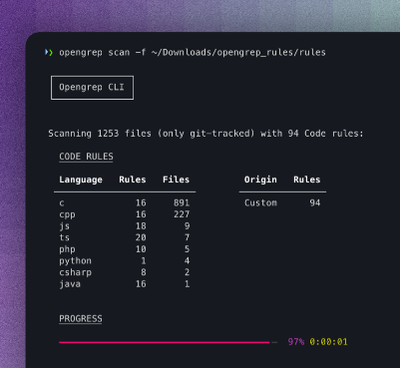
Security News
Opengrep Emerges as Open Source Alternative Amid Semgrep Licensing Controversy
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
svg-to-pdfkit
Advanced tools
The svg-to-pdfkit npm package allows you to render SVG images into PDF documents using the PDFKit library. This is useful for generating PDFs that include vector graphics, which can be scaled without loss of quality.
Render SVG to PDF
This feature allows you to render an SVG string into a PDF document. The code sample demonstrates how to create a PDF document, render an SVG circle into it, and save the output as a PDF file.
const PDFDocument = require('pdfkit');
const fs = require('fs');
const SVGtoPDF = require('svg-to-pdfkit');
const doc = new PDFDocument();
doc.pipe(fs.createWriteStream('output.pdf'));
const svg = '<svg height="100" width="100"><circle cx="50" cy="50" r="40" stroke="black" stroke-width="3" fill="red" /></svg>';
SVGtoPDF(doc, svg, 0, 0);
doc.end();
Positioning SVG in PDF
This feature allows you to specify the position where the SVG should be rendered in the PDF document. The code sample demonstrates how to render an SVG circle at coordinates (100, 150) in the PDF.
const PDFDocument = require('pdfkit');
const fs = require('fs');
const SVGtoPDF = require('svg-to-pdfkit');
const doc = new PDFDocument();
doc.pipe(fs.createWriteStream('output.pdf'));
const svg = '<svg height="100" width="100"><circle cx="50" cy="50" r="40" stroke="black" stroke-width="3" fill="red" /></svg>';
SVGtoPDF(doc, svg, 100, 150);
doc.end();
Scaling SVG in PDF
This feature allows you to scale the SVG when rendering it into the PDF document. The code sample demonstrates how to render an SVG circle with a width and height of 200 units in the PDF.
const PDFDocument = require('pdfkit');
const fs = require('fs');
const SVGtoPDF = require('svg-to-pdfkit');
const doc = new PDFDocument();
doc.pipe(fs.createWriteStream('output.pdf'));
const svg = '<svg height="100" width="100"><circle cx="50" cy="50" r="40" stroke="black" stroke-width="3" fill="red" /></svg>';
SVGtoPDF(doc, svg, 0, 0, { width: 200, height: 200 });
doc.end();
PDFKit is a JavaScript library for generating PDF documents, but it does not natively support rendering SVG. It is often used in conjunction with svg-to-pdfkit to achieve SVG rendering in PDFs.
svg2pdf.js is a library that converts SVG elements to PDF using jsPDF. It provides similar functionality to svg-to-pdfkit but is designed to work with jsPDF instead of PDFKit.
canvg is a library that converts SVG into Canvas. While it does not directly convert SVG to PDF, it can be used in combination with libraries that convert Canvas to PDF to achieve similar results.
Insert SVG into a PDF document created with PDFKit.
npm install svg-to-pdfkit --save
SVGtoPDF(doc, svg, x, y, options);
If you prefer, you can add the function to the PDFDocument prototype:
PDFDocument.prototype.addSVG = function(svg, x, y, options) {
return SVGtoPDF(this, svg, x, y, options), this;
};
And then simply call:
doc.addSVG(svg, x, y, options);
doc [PDFDocument] = the PDF document created with PDFKit
svg [SVGElement or string] = the SVG object or XML code
x, y [number] = the position where the SVG will be added
options [Object] = >
- width, height [number] = initial viewport, by default it's the page dimensions
- preserveAspectRatio [string] = override alignment of the SVG content inside its viewport
- useCSS [boolean] = use the CSS styles computed by the browser (for SVGElement only)
- fontCallback [function] = function called to get the fonts, see source code
- imageCallback [function] = same as above for the images (for Node.js)
- documentCallback [function] = same as above for the external SVG documents
- colorCallback [function] = function called to get color, making mapping to CMYK possible
- warningCallback [function] = function called when there is a warning
- assumePt [boolean] = assume that units are PDF points instead of SVG pixels
- precision [number] = precision factor for approximative calculations (default = 3)
In the browser, it's easier to register fonts (see here how) before calling SVGtoPDF. SVGtoPDF doesn't wait for font loading with asynchronous XMLHttpRequest.
Make sure to name the fonts with the exact pattern 'MyFont', 'MyFont-Bold', 'MyFont-Italic', 'MyFont-BoldItalic' (case sensitive), if the font is named font-family="MyFont" in the svg. Missing Bold, Italic, BoldItalic fonts are simulated with stroke and skew angle.
If your fonts don't follow this pattern, or you want to register fonts at the moment they are encountered in the svg, you can use a custom fontCallback function.
https://alafr.github.io/SVG-to-PDFKit/examples/demo.htm
https://alafr.github.io/SVG-to-PDFKit/examples/options.htm
https://runkit.com/alafr/5a1377ff160182001232a91d
FAQs
Insert SVG into a PDF document created with PDFKit
The npm package svg-to-pdfkit receives a total of 123,576 weekly downloads. As such, svg-to-pdfkit popularity was classified as popular.
We found that svg-to-pdfkit demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Opengrep forks Semgrep to preserve open source SAST in response to controversial licensing changes.
Security News
Critics call the Node.js EOL CVE a misuse of the system, sparking debate over CVE standards and the growing noise in vulnerability databases.
Security News
cURL and Go security teams are publicly rejecting CVSS as flawed for assessing vulnerabilities and are calling for more accurate, context-aware approaches.