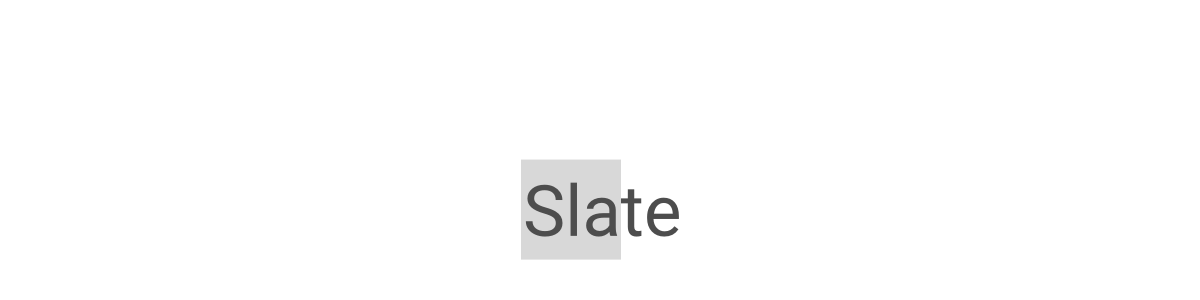
slate-react library implemented with vue
Why use it?
- Use vue internal response implementation to reduce the number of re-renderings
- You can easily use vue-devtools to debug in a vue project
- This library provides the same usage as slate-react
Demo
Check out the live demo of all of the examples
Hooks
const useFocused: () => Ref<boolean, boolean>
Get the current composing state of the editor. It deals with compositionstart, compositionupdate, compositionend events.
Composition events are triggered by typing (composing) with a language that uses a composition character (e.g. Chinese, Japanese, Korean, etc.) example.
Some basic Git commands are:
const useFocused: () => Ref<boolean, boolean>
Get the current focused state of the editor.
const useReadOnly: () => Ref<boolean, boolean>
Get the current readOnly state of the editor.
const useSelected: () => ComputedRef<boolean>
Get the current selected state of an element.
const useSlate: () => Editor;
Get the current editor object from the React context. Re-renders the context whenever changes occur in the editor.
const useSelection: () => ComputedRef<boolean>
Get the current editor selection from the React context.
Packages
slate's codebase is monorepo managed with pnpm workspace
- slate
slate core logic, update synchronously with slate
- slate-dom
Implementation of slate on dom, update synchronously with slate-dom
- slate-vue
Vue components for rendering slate editors
- share-tools
for special processing of proxy type data, obtain the raw pointer, isPlainObject declare
compact files of slate
reactive implement
- packages/slate/src/interfaces/text.ts 115:115
- packages/slate/src/create-editor.ts 94:94
- packages/slate/src/transforms-node/set-nodes.ts 18:18
remove immer
- packages/slate/src/interfaces/node.ts 365:365
- packages/slate/src/interfaces/point.ts 103:103
- packages/slate/src/interfaces/range.ts 224:224
- packages/slate/src/interfaces/transforms/general.ts 322:333
rewrite implement for WeakMap
- packages/un-proxy-weakmap/src/index.ts
- packages/slate-dom/src/utils/weak-maps.ts
import types from globalThis in slate-dom
- packages/slate-dom/src/index.ts
- packages/slate-dom/src/plugin/dom-editor.ts
- packages/slate-dom/src/utils/dom.ts