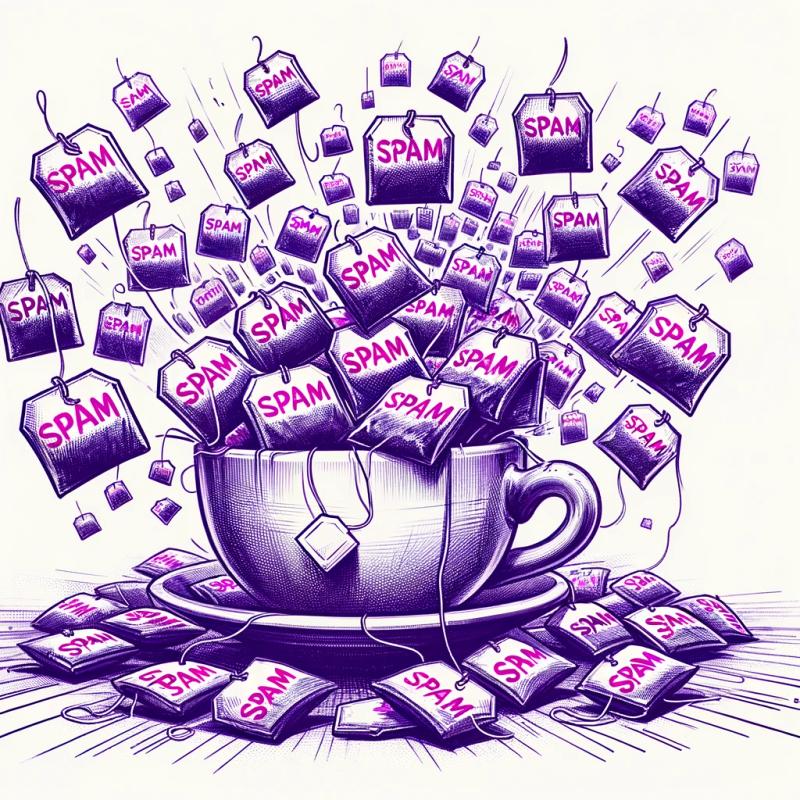
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
stdout-stderr
Advanced tools
Readme
mock stdout and stderr
Usage:
const {stdout, stderr} = require('stdout-stderr')
stdout.start() // start mocking stdout
console.log('writing to stdout') // this will not be displayed
stdout.stop() // stop mocking stdout
assert(stdout.output === 'writing to stdout') // view the output
/* options */
stdout.stripColor = false // disable automatic ansi code stripping
stdout.print = true // also output to screen
This uses the debug module so you can also see the output by setting DEBUG=stdout|stderr|*
.
FAQs
mock stdout and stderr
The npm package stdout-stderr receives a total of 193,686 weekly downloads. As such, stdout-stderr popularity was classified as popular.
We found that stdout-stderr demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.