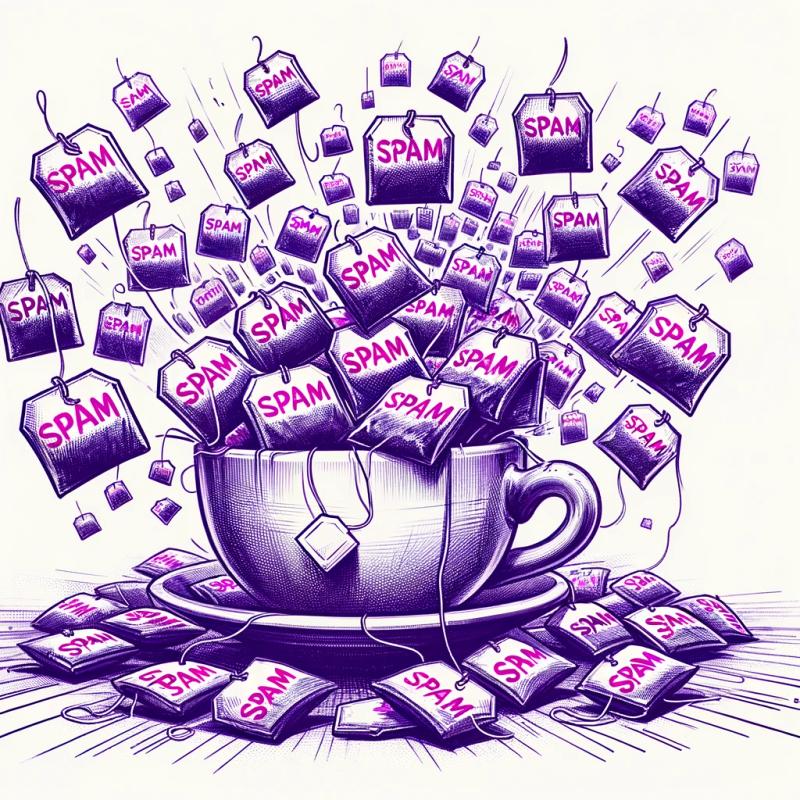
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
ts-sinon
Advanced tools
Readme
Sinon extension providing functions to:
npm install --save-dev ts-sinon
or
yarn add --dev ts-sinon
Importing stubObject function:
import { stubObject } from "ts-sinon";
import * as sinon from "ts-sinon";
const stubObject = sinon.stubObject;
Stub all object methods:
class Test {
method() { return "original" }
}
const test = new Test();
const testStub = stubObject<Test>(test);
testStub.method.returns("stubbed");
expect(testStub.method()).to.equal("stubbed");
Partial stub:
class Test {
public someProp: string = "test";
methodA() { return "A: original" }
methodB() { return "B: original" }
}
const test = new Test();
// second argument must be existing class method name, in this case only "methodA" or "methodB" are accepted.
const testStub = stubObject<Test>(test, ["methodA"]);
expect(testStub.methodA()).to.be.undefined;
expect(testStub.methodB()).to.equal("B: original");
Stub with predefined return values (type-safe):
class Test {
method() { return "original" }
}
const test = new Test();
const testStub = stubObject<Test>(test, { method: "stubbed" });
expect(testStub.method()).to.equal("stubbed");
Importing stubInterface function:
import { stubInterface } from "ts-sinon";
import * as sinon from "ts-sinon";
const stubInterface = sinon.stubInterface;
Interface stub (stub all methods):
interface Test {
method(): string;
}
const testStub = stubInterface<Test>();
expect(testStub.method()).to.be.undefined;
testStub.method.returns("stubbed");
expect(testStub.method()).to.equal("stubbed");
Interface stub with predefined return values (type-safe):
interface Test {
method(): string;
}
// method property has to be the same type as method() return type
const testStub = stubInterface<Test>({ method: "stubbed" });
expect(testStub.method()).to.equal("stubbed");
Importing stubConstructor function:
import { stubConstructor } from "ts-sinon";
import * as sinon from "ts-sinon";
const stubConstructor = sinon.stubConstructor;
Object constructor stub (stub all methods):
class Test {
public someVar: number = 10;
method(): string {
return "value";
}
}
// type will be guessed automatically
const testStub = stubConstructor(Test);
expect(testStub.method()).to.be.undefined;
testStub.method.returns("stubbed");
expect(testStub.method()).to.equal("stubbed");
expect(testStub.someVar).to.equal(10);
testStub.someVar = 20;
expect(testStub.someVar).to.equal(20);
class Test {
constructor(public someVar: string, y: boolean) {}
// ...
}
// it won't allow to pass incorrect args
const testStub = stubConstructor(Test, "someValue", true);
expect(testStub.someVar).to.equal("someValue");
By importing 'ts-sinon' you have access to all sinon methods.
import sinon, { stubInterface } from "ts-sinon";
const functionStub = sinon.stub();
const spy = sinon.spy();
// ...
or
import * as tsSinon from "ts-sinon"
const functionStub = tsSinon.default.stub();
const spy = tsSinon.default.spy();
const tsStubInterface = tsSinon.stubInterface<T>();
// ...
npm test
FAQs
sinon library extension to stub whole object and interfaces
The npm package ts-sinon receives a total of 64,331 weekly downloads. As such, ts-sinon popularity was classified as popular.
We found that ts-sinon demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.