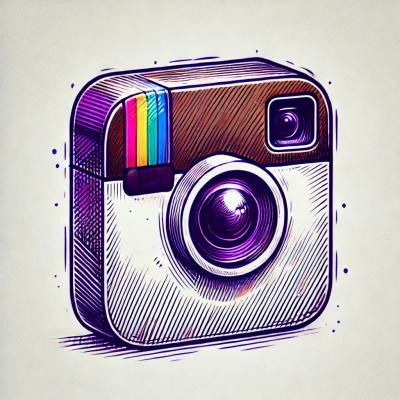
Research
PyPI Package Disguised as Instagram Growth Tool Harvests User Credentials
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
sib-api-v3-sdk
Advanced tools
SendinBlue_provide_a_RESTFul_API_that_can_be_used_with_any_languages__With_this_API_you_will_be_able_to_____Manage_your_campaigns_and_get_the_statistics____Manage_your_contacts____Send_transactional_Emails_and_SMS____and_much_more___You_can_download_our_w
SendinBlue's API exposes the entire SendinBlue features via a standardized programmatic interface. Please refer to the full documentation to learn more.
This is the wrapper for the API. It implements all the features of the API v3. It supports promises.
SendinBlue's API matches the OpenAPI v2 definition. The specification can be downloaded here.
This library is automatically generated by the Swagger Codegen project and is reviewed and maintained by SendinBlue:
To publish the library as a npm, please follow the procedure in "Publishing npm packages".
Then install it via:
npm install sib-api-v3-sdk --save
To use the library locally without publishing to a remote npm registry, first install the dependencies by changing
into the directory containing package.json
(and this README). Let's call this JAVASCRIPT_CLIENT_DIR
. Then run:
npm install
Next, link it globally in npm with the following, also from JAVASCRIPT_CLIENT_DIR
:
npm link
Finally, switch to the directory you want to use your sib-api-v3-sdk from, and run:
npm link /path/to/<JAVASCRIPT_CLIENT_DIR>
You should now be able to require('sib-api-v3-sdk')
in javascript files from the directory you ran the last
command above from.
If the library is hosted at a git repository, e.g. https://github.com/GIT_USER_ID/GIT_REPO_ID then install it via:
npm install GIT_USER_ID/GIT_REPO_ID --save
The library also works in the browser environment via npm and browserify. After following
the above steps with Node.js and installing browserify with npm install -g browserify
,
perform the following (assuming main.js is your entry file, that's to say your javascript file where you actually
use this library):
browserify main.js > bundle.js
Then include bundle.js in the HTML pages.
Using Webpack you may encounter the following error: "Module not found: Error: Cannot resolve module", most certainly you should disable AMD loader. Add/merge the following section to your webpack config:
module: {
rules: [
{
parser: {
amd: false
}
}
]
}
Please follow the installation instruction and execute the following JS code:
var SibApiV3Sdk = require('sib-api-v3-sdk');
var defaultClient = SibApiV3Sdk.ApiClient.instance;
// Configure API key authorization: api-key
var apiKey = defaultClient.authentications['api-key'];
apiKey.apiKey = "YOUR API KEY"
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//apiKey.apiKeyPrefix['api-key'] = "Token"
// Configure API key authorization: partner-key
var partnerKey = defaultClient.authentications['partner-key'];
partnerKey.apiKey = "YOUR API KEY"
// Uncomment the following line to set a prefix for the API key, e.g. "Token" (defaults to null)
//partnerKey.apiKeyPrefix['partner-key'] = "Token"
var api = new SibApiV3Sdk.AccountApi()
api.getAccount().then(function(data) {
console.log('API called successfully. Returned data: ' + data);
}, function(error) {
console.error(error);
});
All URIs are relative to https://api.sendinblue.com/v3
Class | Method | HTTP request | Description |
---|---|---|---|
SibApiV3Sdk.AccountApi | getAccount | GET /account | Get your account information, plan and credits details |
SibApiV3Sdk.CompaniesApi | companiesAttributesGet | GET /companies/attributes | Get company attributes |
SibApiV3Sdk.CompaniesApi | companiesGet | GET /companies | Get all companies |
