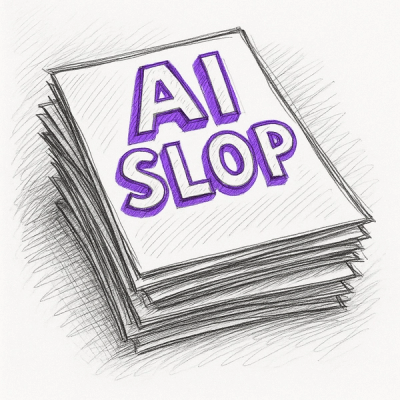
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Lightweight stream oriented messaging library.
SocketMQ supports req/rep
and pub/sub
messaging patterns. Messaging pattern is high level concept of how messages should be handled on sending and receiving. It's client/server & transport agnostic
which means you can use all 4 types of messaging pattern no matter you are connected to a server or being connected from a client with any of the transports supported.
Each request
will be sent to one
connected client/server and wait for response
(round-robin scheduler).
// example/rep.js
var socketmq = require('../')
var smq = socketmq.bind('tcp://127.0.0.1:6363')
smq.on('bind', function() {
console.log('rep bound')
})
var event = 'hello' // or you can call it topic or channel.
smq.on('connect', function(stream) {
console.log('new connection')
smq.req(event, 'request from server', function (msg) {
console.log(msg)
})
})
smq.rep(event, function(msg, reply) {
console.log('requested msg:' + msg)
reply('Hi ' + msg + ', world!')
})
// example/req.js
var socketmq = require('socketmq')
var smq = socketmq.connect('tcp://127.0.0.1:6363')
smq.on('connect', function(stream) {
console.log('req connected to server')
})
setInterval(function() {
smq.req('hello', 'socketmq.req', function(msg) {
console.log('replied msg:' + msg)
})
}, 1000)
smq.rep('hello', function (msg, reply) {
console.log('request from server', msg)
reply('response from client')
})
A pub
message will be distributed to all client/server connected and no response will be sent back. It's a fire and forget messaging pattern. Pub messages could be received by subscribing to the publishing topic.
// example/pub.js
var socketmq = require('socketmq')
var smq = socketmq.connect('tcp://0.0.0.0:6363')
smq.on('connect', function() {
console.log('pub connected');
setInterval(function() {
smq.pub('pub.test', 'hello')
}, 1000)
})
// example/sub.js
var socketmq = require('socketmq')
var smq = socketmq.bind('tcp://127.0.0.1:6363')
smq.on('bind', function() {
console.log('sub bound')
})
smq.sub('pub.test', function(data) {
console.log('got pub message: ' + data)
})
SocketMQ can tag the streams and send messages to streams with specific tags.
var socketmq = require('socketmq')
var tcpUri = 'tcp://127.0.0.1:6363'
var smq = socketmq.connect(tcpUri, function(stream) {
// Tag the stream.
smq.tag(stream, 'tcp')
})
var tlsUri = 'tls://127.0.0.1:46363'
smq.connect(tlsUri, function(stream) {
smq.tag(stream, 'tls')
})
// Then send messages using `reqTag` of `pubTag`
// `req` message only to server with `tcp` tag
smq.reqTag('tcp', 'hello', 'message', function(){
// ...
})
// `pub` message only to server with `tls` tag
smq.pubTag('tls', 'hello', 'message')
FAQs
Lightweight messaging library for node & browser.
The npm package socketmq receives a total of 12 weekly downloads. As such, socketmq popularity was classified as not popular.
We found that socketmq demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.