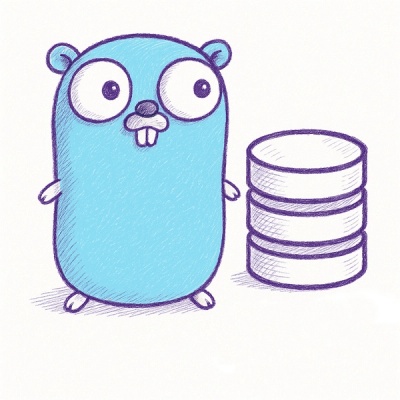
Security News
Official Go SDK for MCP in Development, Stable Release Expected in August
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
styled-dropdown-component
Advanced tools
The bootstrap dropdown component created with styled-components
The bootstrap dropdown component made with styled-components.
This is a modular approach to use bootstrap components for quick prototypes, as an entrypoint of your own component library, or if you need just one bootstrap component for your application. To work with ease with any other libary or framework this component is built with styled-components.
Note: this component has a peer dependency on
styled-components
> v4. To use this component you also need tonpm i styled-components -S
.
$ npm i styled-dropdown-component -S
or
$ yarn add styled-dropdown-component
For detailed information take a look at the documentation.
To use HTML that uses the Boostrap style, use styled-base-components.
Note: if you want this example to work you need to install the
styled-button-component
as well.
/*
if you installed `styled-bootstrap-components` use
import { ... } from 'styled-bootstrap-components'
instead.
*/
import React from 'react';
import { Button } from 'styled-button-component';
import {
Dropdown,
DropdownItem,
DropdownMenu,
} from 'styled-dropdown-component';
export class SimpleDropdown extends React.Component {
constructor(props) {
super();
this.state = {
hidden: true,
};
}
handleOpenCloseDropdown() {
this.setState({
hidden: !this.state.hidden,
});
}
render() {
const { hidden } = this.state;
return (
<Dropdown>
<Button
secondary
dropdownToggle
onClick={() => this.handleOpenCloseDropdown()}
>
Dropdown Button
</Button>
<DropdownMenu hidden={hidden}>
<DropdownItem>Action</DropdownItem>
<DropdownItem>Another action</DropdownItem>
<DropdownItem>Something else here</DropdownItem>
</DropdownMenu>
</Dropdown>
);
}
};
Properties which can be added to the component to change the visual appearance.
active
only on DropdownItem Type: booleanhidden
only on DropdownMenu Type: booleannoRadius
only on DropdownMenu Type: booleanMIT © Lukas Aichbauer
FAQs
The bootstrap dropdown component created with styled-components
The npm package styled-dropdown-component receives a total of 181 weekly downloads. As such, styled-dropdown-component popularity was classified as not popular.
We found that styled-dropdown-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.
Security News
New research reveals that LLMs often fake understanding, passing benchmarks but failing to apply concepts or stay internally consistent.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.