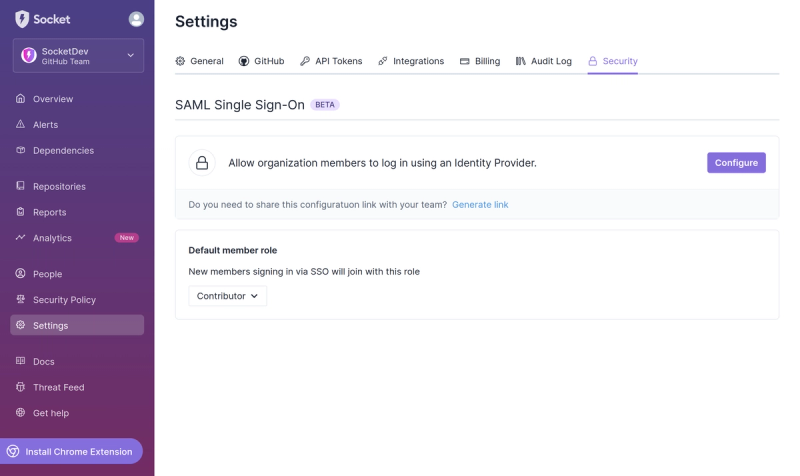
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
slonik-plus
Advanced tools
Readme
Extends slonik to add definable typescript models and additional features like upsert
.
It is not an ORM, but it adds the benefits of definable TypeScript models that can make querying / updates much easier.
This is a library I've written and have used extensively in large enterprise systems. I feel it would be useful to others, so I'm open sourcing it.
Unfortunately, I don't have time to write full, proper external documentation yet. However, its options are documented, and can be seen using intellisense or by examining the code in this repo.
Now that it's open source, there is certainly room for improvement and expanded functionality. You are welcome to submit PRs and requests in the issues!
Also, if anyone finds it useful and would like to help by expanding it or the readme, it would be greatly appreciated!
// Note: In addition to our extras, all slonik exports are included, so you can import them
// directly from the slonik-plus library
import { createPoolPlus } from "slonik-plus";
const pool = createPoolPlus(/* ... */);
Unlike an ORM, models do not get created on PG. They're simply a typescript definition which provide binding to database objects & queries.
// Note: Check intellisense for more options in the `@record` param such as appending
// to the query or overriding entirely, which allows you to create a model with a specific
// query attached
@record({ table: 'listing' })
export class ListingRecord extends DbRecord<
ListingRecord,
// Note: This optional config object allows customizing the constructor's input parameter
{
exclude: 'listingId' // Exclude the listingId, because it's auto-generated by PG
partial: 'allowsHourly' // Make optional, because the PG table has a default value specifed
}
> {
// Note: primaryKey can also be numeric for multi-prop keys
@column({ type: 'bigint', primaryKey: true, out: false })
readonly declare listingId: number;
@column({ type: 'listing.listing_site' })
readonly declare listingSite: T;
@column({ type: 'text' })
declare title: string;
@column({ type: 'timestamptz', out: false })
readonly declare postDt: Date;
// Note: A trigger sets this field, so we make it readonly and use out: false to
// prevent it from being updatable
@column({ type: 'timestamptz', out: false })
readonly declare updatedDt?: Date;
@column({ type: 'text' })
declare summary?: string;
@column({ type: 'bool' })
declare allowsHourly: boolean;
}
// Get listing records
const recs = await pool.maybeMany(
ListingRecord,
{ append: sql.fragment`WHERE allows_hourly = true` } // See intellisense for more options
);
// Can still use regular queries
const manualRecs = await pool.maybeMany(sql.unsafe`SELECT * from listing`);
// Upsert
const rec1 = new ProjectListing({ /* ... */ });
const rec2 = new ProjectListing({ /* ... */ });
await pool.upsert(
[ rec1, rec2 ], // Can also update a signle record
{ /* Note: See intellisense for optional extended options */ }
);
// Modify & Update
rec1.allowsHourly = false;
await rec1.update(pool);
FAQs
Extends slonik to add definable typescript models and additional features
The npm package slonik-plus receives a total of 1 weekly downloads. As such, slonik-plus popularity was classified as not popular.
We found that slonik-plus demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.