SibApiV3Sdk.CompaniesApi | companiesIdDelete | DELETE /companies/{id} | Delete a company |
SibApiV3Sdk.CompaniesApi | companiesIdGet | GET /companies/{id} | Get a company |
SibApiV3Sdk.CompaniesApi | companiesIdPatch | PATCH /companies/{id} | Update a company |
SibApiV3Sdk.CompaniesApi | companiesLinkUnlinkIdPatch | PATCH /companies/link-unlink/{id} | Link and Unlink company with contacts and deals |
SibApiV3Sdk.CompaniesApi | companiesPost | POST /companies | Create a company |
SibApiV3Sdk.ContactsApi | addContactToList | POST /contacts/lists/{listId}/contacts/add | Add existing contacts to a list |
SibApiV3Sdk.ContactsApi | createAttribute | POST /contacts/attributes/{attributeCategory}/{attributeName} | Create contact attribute |
SibApiV3Sdk.ContactsApi | createContact | POST /contacts | Create a contact |
SibApiV3Sdk.ContactsApi | createDoiContact | POST /contacts/doubleOptinConfirmation | Create Contact via DOI (Double-Opt-In) Flow |
SibApiV3Sdk.ContactsApi | createFolder | POST /contacts/folders | Create a folder |
SibApiV3Sdk.ContactsApi | createList | POST /contacts/lists | Create a list |
SibApiV3Sdk.ContactsApi | deleteAttribute | DELETE /contacts/attributes/{attributeCategory}/{attributeName} | Delete an attribute |
SibApiV3Sdk.ContactsApi | deleteContact | DELETE /contacts/{identifier} | Delete a contact |
SibApiV3Sdk.ContactsApi | deleteFolder | DELETE /contacts/folders/{folderId} | Delete a folder (and all its lists) |
SibApiV3Sdk.ContactsApi | deleteList | DELETE /contacts/lists/{listId} | Delete a list |
SibApiV3Sdk.ContactsApi | getAttributes | GET /contacts/attributes | List all attributes |
SibApiV3Sdk.ContactsApi | getContactInfo | GET /contacts/{identifier} | Get a contact's details |
SibApiV3Sdk.ContactsApi | getContactStats | GET /contacts/{identifier}/campaignStats | Get email campaigns' statistics for a contact |
SibApiV3Sdk.ContactsApi | getContacts | GET /contacts | Get all the contacts |
SibApiV3Sdk.ContactsApi | getContactsFromList | GET /contacts/lists/{listId}/contacts | Get contacts in a list |
SibApiV3Sdk.ContactsApi | getFolder | GET /contacts/folders/{folderId} | Returns a folder's details |
SibApiV3Sdk.ContactsApi | getFolderLists | GET /contacts/folders/{folderId}/lists | Get lists in a folder |
SibApiV3Sdk.ContactsApi | getFolders | GET /contacts/folders | Get all folders |
SibApiV3Sdk.ContactsApi | getList | GET /contacts/lists/{listId} | Get a list's details |
SibApiV3Sdk.ContactsApi | getLists | GET /contacts/lists | Get all the lists |
SibApiV3Sdk.ContactsApi | importContacts | POST /contacts/import | Import contacts |
SibApiV3Sdk.ContactsApi | removeContactFromList | POST /contacts/lists/{listId}/contacts/remove | Delete a contact from a list |
SibApiV3Sdk.ContactsApi | requestContactExport | POST /contacts/export | Export contacts |
SibApiV3Sdk.ContactsApi | updateAttribute | PUT /contacts/attributes/{attributeCategory}/{attributeName} | Update contact attribute |
SibApiV3Sdk.ContactsApi | updateBatchContacts | POST /contacts/batch | Update multiple contacts |
SibApiV3Sdk.ContactsApi | updateContact | PUT /contacts/{identifier} | Update a contact |
SibApiV3Sdk.ContactsApi | updateFolder | PUT /contacts/folders/{folderId} | Update a folder |
SibApiV3Sdk.ContactsApi | updateList | PUT /contacts/lists/{listId} | Update a list |
SibApiV3Sdk.ConversationsApi | conversationsAgentOnlinePingPost | POST /conversations/agentOnlinePing | Sets agent’s status to online for 2-3 minutes |
SibApiV3Sdk.ConversationsApi | conversationsMessagesIdDelete | DELETE /conversations/messages/{id} | Delete a message sent by an agent |
SibApiV3Sdk.ConversationsApi | conversationsMessagesIdGet | GET /conversations/messages/{id} | Get a message |
SibApiV3Sdk.ConversationsApi | conversationsMessagesIdPut | PUT /conversations/messages/{id} | Update a message sent by an agent |
