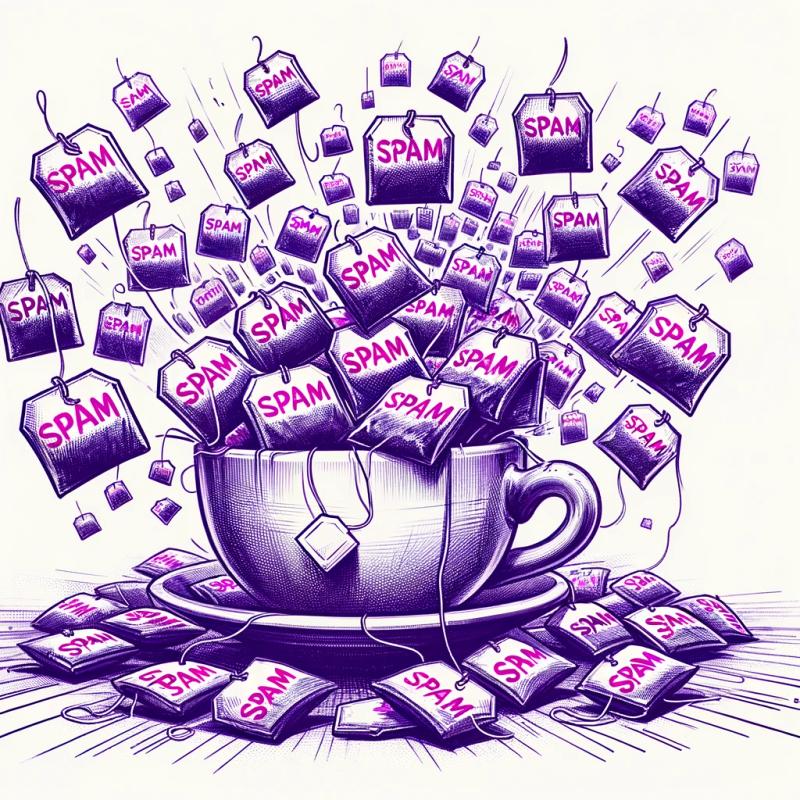
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
shortcuts.js
Advanced tools
Readme
A simple library for Apple's Shortcuts.
You can install this module with NPM:
npm install shortcuts.js
If you prefer Yarn, you can use that as well:
yarn add shortcuts.js
First, you must require this library:
const shortcuts = require("shortcuts.js");
Afterwards, you can use its methods. One of the simplest things you can do with this library is grab the ID from an iCloud URL. This is useful for later methods such as getting a shortcut's details from a user-submitted value, which might not always be just the ID.
// Get an ID from a iCloud URL
const id = shortcuts.idFromURL("https://www.icloud.com/shortcuts/903110dea9a944f48fef9e94317fb686");
This example uses promise chaining to get shortcut metadata from an iCloud URL:
// Chain promises to get a shortcut's metadata
shortcuts.getShortcutDetails(id).then(shortcut => {
console.log(`Hi, I'm ${id}! What's your name?`);
return shortcut.getMetadata();
}).then(metadata => {
console.log(`I have ${metadata.actions.length} actions! How cool is that?`);
}).catch(error => {
console.log(`${error.code}? How could this happen!`);
});
You can also use async/await to simplify things:
async function getBasicInfo() {
const shortcut = await shortcuts.getShortcutDetails(id);
const metadata = await shortcut.getMetadata();
return `Hi! I'm ${shortcut.name}. I have ${metadata.importQuestions.length} import questions, and I'm happy to be here. What's your name?`;
}
getBasicInfo().then(console.log).catch(error => {
console.log(`There was an error: ${error.code}. At least I can say that I tried...`);
});
This is the documentation for Shortcuts.js.
getShortcutDetails(id)
This method gets the details of a shortcut.
The id
argument is required and specifies the public ID of the shortcut to fetch. It can be found in the shareable URLs, in the form of https://icloud.com/shortcuts/<id>
.
This method immediately returns a promise, which will resolve to a Shortcut
.
idFromURL(url, allowSingle)
Gets a shortcut ID from its URL.
This method returns either false
or a string. If it returns a string, that string will be the parsed ID from the URL, or false
if the URL was unable to be parsed.
The url
is a string that can take on multiple forms:
903110dea9a944f48fef9e94317fb686
)http://icloud.com/shortcuts/903110dea9a944f48fef9e94317fb686
)https
protocol (https://icloud.com/shortcuts/903110dea9a944f48fef9e94317fb686
)www
subdomain (http://www.icloud.com/shortcuts/903110dea9a944f48fef9e94317fb686
)Shortcut
properties and methodsThis is what the getShortcutDetails()
promise will resolve with.
name
⟶ String
The user-specified name of the shortcut.
longDescription
⟶ String
The long description of the shortcut. This does not seem to be settable by users.
creationDate
⟶ Date
The date that the shortcut was created.
modificationDate
⟶ Date
The date that the shortcut was last modified on.
downloadURL
⟶ String
The URL to download the shortcut as a PLIST
icon
⟶ ShortcutIcon
Details of the icon of this shortcut.
response
⟶ Object
The full API response.
id
⟶ String
The ID of the shortcut.
getLink()
⟶ String
Gets the shortcut's landing page URL.
getMetadata()
⟶ Promise<ShortcutMetadata>
Downloads and parses the shortcut's metadata.
ShortcutIcon
propertiesA shortcut's icon.
color
⟶ Number
The color of the shortcut's icon, with transparency.
downloadURL
⟶ String
The URL to download the shortcut's icon.
glyph
⟶ Number
The ID of the glyph.
hexColor
⟶ String
The hexadecimal color of the shortcut's icon.
ShortcutMetadata
propertiesThe shortcut file with all the properties parsed into a custom class. The object will be found after calling getMetadata()
on a shortcut.
client
⟶ Object
Details of the client used to create this shortcut.
icon
⟶ Object
Details of the icon of this shortcut.
importQuestions
⟶ ImportQuestion[]
The user-specified name of the shortcut.
types
⟶ String[]
An unknown property.
actions
⟶ Action[]
A list of actions that the shortcut performs.
inputContentItemClasses
⟶ String[]
A list of services that the shortcut uses.
Action
propertiesA single action in a shortcut. The object will be found in the ShortcutMetadata.actions
property.
identifier
⟶ String
A namespace of the action.
parameters
⟶ Object
An object denoting a shortcut action. The properties in this object are not renamed and follow the Apple naming convention.
ImportQuestion
propertiesA single question to be asked when importing a shortcut into the Shortcuts app. The object will be found in the ShortcutMetadata.importQuestions
property.
parameterKey
⟶ String
An unknown property.
category
⟶ String
The category of the question.
actionIndex
⟶ Number
The action index of the question.
text
⟶ String
The question to be asked.
defaultValue
⟶ String
The default value for the question to be asked.
FAQs
A simple library for Apple's Shortcuts.
The npm package shortcuts.js receives a total of 0 weekly downloads. As such, shortcuts.js popularity was classified as not popular.
We found that shortcuts.js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.