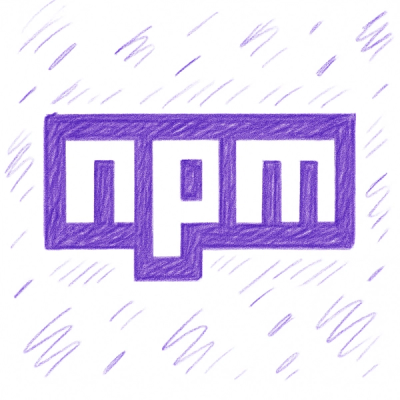
Security News
npm Adopts OIDC for Trusted Publishing in CI/CD Workflows
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
simplest-react-store
Advanced tools
[npm-url]: https://npmjs.org/package/simplest-react-store [npm-image]: http://img.shields.io/npm/v/simplest-react-store.svg
Simplest react store that does the job.
This package was originally written for fun some years ago.
Lightweight and easy-to-use state management solution for React applications. It provides a simple API for creating and managing stores, making state management more approachable and less cumbersome. With this package, developers can quickly set up and utilize state management in their React projects without the need for complex setups or extensive boilerplate code.
The key features of Simplest-React-Store include:
Minimalist Approach: The package embraces simplicity and aims to provide a straightforward solution for state management in React applications.
Small Footprint: Simplest-React-Store is designed to be lightweight and has a minimal impact on bundle size, ensuring optimal performance for applications.
Intuitive API: The API is intuitive and easy to understand, making it accessible for developers of all skill levels.
Using npm:
npm install simplest-react-store
Using yarn:
yarn add simplest-react-store
// store.ts
import { createStore } from 'simplest-react-store';
// Define the initial state of the store. This includes the properties isHelloShown, helloText, and user with their initial values.
const initialState = {
isHelloShown: false,
helloText: "",
user: {
name: "Matt",
age: 40,
},
};
export type State = typeof initialState;
// Define the actions that can be performed on the store.
// These actions are functions that receive the current state and
// any necessary parameters, and they update the state accordingly.
const actions = {
showHello: (state: State, toggle: boolean) => (state.isHelloShown = toggle),
setHelloText: (state: State, value: string) => (state.helloText = value),
setUser(
state: State,
newUserData: Partial<State["user"]>
) {
// For objects, it works similar to a standard reducer and is particularly useful for handling nested objects
// Update the user state by merging newUserData with the current user object
return { user: { ...state.user, ...newUserData } };
},
};
// Create the store by calling the createStore function and passing the initial state and actions.
export const { useStore, useStoreProp, Provider } = createStore(
initialState,
actions
);
Wrap your React application with the Provider component provided by the store. This makes the store available to all components in the application.
// _app.tsx
import { Provider as MyStoreProvider } from "./store";
function App({ Component, pageProps }: AppProps) {
return (
<MyStoreProvider>
/* Your app code */
</MyStoreProvider>
);
}
Access the store's state and actions in your components using the useStore and useStoreProp hooks.
// MyComponent.tsx
import { useEffect } from 'react';
import { useStoreProp } from "store";
export default function MyComponent() {
const [helloText] = useStoreProp("helloText");
const [user] = useStoreProp("user");
const [showHello, dispatch] = useStoreProp("isHelloShown");
useEffect(() => {
// You can use same dispatch for all actions as it is the same store
dispatch.setUser({name: "David", age: 19});
dispatch.setHelloText("Hello");
dispatch.showHello(true);
}, [dispatch]);
return (
<div>
{showHello && (
<span>
{helloText} {user.name}
</span>
)}
</div>
);
}
That's it! Now you can use the state properties and actions from the store in your components to manage and update the state of your application. Remember to import the necessary functions and components from the store.ts file where needed.
If you have any idea for an improvement, please file an issue. Feel free to make a PR if you are willing to collaborate on the project. Thank you :)
FAQs
[npm-url]: https://npmjs.org/package/simplest-react-store [npm-image]: http://img.shields.io/npm/v/simplest-react-store.svg
The npm package simplest-react-store receives a total of 0 weekly downloads. As such, simplest-react-store popularity was classified as not popular.
We found that simplest-react-store demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
npm now supports Trusted Publishing with OIDC, enabling secure package publishing directly from CI/CD workflows without relying on long-lived tokens.
Research
/Security News
A RubyGems malware campaign used 60 malicious packages posing as automation tools to steal credentials from social media and marketing tool users.
Security News
The CNA Scorecard ranks CVE issuers by data completeness, revealing major gaps in patch info and software identifiers across thousands of vulnerabilities.