SibApiV3Sdk.ConversationsApi | conversationsMessagesPost | POST /conversations/messages | Send a message as an agent |
SibApiV3Sdk.ConversationsApi | conversationsPushedMessagesIdDelete | DELETE /conversations/pushedMessages/{id} | Delete an automated message |
SibApiV3Sdk.ConversationsApi | conversationsPushedMessagesIdGet | GET /conversations/pushedMessages/{id} | Get an automated message |
SibApiV3Sdk.ConversationsApi | conversationsPushedMessagesIdPut | PUT /conversations/pushedMessages/{id} | Update an automated message |
SibApiV3Sdk.ConversationsApi | conversationsPushedMessagesPost | POST /conversations/pushedMessages | Send an automated message to a visitor |
SibApiV3Sdk.DealsApi | crmAttributesDealsGet | GET /crm/attributes/deals | Get deal attributes |
SibApiV3Sdk.DealsApi | crmDealsGet | GET /crm/deals | Get all deals |
SibApiV3Sdk.DealsApi | crmDealsIdDelete | DELETE /crm/deals/{id} | Delete a deal |
SibApiV3Sdk.DealsApi | crmDealsIdGet | GET /crm/deals/{id} | Get a deal |
SibApiV3Sdk.DealsApi | crmDealsIdPatch | PATCH /crm/deals/{id} | Update a deal |
SibApiV3Sdk.DealsApi | crmDealsLinkUnlinkIdPatch | PATCH /crm/deals/link-unlink/{id} | Link and Unlink a deal with contacts and companies |
SibApiV3Sdk.DealsApi | crmDealsPost | POST /crm/deals | Create a deal |
SibApiV3Sdk.DealsApi | crmPipelineDetailsGet | GET /crm/pipeline/details | Get pipeline stages |
SibApiV3Sdk.EmailCampaignsApi | createEmailCampaign | POST /emailCampaigns | Create an email campaign |
SibApiV3Sdk.EmailCampaignsApi | deleteEmailCampaign | DELETE /emailCampaigns/{campaignId} | Delete an email campaign |
SibApiV3Sdk.EmailCampaignsApi | emailExportRecipients | POST /emailCampaigns/{campaignId}/exportRecipients | Export the recipients of an email campaign |
SibApiV3Sdk.EmailCampaignsApi | getAbTestCampaignResult | GET /emailCampaigns/{campaignId}/abTestCampaignResult | Get an A/B test email campaign results |
SibApiV3Sdk.EmailCampaignsApi | getEmailCampaign | GET /emailCampaigns/{campaignId} | Get an email campaign report |
SibApiV3Sdk.EmailCampaignsApi | getEmailCampaigns | GET /emailCampaigns | Return all your created email campaigns |
SibApiV3Sdk.EmailCampaignsApi | getSharedTemplateUrl | GET /emailCampaigns/{campaignId}/sharedUrl | Get a shared template url |
SibApiV3Sdk.EmailCampaignsApi | sendEmailCampaignNow | POST /emailCampaigns/{campaignId}/sendNow | Send an email campaign immediately, based on campaignId |
SibApiV3Sdk.EmailCampaignsApi | sendReport | POST /emailCampaigns/{campaignId}/sendReport | Send the report of a campaign |
SibApiV3Sdk.EmailCampaignsApi | sendTestEmail | POST /emailCampaigns/{campaignId}/sendTest | Send an email campaign to your test list |
SibApiV3Sdk.EmailCampaignsApi | updateCampaignStatus | PUT /emailCampaigns/{campaignId}/status | Update an email campaign status |
SibApiV3Sdk.EmailCampaignsApi | updateEmailCampaign | PUT /emailCampaigns/{campaignId} | Update an email campaign |
SibApiV3Sdk.EmailCampaignsApi | uploadImageToGallery | POST /emailCampaigns/images | Upload an image to your account's image gallery |
SibApiV3Sdk.FilesApi | crmFilesGet | GET /crm/files | Get all files |
SibApiV3Sdk.FilesApi | crmFilesIdDataGet | GET /crm/files/{id}/data | Get file details |
SibApiV3Sdk.FilesApi | crmFilesIdDelete | DELETE /crm/files/{id} | Delete a file |
SibApiV3Sdk.FilesApi | crmFilesIdGet | GET /crm/files/{id} | Download a file |
SibApiV3Sdk.FilesApi | crmFilesPost | POST /crm/files | Upload a file |
SibApiV3Sdk.InboundParsingApi | getInboundEmailAttachment | GET /inbound/attachments/{downloadToken} | Retrieve inbound attachment with download token. |
SibApiV3Sdk.InboundParsingApi | getInboundEmailEvents | GET /inbound/events | Get the list of all the events for the received emails. |
