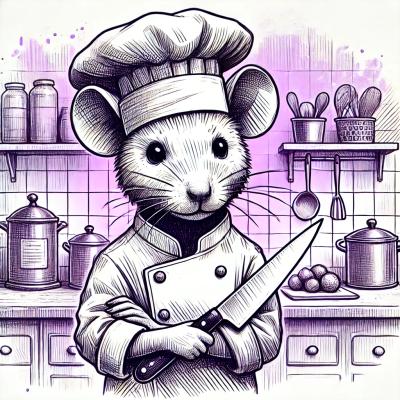
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
sort-object-keys
Advanced tools
The sort-object-keys npm package is designed to sort the keys of an object based on a specific order provided by the user or in natural order if no order is specified. This can be particularly useful for ensuring consistent object key ordering, for example, when formatting JSON files or ensuring consistent object structures in code.
Sort keys in natural order
This feature allows the user to sort the keys of an object in ascending natural order. It is useful for creating predictable object key orders.
const sortObjectKeys = require('sort-object-keys');
const obj = {b: 2, a: 1, c: 3};
const sortedObj = sortObjectKeys(obj);
console.log(sortedObj); // Output: {a: 1, b: 2, c: 3}
Sort keys according to a custom order
This feature allows the user to sort the keys of an object based on a custom order array provided. This is particularly useful when a specific key order is required for readability or functionality.
const sortObjectKeys = require('sort-object-keys');
const order = ['c', 'a', 'b'];
const obj = {b: 2, a: 1, c: 3};
const sortedObj = sortObjectKeys(obj, order);
console.log(sortedObj); // Output: {c: 3, a: 1, b: 2}
Lodash's sortBy function offers similar functionality for sorting collections based on one or several criteria. While it primarily focuses on array sorting, it can be adapted for object keys sorting. It differs from sort-object-keys as it is part of a larger utility library and not focused solely on object key sorting.
Returns a copy of an object with all keys sorted.
The second argument is optional and is used for ordering - to provide custom sorts. You can either pass an array containing ordered keys or a function to sort the keys (same signature as in Array.prototype.sort()
).
const assert = require('assert');
const sortObject = require('sort-object-keys');
assert.equal(JSON.stringify({
c: 1,
b: 1,
d: 1,
a: 1,
}), JSON.stringify({
a: 1,
b: 1,
c: 1,
d: 1,
}));
assert.equal(JSON.stringify(sortObject({
c: 1,
b: 1,
d: 1,
a: 1,
}, ['b', 'a', 'd', 'c'])), JSON.stringify({
b: 1,
a: 1,
d: 1,
c: 1,
}));
function removeKeyAncCompareIndex(keyA, keyB){
var a = parseInt(keyA.slice(4));
var b = parseInt(keyB.slice(4));
return a - b;
}
assert.equal(JSON.stringify(sortObject({
"key-1": 1,
"key-3": 1,
"key-10": 1,
"key-2": 1,
}, removeKeyAncCompareIndex)), JSON.stringify({
"key-1": 1,
"key-2": 1,
"key-3": 1,
"key-10": 1,
}));
FAQs
Sort an object's keys, including an optional key list
The npm package sort-object-keys receives a total of 1,534,200 weekly downloads. As such, sort-object-keys popularity was classified as popular.
We found that sort-object-keys demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.