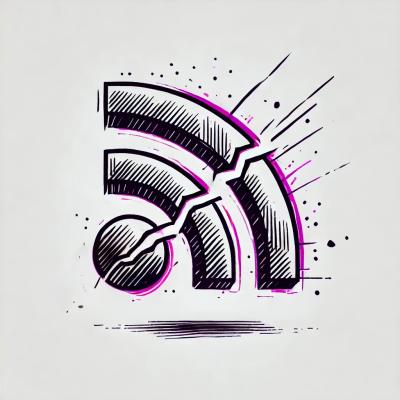
Security News
CISA Kills Off RSS Feeds for KEVs and Cyber Alerts
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
svelte-forms-lib
Advanced tools
This is a lightweight library for managing forms in Svelte, with an Formik inspired API.
This module is distributed via npm which is bundled with node and
should be installed as one of your project's dependencies
:
npm install svelte-forms-lib
This package also depends on
svelte
. Please make sure you have it installed as well.
<script>
import { createForm } from "svelte-forms-lib";
const { form, handleChange, handleSubmit } = createForm({
initialValues: {
name: "",
email: ""
},
onSubmit: values => {
// make form submission request with `values`
}
})
</script>
<form on:submit={handleSubmit}>
<label for="name">Name</label>
<input
type="text"
name="name"
bind:value={$form.name}
on:change={handleChange}
/>
<label>Email</label>
<input
type="email"
name="email"
bind:value={$form.email}
on:change={handleChange}
/>
<button type="submit">Submit</button>
</form>
The createForm
function requires at minimum a initialValues
object which contains the initial state of the form and a submit
function which will be called upon submitting the form.
Because the library is built using the Store API in Svelte, the values exposed by createForm
are observables.
// all observables returned by `createForm`
const { form, errors, touched, isValid, isSubmitting, isValidating, state } = createForm({...})
Within the template they can be read using the $
prefix i.e. $form
, $errors
. For example to access isValid
we'll use the $
prefix in the template
<p>This form is {$isValid} </p>
This library works best with yup for form validation.
<script>
import { createForm } from "svelte-forms-lib";
import yup from "yup";
const { form, errors, handleChange, handleSubmit } = createForm({
initialValues: {
name: "",
email: ""
},
validationSchema: yup.object().shape({
name: yup.string().required(),
email: yup.string().email().required()
}),
onSubmit: values => {
// make form submission request with `values`
}
})
</script>
<form on:submit={handleSubmit}>
<label for="name">Name</label>
<input
type="text"
name="name"
bind:value={$form.name}
on:change={handleChange}
/>
{#if $errors.name}
<em>{$errors.name}</em>
{/if}
<label for="email">Email</label>
<input
type="email"
name="email"
bind:value={$form.email}
on:change={handleChange}
/>
{#if $errors.email}
<em>{$errors.email}</em>
{/if}
<button type="submit">Submit</button>
</form>
Custom validation is also possible:
<script>
import { createForm } from "svelte-forms-lib";
import yup from "yup";
const { form, errors, handleChange, handleSubmit } = createForm({
initialValues: {
name: "",
email: ""
},
validate: values => {
let error = {};
if (values.name === '') {
error.name = "Name is required"
}
if (values.email === '') {
error.email = "Email is required"
}
return error;
},
onSubmit: values => {
// make form submission request with `values`
}
})
</script>
<form on:submit={handleSubmit}>
<label for="name">Name</label>
<input
type="text"
name="name"
bind:value={$form.name}
on:change={handleChange}
/>
{#if $errors.name}
<em>{$errors.name}</em>
{/if}
<label for="email">Email</label>
<input
type="email"
name="email"
bind:value={$form.email}
on:change={handleChange}
/>
{#if $errors.email}
<em>{$errors.email}</em>
{/if}
<button type="submit">Submit</button>
</form>
Currently custom validation is only run when submitting the form. Field validation will be added in the near future.
FAQs
Svelte forms lib - A lightweight library for managing forms in Svelte v3
The npm package svelte-forms-lib receives a total of 4,746 weekly downloads. As such, svelte-forms-lib popularity was classified as popular.
We found that svelte-forms-lib demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CISA is discontinuing official RSS support for KEV and cybersecurity alerts, shifting updates to email and social media, disrupting automation workflows.
Security News
The MCP community is launching an official registry to standardize AI tool discovery and let agents dynamically find and install MCP servers.
Research
Security News
Socket uncovers an npm Trojan stealing crypto wallets and BullX credentials via obfuscated code and Telegram exfiltration.