SibApiV3Sdk.InboundParsingApi | getInboundEmailEventsByUuid | GET /inbound/events/{uuid} | Fetch all events history for one particular received email. |
SibApiV3Sdk.MasterAccountApi | corporateMasterAccountGet | GET /corporate/masterAccount | Get the details of requested master account |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountGet | GET /corporate/subAccount | Get the list of all the sub-accounts of the master account. |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountIdDelete | DELETE /corporate/subAccount/{id} | Delete a sub-account |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountIdGet | GET /corporate/subAccount/{id} | Get sub-account details |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountIdPlanPut | PUT /corporate/subAccount/{id}/plan | Update sub-account plan |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountPost | POST /corporate/subAccount | Create a new sub-account under a master account. |
SibApiV3Sdk.MasterAccountApi | corporateSubAccountSsoTokenPost | POST /corporate/subAccount/ssoToken | Generate SSO token to access Sendinblue |
SibApiV3Sdk.NotesApi | crmNotesGet | GET /crm/notes | Get all notes |
SibApiV3Sdk.NotesApi | crmNotesIdDelete | DELETE /crm/notes/{id} | Delete a note |
SibApiV3Sdk.NotesApi | crmNotesIdGet | GET /crm/notes/{id} | Get a note |
SibApiV3Sdk.NotesApi | crmNotesIdPatch | PATCH /crm/notes/{id} | Update a note |
SibApiV3Sdk.NotesApi | crmNotesPost | POST /crm/notes | Create a note |
SibApiV3Sdk.ProcessApi | getProcess | GET /processes/{processId} | Return the informations for a process |
SibApiV3Sdk.ProcessApi | getProcesses | GET /processes | Return all the processes for your account |
SibApiV3Sdk.ResellerApi | addCredits | POST /reseller/children/{childIdentifier}/credits/add | Add Email and/or SMS credits to a specific child account |
SibApiV3Sdk.ResellerApi | associateIpToChild | POST /reseller/children/{childIdentifier}/ips/associate | Associate a dedicated IP to the child |
SibApiV3Sdk.ResellerApi | createChildDomain | POST /reseller/children/{childIdentifier}/domains | Create a domain for a child account |
SibApiV3Sdk.ResellerApi | createResellerChild | POST /reseller/children | Creates a reseller child |
SibApiV3Sdk.ResellerApi | deleteChildDomain | DELETE /reseller/children/{childIdentifier}/domains/{domainName} | Delete the sender domain of the reseller child based on the childIdentifier and domainName passed |
SibApiV3Sdk.ResellerApi | deleteResellerChild | DELETE /reseller/children/{childIdentifier} | Delete a single reseller child based on the child identifier supplied |
SibApiV3Sdk.ResellerApi | dissociateIpFromChild | POST /reseller/children/{childIdentifier}/ips/dissociate | Dissociate a dedicated IP to the child |
SibApiV3Sdk.ResellerApi | getChildAccountCreationStatus | GET /reseller/children/{childIdentifier}/accountCreationStatus | Get the status of a reseller's child account creation, whether it is successfully created (exists) or not based on the identifier supplied |
SibApiV3Sdk.ResellerApi | getChildDomains | GET /reseller/children/{childIdentifier}/domains | Get all sender domains for a specific child account |
SibApiV3Sdk.ResellerApi | getChildInfo | GET /reseller/children/{childIdentifier} | Get a child account's details |
SibApiV3Sdk.ResellerApi | getResellerChilds | GET /reseller/children | Get the list of all children accounts |
SibApiV3Sdk.ResellerApi | getSsoToken | GET /reseller/children/{childIdentifier}/auth | Get session token to access Sendinblue (SSO) |
SibApiV3Sdk.ResellerApi | removeCredits | POST /reseller/children/{childIdentifier}/credits/remove | Remove Email and/or SMS credits from a specific child account |
