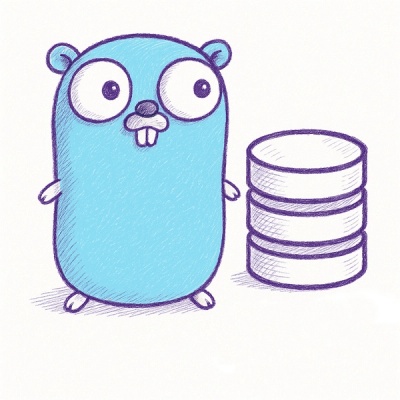
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
SQLite client for Node.js applications with SQL-based migrations API written in Typescript
The sqlite npm package is a wrapper for SQLite, a C library that provides a lightweight, disk-based database. It doesn't require a separate server process and allows access to the database using a nonstandard variant of the SQL query language. The sqlite npm package allows you to interact with SQLite databases in a Node.js environment.
Create a Database
This code demonstrates how to create an in-memory SQLite database, create a table, insert data, and query the data.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
for (let i = 0; i < 10; i++) {
stmt.run('Ipsum ' + i);
}
stmt.finalize();
db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {
console.log(row.id + ': ' + row.info);
});
});
db.close();
Open an Existing Database
This code demonstrates how to open an existing SQLite database file, query data from a table, and print the results.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database('example.db');
db.serialize(() => {
db.each('SELECT rowid AS id, info FROM lorem', (err, row) => {
console.log(row.id + ': ' + row.info);
});
});
db.close();
Parameterized Queries
This code demonstrates how to use parameterized queries to prevent SQL injection attacks. It shows how to insert and query data using placeholders.
const sqlite3 = require('sqlite3').verbose();
const db = new sqlite3.Database(':memory:');
db.serialize(() => {
db.run('CREATE TABLE lorem (info TEXT)');
const stmt = db.prepare('INSERT INTO lorem VALUES (?)');
stmt.run('Ipsum 1');
stmt.finalize();
db.get('SELECT info FROM lorem WHERE info = ?', ['Ipsum 1'], (err, row) => {
console.log(row.info);
});
});
db.close();
better-sqlite3 is a wrapper for SQLite3 that is faster and simpler to use than the sqlite package. It provides a more synchronous API, which can be easier to work with in many cases. Unlike sqlite, better-sqlite3 does not require a callback for each query, making the code more readable and easier to maintain.
Sequelize is a promise-based Node.js ORM for Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. It features solid transaction support, relations, eager and lazy loading, read replication, and more. While it supports SQLite, it is more complex and feature-rich compared to the sqlite package, making it suitable for larger applications with more complex database interactions.
Knex.js is a SQL query builder for PostgreSQL, MySQL, MariaDB, SQLite3, and Oracle. It is designed to be flexible and powerful, allowing you to build complex queries with ease. Knex.js can be used as a query builder in conjunction with an ORM or as a standalone query builder. It provides a more abstracted way to interact with databases compared to the sqlite package.
A wrapper library that adds ES6 promises and SQL-based migrations API to sqlite3 (docs).
note v4 of sqlite
has breaking changes compared to v3! Please see CHANGELOG.md
for more details.
# v4 of sqlite is targted for nodejs 10 and on.
$ npm install sqlite@4.0.0-beta.2 --save
# If you need a legacy version for an older version of nodejs
# install v3 instead, and look at the v3 branch readme for usage details
$ npm install sqlite@3 --save
This module has the same API as the original sqlite3
library (docs),
except that all its API methods return ES6 Promises and do not accept callback arguments (with the exception of each()
).
import { open } from 'sqlite'
// this is a top-level await
(async () => {
// open the database
const db = await open({
filename: '/tmp/database.db'
})
})()
or
import { open } from 'sqlite'
open({
filename: '/tmp/database.db'
}).then((db) => {
// do your thing
})
If you want to enable the database object cache
import { open } from 'sqlite'
(async () => {
const db = await open({
filename: '/tmp/database.db',
cache: true
})
})()
You can use an alternative library to sqlite3
as long as it conforms to the sqlite3
API.
For example, using sqlite3-offline
:
import { open } from 'sqlite'
(async () => {
const db = await open({
filename: '/tmp/database.db',
cache: true
})
})()
open
config params
// db is an instance of Sqlite3Database
// which is a wrapper around `sqlite3#Database`
const db = await open({
/**
* Valid values are filenames, ":memory:" for an anonymous in-memory
* database and an empty string for an anonymous disk-based database.
