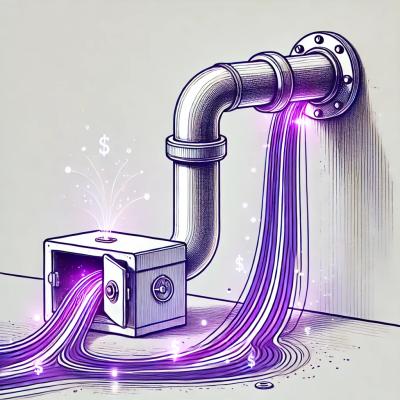
Research
Security News
Malicious npm Package Targets Solana Developers and Hijacks Funds
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
sort-algorithms-js
Advanced tools
production-ready sort algorithms implementation in javascript.
sort algorithms implementation with ability to use a compare callback similar to javascript .sort
.
npm install --save sort-algorithms-js
const {
bubbleSort, insertionSort,
selectionSort, radixSort,
mergeSort, heapSort, quickSort
} = require('sort-algorithms-js');
import {
bubbleSort, insertionSort,
selectionSort, radixSort,
mergeSort, heapSort, quickSort
} from 'sort-algorithms-js';
runtime complexity: O(n^2)
bubbleSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
bubbleSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
1k | 0 seconds 5 ms | 0 seconds 9 ms |
10k | 0 seconds 227 ms | 0 seconds 249 ms |
50k | 6 seconds 411 ms | 7 seconds 998 ms |
100k | 26 seconds 653 ms | 29 seconds 735 ms |
1M | ❌ |
runtime complexity: O(n^2)
insertionSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
insertionSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
1k | 0 seconds 5 ms | 0 seconds 10 ms |
10k | 0 seconds 129 ms | 0 seconds 145 ms |
50k | 3 seconds 49 ms | 3 seconds 596 ms |
100k | 13 seconds 575 ms | 16 seconds 876 ms |
1M | ❌ |
runtime complexity: O(n^2)
selectionSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
selectionSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
1k | 0 seconds 4 ms | 0 seconds 8 ms |
10k | 0 seconds 125 ms | 0 seconds 139 ms |
50k | 2 seconds 178 ms | 2 seconds 302 ms |
100k | 9 seconds 740 ms | 10 seconds 460 ms |
1M | ❌ |
Only sorts numbers in O(n*d) runtime : d is the number of digits in the largest number.
radixSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
radixSort([2, 1, 7, 3, 9, -1, -5], 'desc'); // [ 9, 7, 3, 2, 1, -1, -5 ]
radixSort([{ id: 341 }, { id: 947 }, { id: 132 }], 'asc', (obj) => obj.id); // [ { id: 132 }, { id: 341 }, { id: 947 } ]
radixSort([{ id: 341 }, { id: 947 }, { id: 132 }], 'desc', (obj) => obj.id); // [ { id: 947 }, { id: 341 }, { id: 132 } ]
Node v14 | ||
input size | best time | worst time |
10k | 0 seconds 21 ms | 0 seconds 30 ms |
50k | 0 seconds 61 ms | 0 seconds 81 ms |
100k | 0 seconds 97 ms | 0 seconds 115 ms |
1M | 1 seconds 27 ms | 1 seconds 103 ms |
10M | 13 seconds 844 ms | 17 seconds 257 ms |
50M | ❌ |
runtime complexity: O(n*log(n))
heapSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
heapSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
10k | 0 seconds 12 ms | 0 seconds 14 ms |
50k | 0 seconds 21 ms | 0 seconds 25 ms |
100k | 0 seconds 31 ms | 0 seconds 44 ms |
1M | 0 seconds 283 ms | 0 seconds 313 ms |
10M | 5 seconds 219 ms | 6 seconds 367 ms |
50M | 34 seconds 21 ms | 46 seconds 167 ms |
100M | 76 seconds 485 ms | 87 seconds 991 ms |
runtime complexity: O(n*log(n))
mergeSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
mergeSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
10k | 0 seconds 16 ms | 0 seconds 23 ms |
50k | 0 seconds 38 ms | 0 seconds 45 ms |
100k | 0 seconds 54 ms | 0 seconds 60 ms |
1M | 0 seconds 413 ms | 0 seconds 435 ms |
10M | 5 seconds 78 ms | 6 seconds 712 ms |
50M | 33 seconds 229 ms | 35 seconds 659 ms |
100M | 82 seconds 777 ms | 86 seconds 194 ms |
runtime complexity: O(n*log(n))
quickSort([2, 1, 7, 3, 9, -1, -5]); // [ -5, -1, 1, 2, 3, 7, 9 ]
quickSort([2, 1, 7, 3, 9, -1, -5], (a, b) => b - a); // [ 9, 7, 3, 2, 1, -1, -5 ]
Node v14 | ||
input size | best time | worst time |
10k | 0 seconds 6 ms | 0 seconds 13 ms |
50k | 0 seconds 18 ms | 0 seconds 26 ms |
100k | 0 seconds 26 ms | 0 seconds 34 ms |
1M | 0 seconds 167 ms | 0 seconds 187 ms |
10M | 1 seconds 831 ms | 2 seconds 188 ms |
50M | 10 seconds 402 ms | 14 seconds 777 ms |
100M | 24 seconds 253 ms | 34 seconds 705 ms |
grunt build
The MIT License. Full License is here
FAQs
production-ready sort algorithms implementation in javascript.
The npm package sort-algorithms-js receives a total of 93 weekly downloads. As such, sort-algorithms-js popularity was classified as not popular.
We found that sort-algorithms-js demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
A malicious npm package targets Solana developers, rerouting funds in 2% of transactions to a hardcoded address.
Security News
Research
Socket researchers have discovered malicious npm packages targeting crypto developers, stealing credentials and wallet data using spyware delivered through typosquats of popular cryptographic libraries.
Security News
Socket's package search now displays weekly downloads for npm packages, helping developers quickly assess popularity and make more informed decisions.