SibApiV3Sdk.ResellerApi | updateChildAccountStatus | PUT /reseller/children/{childIdentifier}/accountStatus | Update info of reseller's child account status based on the childIdentifier supplied |
SibApiV3Sdk.ResellerApi | updateChildDomain | PUT /reseller/children/{childIdentifier}/domains/{domainName} | Update the sender domain of reseller's child based on the childIdentifier and domainName passed |
SibApiV3Sdk.ResellerApi | updateResellerChild | PUT /reseller/children/{childIdentifier} | Update info of reseller's child based on the child identifier supplied |
SibApiV3Sdk.SMSCampaignsApi | createSmsCampaign | POST /smsCampaigns | Creates an SMS campaign |
SibApiV3Sdk.SMSCampaignsApi | deleteSmsCampaign | DELETE /smsCampaigns/{campaignId} | Delete an SMS campaign |
SibApiV3Sdk.SMSCampaignsApi | getSmsCampaign | GET /smsCampaigns/{campaignId} | Get an SMS campaign |
SibApiV3Sdk.SMSCampaignsApi | getSmsCampaigns | GET /smsCampaigns | Returns the information for all your created SMS campaigns |
SibApiV3Sdk.SMSCampaignsApi | requestSmsRecipientExport | POST /smsCampaigns/{campaignId}/exportRecipients | Export an SMS campaign's recipients |
SibApiV3Sdk.SMSCampaignsApi | sendSmsCampaignNow | POST /smsCampaigns/{campaignId}/sendNow | Send your SMS campaign immediately |
SibApiV3Sdk.SMSCampaignsApi | sendSmsReport | POST /smsCampaigns/{campaignId}/sendReport | Send an SMS campaign's report |
SibApiV3Sdk.SMSCampaignsApi | sendTestSms | POST /smsCampaigns/{campaignId}/sendTest | Send a test SMS campaign |
SibApiV3Sdk.SMSCampaignsApi | updateSmsCampaign | PUT /smsCampaigns/{campaignId} | Update an SMS campaign |
SibApiV3Sdk.SMSCampaignsApi | updateSmsCampaignStatus | PUT /smsCampaigns/{campaignId}/status | Update a campaign's status |
SibApiV3Sdk.SendersApi | createSender | POST /senders | Create a new sender |
SibApiV3Sdk.SendersApi | deleteSender | DELETE /senders/{senderId} | Delete a sender |
SibApiV3Sdk.SendersApi | getIps | GET /senders/ips | Get all the dedicated IPs for your account |
SibApiV3Sdk.SendersApi | getIpsFromSender | GET /senders/{senderId}/ips | Get all the dedicated IPs for a sender |
SibApiV3Sdk.SendersApi | getSenders | GET /senders | Get the list of all your senders |
SibApiV3Sdk.SendersApi | updateSender | PUT /senders/{senderId} | Update a sender |
SibApiV3Sdk.TasksApi | crmTasksGet | GET /crm/tasks | Get all tasks |
SibApiV3Sdk.TasksApi | crmTasksIdDelete | DELETE /crm/tasks/{id} | Delete a task |
SibApiV3Sdk.TasksApi | crmTasksIdGet | GET /crm/tasks/{id} | Get a task |
SibApiV3Sdk.TasksApi | crmTasksIdPatch | PATCH /crm/tasks/{id} | Update a task |
SibApiV3Sdk.TasksApi | crmTasksPost | POST /crm/tasks | Create a task |
SibApiV3Sdk.TasksApi | crmTasktypesGet | GET /crm/tasktypes | Get all task types |
SibApiV3Sdk.TransactionalEmailsApi | blockNewDomain | POST /smtp/blockedDomains | Add a new domain to the list of blocked domains |
SibApiV3Sdk.TransactionalEmailsApi | createSmtpTemplate | POST /smtp/templates | Create an email template |
SibApiV3Sdk.TransactionalEmailsApi | deleteBlockedDomain | DELETE /smtp/blockedDomains/{domain} | Unblock an existing domain from the list of blocked domains |
SibApiV3Sdk.TransactionalEmailsApi | deleteHardbounces | POST /smtp/deleteHardbounces | Delete hardbounces |
SibApiV3Sdk.TransactionalEmailsApi | deleteScheduledEmailById | DELETE /smtp/email/{identifier} | Delete scheduled emails by batchId or messageId |
SibApiV3Sdk.TransactionalEmailsApi | deleteSmtpTemplate | DELETE /smtp/templates/{templateId} | Delete an inactive email template |
SibApiV3Sdk.TransactionalEmailsApi | getAggregatedSmtpReport | GET /smtp/statistics/aggregatedReport | Get your transactional email activity aggregated over a period of time |
SibApiV3Sdk.TransactionalEmailsApi | getBlockedDomains | GET /smtp/blockedDomains | Get the list of blocked domains |
