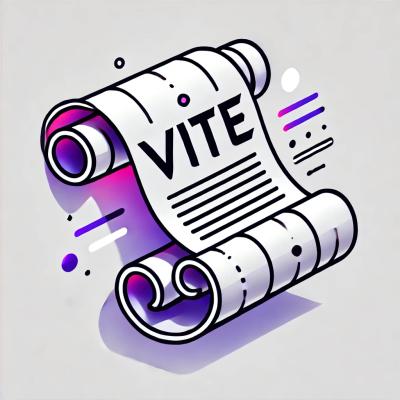
Security News
Vite Releases Technical Preview of Rolldown-Vite, a Rust-Based Bundler
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
A powerful and modular Node.js database library for managing dynamic models, transactions, caching, RBAC, GraphQL, and more.
ScorpDB is a powerful, modular Node.js database library designed to handle complex database operations with ease. It comes with features such as dynamic model management, transaction handling, caching, RBAC (Role-Based Access Control), GraphQL integration, health checks, and much more. ScorpDB is perfect for developers looking to build scalable, secure, and maintainable applications.
Install ScorpDB via npm:
npm install scorpdb
npm install scorpdb -g
Setup
Create a scorp.config.json file in your project root to define your database configuration:
// file: scorp.connect.json
{
"database": {
"host": "localhost",
"port": 3306,
"user": "root",
"password": "your-password",
"database": "your-database"
}
}
- scorp help Display a list of available commands.
- scorp install Setup the ScorpDB module and configuration file.
- scorp config view Display the current database configuration.
- scorp config reset Reset the database configuration file.
- scorp npm update Update ScorpDB to the latest version.
Usage
Import the Library
const ScorpDB = require('scorpdb');
Database Connection
Connect to the database:
(async () => {
try {
await ScorpDB.Database.connect();
console.log('Database connected!');
} catch (error) {
console.error('Connection error:', error.message);
}
})();
Disconnect from the database:
await ScorpDB.Database.disconnect();
Dynamic Models
Define and use models for database operations:
const userModel = new ScorpDB.Model('users');
// Fetch all users
const users = await userModel.findAll();
console.log(users);
// Insert a new user
await userModel.insert({ name: 'John Doe', email: 'john@example.com' });
// Update a user
await userModel.update(1, { name: 'John Smith' });
// Delete a user
await userModel.delete(1);
Transactions
Handle database transactions:
const transaction = ScorpDB.Transaction;
await transaction.start();
try {
await ScorpDB.Database.query('INSERT INTO accounts (balance) VALUES (?)', [100]);
await transaction.commit();
console.log('Transaction committed.');
} catch (error) {
await transaction.rollback();
console.error('Transaction rolled back:', error.message);
}
Caching
Use Redis for caching:
await ScorpDB.Cache.set('key', 'value');
const value = await ScorpDB.Cache.get('key');
console.log('Cached value:', value);
RBAC (Role-Based Access Control)
Define roles and permissions:
ScorpDB.RBAC.addRole('admin', ['create', 'read', 'update', 'delete']);
const access = ScorpDB.RBAC.hasAccess('admin', 'create');
console.log('Admin access to create:', access);
GraphQL Integration
Query your database using GraphQL:
const query = `
{
hello
}
`;
const result = await ScorpDB.GraphQL.executeQuery(query);
console.log(result);
Health Checks
Monitor the health of the database and caching systems:
const status = await ScorpDB.HealthCheck.getStatus();
console.log('System Health:', status);
Scheduler
Schedule recurring tasks:
ScorpDB.Scheduler.schedule('taskName', '0 3 * * *', () => {
console.log('Task executed at 3 AM!');
});
Backup and Restore
Back up and restore your database:
ScorpDB.Backup.backup('backup.sql');
ScorpDB.Backup.restore('backup.sql');
AI-Based Query Optimization
Optimize your SQL queries using AI:
const originalQuery = 'SELECT * FROM users WHERE email LIKE "%@example.com"';
const optimizedQuery = await ScorpDB.AIOptimizer.optimizeQuery(originalQuery);
console.log('Optimized Query:', optimizedQuery);
Job Queue
Manage background tasks:
const emailQueue = new ScorpDB.JobQueue('emailQueue');
emailQueue.addJob({ email: 'test@example.com', message: 'Hello!' });
emailQueue.process(5, async (job) => {
console.log(`Sending email to ${job.data.email}`);
});
Migration Management
Apply or rollback migrations:
await ScorpDB.Migrations.runMigrations(); // Apply all migrations
await ScorpDB.Migrations.rollbackMigration('001_create_users_table.js'); // Rollback a specific migration
Contribution
We welcome contributions to improve ScorpDB. Please feel free to open issues or submit pull requests.
License
ScorpDB is open-source software licensed under the MIT License.
FAQs
A powerful and modular Node.js database library for managing dynamic models, transactions, caching, RBAC, GraphQL, and more.
The npm package scorpdb receives a total of 0 weekly downloads. As such, scorpdb popularity was classified as not popular.
We found that scorpdb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 0 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Vite releases Rolldown-Vite, a Rust-based bundler preview offering faster builds and lower memory usage as a drop-in replacement for Vite.
Research
Security News
A malicious npm typosquat uses remote commands to silently delete entire project directories after a single mistyped install.
Research
Security News
Malicious PyPI package semantic-types steals Solana private keys via transitive dependency installs using monkey patching and blockchain exfiltration.