* Anonymous databases are not persisted and when closing the database
* handle, their contents are lost.
*/
filename: string
/**
* One or more of sqlite3.OPEN_READONLY, sqlite3.OPEN_READWRITE and
* sqlite3.OPEN_CREATE. The default value is OPEN_READWRITE | OPEN_CREATE.
*/
mode?: OpenDatabaseEnum
/**
* Use an alternative library instead of sqlite3. The interface of the library must
* conform to `sqlite3`.
*
* The default is to use `sqlite3` as the driver.
*
* @see https://github.com/mapbox/node-sqlite3/wiki/API
*/
driver?: any
/**
* If true, uses the `sqlite3` built-in database object cache to avoid opening the same
* database multiple times.
*
* Does not apply if `driver` is defined.
*
* @see https://github.com/mapbox/node-sqlite3/wiki/Caching
*/
cached?: boolean
/**
* Enables verbose mode.
*
* This only applies to the `sqlite3` driver.
*/
verbose?: boolean
})
src/**/__tests__
directory for more example usagesdocs/
directory for full documentation.sqlite3
library API docsawait db.exec('CREATE TABLE tbl (col TEXT)')
await db.exec('INSERT INTO tbl VALUES ("test")')
const result = await db.get('SELECT col FROM tbl WHERE col = ?', 'test')
// { col: 'test' }
const result = await db.get('SELECT col FROM tbl WHERE col = ?', ['test'])
// { col: 'test' }
const result = await db.get('SELECT col FROM tbl WHERE col = :test', {
':test': 'test'
})
// { col: 'test' }
const result = await db.all('SELECT col FROM tbl')
// [{ col: 'test' }]
const result = await db.run(
'INSERT INTO tbl (col) VALUES (?)',
'foo'
)
/*
{
// row ID of the inserted row
lastId: 1,
// instance of Sqlite3Statement
// which is a wrapper around `sqlite3#Statement`
stmt: <Sqlite3Statement>
}
*/
const result = await db.run('INSERT INTO tbl(col) VALUES (:col)', {
':col': 'something'
})
const result = await db.run(
'UPDATE tbl SET col = ? WHERE col = ?',
'foo',
'test'
)
/*
{
// number of rows changed
changes: 1,
// instance of Sqlite3Statement
// which is a wrapper around `sqlite3#Statement`
stmt: <Sqlite3Statement>
}
*/
// stmt is an instance of Sqlite3Statement
// which is a wrapper around `sqlite3#Statement`
const stmt = await db.prepare('SELECT col FROM tbl WHERE 1 = ? AND 5 = ?5')
await stmt.bind({ 1: 1, 5: 5 })
let result = await stmt.get()
// { col: 'some text' }
const stmt = await db.prepare(
'SELECT col FROM tbl WHERE 13 = @thirteen ORDER BY col DESC'
)
const result = await stmt.all({ '@thirteen': 13 })
Useful if you need to call methods that are not supported yet.
const rawDb = db.getDatabaseInstance()
const rawStatement = stmt.getStatementInstance()
await db.close()
This module is compatible with sql-template-strings.
import SQL from 'sql-template-strings'
const book = 'harry potter';
const author = 'J. K. Rowling';
const data = await db.all(SQL`SELECT author FROM books WHERE name = ${book} AND author = ${author}`);
This module comes with a lightweight migrations API that works with SQL-based migration files
With default configuration, you can create a migrations/
directory in your project with SQL files,
and call the migrate()
method to run the SQL in the directory against the database.
See this project's migrations/
folder for examples.
await db.migrate({
/**
* If true, will force the migration API to rollback and re-apply the latest migration over
* again each time when Node.js app launches.
*/
force?: boolean
/**
* Migrations table name. Default is 'migrations'
*/
table?: string
/**
* Path to the migrations folder. Default is `path.join(process.cwd(), 'migrations')`
*/
migrationsPath?: string
})
See the docs
directory for full documentation.
The MIT License © 2020-present Kriasoft / Theo Gravity. All rights reserved.
Made with ♥ by Konstantin Tarkus (@koistya), Theo Gravity and contributors
FAQs
SQLite client for Node.js applications with SQL-based migrations API written in Typescript
The npm package sqlite receives a total of 177,660 weekly downloads. As such, sqlite popularity was classified as popular.
We found that sqlite demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 3 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.