SibApiV3Sdk.TransactionalEmailsApi | getEmailEventReport | GET /smtp/statistics/events | Get all your transactional email activity (unaggregated events) |
SibApiV3Sdk.TransactionalEmailsApi | getScheduledEmailByBatchId | GET /smtp/emailStatus/{batchId} | Fetch scheduled emails by batchId |
SibApiV3Sdk.TransactionalEmailsApi | getScheduledEmailByMessageId | GET /smtp/emailStatus/{messageId} | Fetch scheduled email by messageId |
SibApiV3Sdk.TransactionalEmailsApi | getSmtpReport | GET /smtp/statistics/reports | Get your transactional email activity aggregated per day |
SibApiV3Sdk.TransactionalEmailsApi | getSmtpTemplate | GET /smtp/templates/{templateId} | Returns the template information |
SibApiV3Sdk.TransactionalEmailsApi | getSmtpTemplates | GET /smtp/templates | Get the list of email templates |
SibApiV3Sdk.TransactionalEmailsApi | getTransacBlockedContacts | GET /smtp/blockedContacts | Get the list of blocked or unsubscribed transactional contacts |
SibApiV3Sdk.TransactionalEmailsApi | getTransacEmailContent | GET /smtp/emails/{uuid} | Get the personalized content of a sent transactional email |
SibApiV3Sdk.TransactionalEmailsApi | getTransacEmailsList | GET /smtp/emails | Get the list of transactional emails on the basis of allowed filters |
SibApiV3Sdk.TransactionalEmailsApi | sendTestTemplate | POST /smtp/templates/{templateId}/sendTest | Send a template to your test list |
SibApiV3Sdk.TransactionalEmailsApi | sendTransacEmail | POST /smtp/email | Send a transactional email |
SibApiV3Sdk.TransactionalEmailsApi | smtpBlockedContactsEmailDelete | DELETE /smtp/blockedContacts/{email} | Unblock or resubscribe a transactional contact |
SibApiV3Sdk.TransactionalEmailsApi | smtpLogMessageIdDelete | DELETE /smtp/log/{messageId} | Delete an SMTP transactional log |
SibApiV3Sdk.TransactionalEmailsApi | updateSmtpTemplate | PUT /smtp/templates/{templateId} | Update an email template |
SibApiV3Sdk.TransactionalSMSApi | getSmsEvents | GET /transactionalSMS/statistics/events | Get all your SMS activity (unaggregated events) |
SibApiV3Sdk.TransactionalSMSApi | getTransacAggregatedSmsReport | GET /transactionalSMS/statistics/aggregatedReport | Get your SMS activity aggregated over a period of time |
SibApiV3Sdk.TransactionalSMSApi | getTransacSmsReport | GET /transactionalSMS/statistics/reports | Get your SMS activity aggregated per day |
SibApiV3Sdk.TransactionalSMSApi | sendTransacSms | POST /transactionalSMS/sms | Send SMS message to a mobile number |
SibApiV3Sdk.WebhooksApi | createWebhook | POST /webhooks | Create a webhook |
SibApiV3Sdk.WebhooksApi | deleteWebhook | DELETE /webhooks/{webhookId} | Delete a webhook |
SibApiV3Sdk.WebhooksApi | getWebhook | GET /webhooks/{webhookId} | Get a webhook details |
SibApiV3Sdk.WebhooksApi | getWebhooks | GET /webhooks | Get all webhooks |
SibApiV3Sdk.WebhooksApi | updateWebhook | PUT /webhooks/{webhookId} | Update a webhook |
FAQs
SendinBlue_provide_a_RESTFul_API_that_can_be_used_with_any_languages__With_this_API_you_will_be_able_to_____Manage_your_campaigns_and_get_the_statistics____Manage_your_contacts____Send_transactional_Emails_and_SMS____and_much_more___You_can_download_our_w
The npm package sib-api-v3-sdk receives a total of 38,400 weekly downloads. As such, sib-api-v3-sdk popularity was classified as popular.
We found that sib-api-v3-sdk demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
A deceptive PyPI package posing as an Instagram growth tool collects user credentials and sends them to third-party bot services.
Product
Socket now supports pylock.toml, enabling secure, reproducible Python builds with advanced scanning and full alignment with PEP 751's new standard.
Security News
Research
Socket uncovered two npm packages that register hidden HTTP endpoints to delete all files on command.