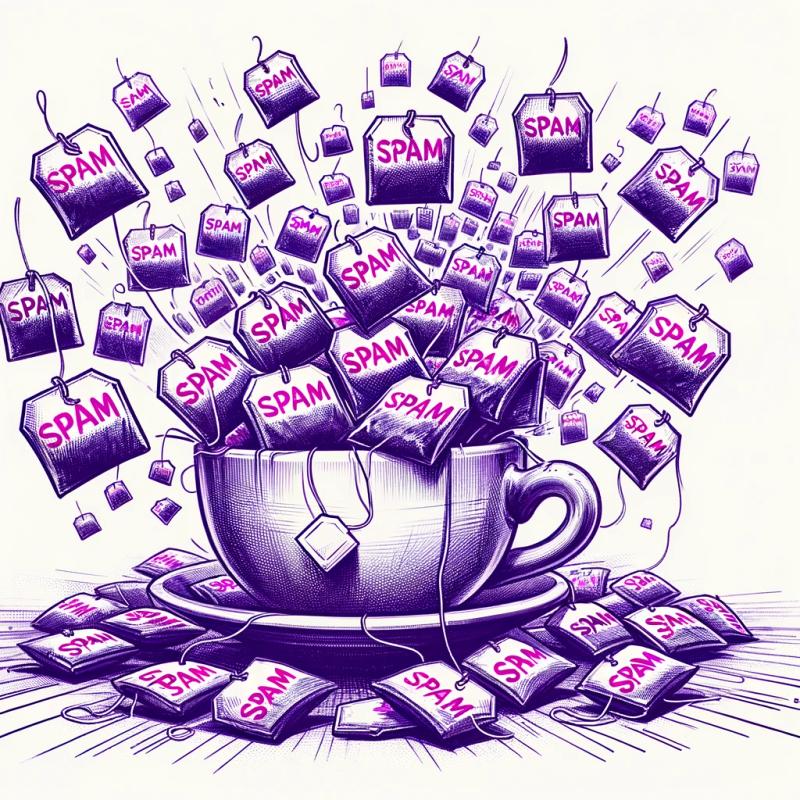
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
transform-async-iterable
Advanced tools
Readme
transform-async-iterable
is a TypeScript package that provides a utility function for transforming iterables and async iterables with a custom transformation function. It enables developers to easily apply transformations to each item in an iterable and generate a new iterable with the transformed results. This module is particularly useful when dealing with collections of data and needing to perform consistent operations on each item.
Install it via your preferred package manager:
npm install transform-async-iterable
or
yarn add transform-async-iterable
Here's how you can use transform-async-iterable
in your TypeScript/JavaScript project:
transform
function:import transform from 'transform-async-iterable';
const transformFunction = (item) => {
// Apply your transformation logic here
return transformedItem;
};
const data = [1, 2, 3, 4, 5];
transform
function, passing in the iterable and transformation function:const transformedData = transform(data, transformFunction);
for await (const item of transformedData) {
// Use the transformed item in your code
}
transform<T, R>(it: Iterable<T> | AsyncIterable<T>, fn: CallbackFn<T, R>): AsyncGenerator<R>
The transform
function accepts two arguments:
it
: The iterable or async iterable to be transformed.fn
: The transformation function that will be applied to each item in the iterable.The transformation function, fn
, is defined using the CallbackFn
type:
type CallbackFn<T, R> = { (item: T): undefined | R | Iterable<R> | AsyncIterable<R> | Promise<R | Iterable<R> | AsyncIterable<R>> };
This allows for flexible transformation operations that can return single transformed item or multiple items in an iterable.
The transformation function can indicate to remove an item from the produced iterable via returning undefined
value.
Note: the
transform()
call always returns an async iterable object, even when the input and the transform function could work in sync mode.
transformSync<T, R>(it: Iterable<T>, fn: SyncCallbackFn<T, R>): Generator<R>
The transformSync
function accepts two arguments:
it
: The iterable to be transformed.fn
: The transformation function that will be applied to each item in the iterable.The transformation function, fn
, is defined using the SyncCallbackFn
type:
type SyncCallbackFn<T, R> = { (item: T): undefined | R | Iterable<R> };
This allows for flexible transformation operations that can return single transformed item or multiple items in an iterable.
The transformation function can indicate to remove an item from the produced iterable via returning undefined
value.
Here are some examples to demonstrate the usage:
import transform from 'transform-async-iterable';
const data = [1, 2, 3, 4, 5];
const transformedData = transform(data, (item) => {
return item * 2;
});
for await (const item of transformedData) {
console.log(item);
}
Output:
2
4
6
8
10
import transform from 'transform-async-iterable';
async function* fetchData() {
yield { id: 1, name: 'John' };
yield { id: 2, name: 'Jane' };
}
const capitalizeName = async (item) => {
const capitalized = item.name.toUpperCase();
return { ...item, name: capitalized };
};
const transformedData = transform(fetchData(), capitalizeName);
for await (const item of transformedData) {
console.log(item);
}
Output:
{ id: 1, name: 'JOHN' }
{ id: 2, name: 'JANE' }
import transform from 'transform-async-iterable';
async function* fetchData() {
yield { id: '1', name: 'John' };
yield { id: '2', name: 'Jane' };
yield { id: '3', name: 'Doe' };
yield { id: '4', name: 'Steve,Emma' };
}
const transformedData = transform(fetchData(), (item) => {
if (item.name === 'Doe') return; // remove from the collection
if (item.name.includes(',')) { // split names to two objects
return item.name.split(',')
.map((name, idx) => ({
id: item.id + '-' + idx,
name: name,
}));
}
return item; // keep the rest as-is
});
for await (const item of transformedData) {
console.log(item);
}
Output:
{ id: '1', name: 'John' }
{ id: '2', name: 'Jane' }
{ id: '4-0', name: 'Steve' }
{ id: '4-1', name: 'Emma' }
import transform from 'transform-async-iterable';
async function* fetchData() {
yield 'a';
yield 'b';
yield '\n';
yield 'c';
yield 'd';
yield '\n';
}
let buffer = '';
const transformedData = transform(fetchData(), (item) => {
if (item === '\n') {
const line = buffer;
buffer = '';
return line;
}
buffer += item;
});
for await (const item of transformedData) {
console.log(item);
}
Output:
ab
cd
Contributions to transform-async-iterable
are welcome! If you have any bug reports, feature requests, or improvements, please open an issue on the GitHub repository.
transform-async-iterable
is licensed under the MIT License.
FAQs
TransformIt is a TypeScript package that provides a utility function for transforming iterables and async iterables with a custom transformation function. It enables developers to easily apply transformations to each item in an iterable and generate a new
The npm package transform-async-iterable receives a total of 22 weekly downloads. As such, transform-async-iterable popularity was classified as not popular.
We found that transform-async-iterable demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.