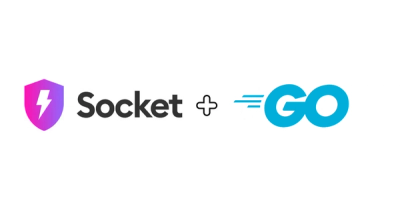
Product
Go Support Is Now Generally Available
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
tangem-sdk-react-native
Advanced tools
The Tangem card is a self-custodial hardware wallet for blockchain assets. The main functions of Tangem cards are to securely create and store a private key from a blockchain wallet and sign blockchain transactions. The Tangem card does not allow users to import/export, backup/restore private keys, thereby guaranteeing that the wallet is unique and unclonable.
iOS 11+ (CoreNFC is required), Xcode 11+ SDK can be imported to iOS 11, but it will work only since iOS 13.
Android with minimal SDK version of 21 and a device with NFC support
npm install tangem-sdk-react-native
Add the following intent filters and metadata tag to your app AndroidManifest.xml
<intent-filter>
<action android:name="android.nfc.action.NDEF_DISCOVERED"/>
<category android:name="android.intent.category.DEFAULT"/>
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.TECH_DISCOVERED"/>
</intent-filter>
<meta-data android:name="android.nfc.action.TECH_DISCOVERED" android:resource="@xml/nfc_tech_filter" />
Create the file android/src/main/res/xml/nfc_tech_filter.xml
and add the following content:
<resources>
<tech-list>
<tech>android.nfc.tech.IsoDep</tech>
<tech>android.nfc.tech.Ndef</tech>
<tech>android.nfc.tech.NfcV</tech>
</tech-list>
</resources>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.reactnativenfcdemo"
android:versionCode="1"
android:versionName="1.0">
<uses-permission android:name="android.permission.INTERNET" />
<uses-permission android:name="android.permission.SYSTEM_ALERT_WINDOW"/>
<uses-permission android:name="android.permission.NFC" />
<uses-sdk
android:minSdkVersion="16"
android:targetSdkVersion="22" />
<application
android:name=".MainApplication"
android:allowBackup="true"
android:label="@string/app_name"
android:icon="@mipmap/ic_launcher"
android:theme="@style/AppTheme">
<activity
android:name=".MainActivity"
android:screenOrientation="portrait"
android:label="@string/app_name"
android:launchMode="singleTask"
android:configChanges="keyboard|keyboardHidden|orientation|screenSize"
android:windowSoftInputMode="adjustResize">
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.NDEF_DISCOVERED"/>
<category android:name="android.intent.category.DEFAULT"/>
</intent-filter>
<intent-filter>
<action android:name="android.nfc.action.TECH_DISCOVERED"/>
</intent-filter>
<meta-data android:name="android.nfc.action.TECH_DISCOVERED" android:resource="@xml/nfc_tech_filter" />
</activity>
<activity android:name="com.facebook.react.devsupport.DevSettingsActivity" />
</application>
</manifest>
project.pbxproj
)LIBRARY_SEARCH_PATHS = (
"\"$(TOOLCHAIN_DIR)/usr/lib/swift/$(PLATFORM_NAME)\"",
- "\"$(TOOLCHAIN_DIR)/usr/lib/swift-5.0/$(PLATFORM_NAME)\"",
"\"$(inherited)\"",
);
Configure your app to detect NFC tags. Turn on Near Field Communication Tag Reading under the Capabilities tab for the project’s target (see Add a capability to a target).
Add the NFCReaderUsageDescription key as a string item to the Info.plist file. For the value, enter a string that describes the reason the app needs access to the device’s NFC reader:
<key>NFCReaderUsageDescription</key>
<string>Some reason</string>
A000000812010208
and D2760000850101
.<key>com.apple.developer.nfc.readersession.iso7816.select-identifiers</key>
<array>
<string>A000000812010208</string>
<string>D2760000850101</string>
</array>
<key>UIRequiredDeviceCapabilities</key>
<array>
<string>nfc</string>
</array>
Tangem SDK is a self-sufficient solution that implements a card abstraction model, methods of interaction with the card and interactions with the user via UI.
The easiest way to use the SDK is to call basic methods. The basic method performs one or more operations and, after that, calls completion block with success or error.
When calling basic methods, there is no need to show the error to the user, since it will be displayed on the NFC popup before it's hidden.
Method RNTangemSdk.startSession()
is needed before running any other method in android, calling this method will ask the user to enable the NFC in case of NFC disabled.
RNTangemSdk.startSession();
It's recommended to check for NFC status before running any other method and call this method again in case of disabled NFC
Method RNTangemSdk.stopSession()
will stop NFC Manager and it's recommended to be called to stop the session.
RNTangemSdk.stopSession();
Method RNTangemSdk.scanCard()
is needed to obtain information from the Tangem card. Optionally, if the card contains a wallet (private and public key pair), it proves that the wallet owns a private key that corresponds to a public one.
RNTangemSdk.scanCard();
Method RNTangemSdk.sign()
allows you to sign one or multiple hashes. The SIGN command will return a corresponding array of signatures.
const cid = "bb03000000000004";
RNTangemSdk.sign(cid, [
"44617461207573656420666f722068617368696e67",
"4461746120666f7220757365642068617368696e67",
]);
Method RNTangemSdk.createWallet()
will create a new wallet on the card. A key pair WalletPublicKey
/ WalletPrivateKey
is generated and securely stored in the card.
var cid = "bb03000000000004";
RNTangemSdk.createWallet(cid);
Method RNTangemSdk.purgeWallet()
deletes all wallet data.
var cid = "bb03000000000004";
RNTangemSdk.purgeWallet(cid);
Access code (PIN1) restricts access to the whole card. App must submit the correct value of Access code in each command. Passcode (PIN2) is required to sign a transaction or to perform some other commands entailing a change of the card state.
var cid = "bb03000000000004";
var pin = "123456";
//TangemSdk.changePin1(cid);
RNTangemSdk.changePin2(cid, pin);
Passing empty string as PIN will trigger SDK dialog for entering the PIN code by user
Method RNTangemSdk.getNFCStatus()
will return current NFC Status which is supported on the device or is NFC enabled on the device.
RNTangemSdk.getNFCStatus();
with RNTangemSdk.on()
and RNTangemSdk.removeListener()
you should be able to add/remove listener on the certain events
Supported Events: NFCStateChange
RNTangemSdk.on("NFCStateChange", (enabled) => {
console.log(enabled);
});
fetch
functionNo static method delimiterOffset(Ljava/lang/String;IILjava/lang/String;)I in class Lokhttp3/internal/Util; or its super classes (declaration of 'okhttp3.internal.Util' appears in base.apk!classes3.dex)
Include this dependencies in android/app/build.gradle
file in dependencies
section
implementation "com.squareup.okhttp3:okhttp:4.2.1"
implementation "com.squareup.okhttp3:logging-interceptor:4.2.1"
implementation "com.squareup.okhttp3:okhttp-urlconnection:4.2.1"
FAQs
React Native Tangem Sdk
The npm package tangem-sdk-react-native receives a total of 11 weekly downloads. As such, tangem-sdk-react-native popularity was classified as not popular.
We found that tangem-sdk-react-native demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Socket's Go support is now generally available, bringing automatic scanning and deep code analysis to all users with Go projects.
Security News
vlt adds real-time security selectors powered by Socket, enabling developers to query and analyze package risks directly in their dependency graph.
Security News
CISA extended MITRE’s CVE contract by 11 months, avoiding a shutdown but leaving long-term governance and coordination issues unresolved.