys will be automatically lowercased.
Type: Buffer
The response body. The Buffer contents will be streamable but is also exposed directly as response.body
.
Type: string
The request URL string.
FAQs
A response-like object for mocking a Node.js HTTP response stream
We found that responselike demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Snyk's use of malicious npm packages for research raises ethical concerns, highlighting risks in public deployment, data exfiltration, and unauthorized testing.
Research
Security News
Socket researchers found several malicious npm packages typosquatting Chalk and Chokidar, targeting Node.js developers with kill switches and data theft.
Security News
pnpm 10 blocks lifecycle scripts by default to improve security, addressing supply chain attack risks but sparking debate over compatibility and workflow changes.