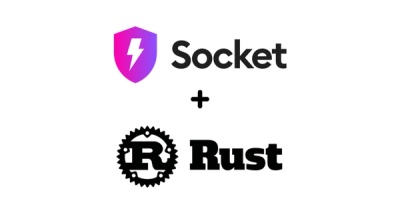
Product
Introducing Rust Support in Socket
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
rollup-plugin-peer-deps-external
Advanced tools
Rollup plugin to automatically add a library's peerDependencies to its bundle's external config.
The rollup-plugin-peer-deps-external package is a Rollup plugin that automatically marks peer dependencies as external. This helps to avoid bundling peer dependencies into your package, ensuring that they are resolved from the consuming project's dependencies.
Automatically Externalize Peer Dependencies
This feature automatically marks all peer dependencies listed in your package.json as external, preventing them from being bundled into your output file. This is useful for library authors who want to ensure that peer dependencies are resolved from the consuming project's dependencies.
const peerDepsExternal = require('rollup-plugin-peer-deps-external');
module.exports = {
input: 'src/index.js',
output: {
file: 'dist/bundle.js',
format: 'cjs'
},
plugins: [
peerDepsExternal()
]
};
The rollup-plugin-auto-external package automatically marks dependencies and peer dependencies as external. It provides more flexibility by allowing you to configure which dependencies to externalize. Compared to rollup-plugin-peer-deps-external, it offers a broader scope by including regular dependencies as well.
The rollup-plugin-node-externals package is another Rollup plugin that marks Node.js built-in modules and dependencies as external. It is more comprehensive in scope, as it can handle built-in modules, dependencies, and peer dependencies. This makes it a good choice for Node.js projects that need to externalize a wide range of modules.
Automatically externalize peerDependencies
in a rollup
bundle.
When bundling a library using rollup
, we generally want to keep from including peerDependencies
since they are expected to be provided by the consumer of the library. By excluding these dependencies, we keep bundle size down and avoid bundling duplicate dependencies.
We can achieve this using the rollup external
configuration option, providing it a list of the peer dependencies to exclude from the bundle. This plugin automates the process, automatically adding a library's peerDependencies
to the external
configuration.
npm install --save-dev rollup-plugin-peer-deps-external
// Add to plugins array in rollup.config.js
import peerDepsExternal from 'rollup-plugin-peer-deps-external';
export default {
plugins: [
// Preferably set as first plugin.
peerDepsExternal(),
],
}
If your package.json
is not in the current working directory you can specify the path to the file
// Add to plugins array in rollup.config.js
import peerDepsExternal from 'rollup-plugin-peer-deps-external';
export default {
plugins: [
// Preferably set as first plugin.
peerDepsExternal({
packageJsonPath: 'my/folder/package.json'
}),
],
}
Set includeDependencies
to true
to also externalize regular dependencies in addition to peer deps.
// Add to plugins array in rollup.config.js
import peerDepsExternal from 'rollup-plugin-peer-deps-external';
export default {
plugins: [
// Preferably set as first plugin.
peerDepsExternal({
includeDependencies: true,
}),
],
}
This plugin is compatible with module path format applied by, for example, babel-plugin-lodash
. For any module name in peerDependencies
, all paths beginning with that module name will also be added to external
.
E.g.: If lodash
is in peerDependencies
, an import of lodash/map
would be added to externals.
FAQs
Rollup plugin to automatically add a library's peerDependencies to its bundle's external config.
We found that rollup-plugin-peer-deps-external demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket now supports Rust and Cargo, offering package search for all users and experimental SBOM generation for enterprise projects.
Product
Socket’s precomputed reachability slashes false positives by flagging up to 80% of vulnerabilities as irrelevant, with no setup and instant results.
Product
Socket is launching experimental protection for Chrome extensions, scanning for malware and risky permissions to prevent silent supply chain attacks.