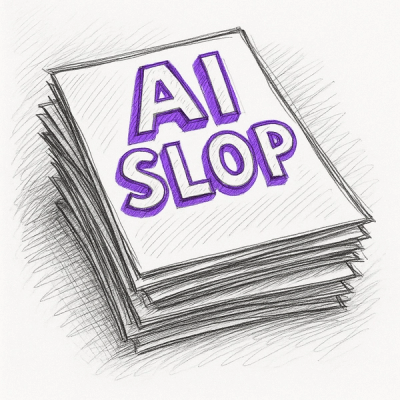
Security News
Django Joins curl in Pushing Back on AI Slop Security Reports
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
tslint-eslint-rules
Advanced tools
You want to code in TypeScript but miss all the rules available in ESLint?
Now you can combine both worlds by using this TSLint plugin!
npm install --save-dev tslint-eslint-rules
tslint-eslint-rules
folder:In your tslint.json
file, add the rulesDirectory
property, e.g:
{
"rulesDirectory": "node_modules/tslint-eslint-rules/dist/rules",
"rules": {
"no-constant-condition": true
}
}
You can also pass an array of strings to the rulesDirectory
property to combine this plugin with other community custom rules.
In your tslint.json
file, insert the rules as described below.
The list below shows all the existing ESLint rules and the similar rules available in TSLint.
The following rules point out areas where you might have made mistakes.
comma-dangle => trailing-comma (native)
Description: disallow or enforce trailing commas (recommended)
Usage
"trailing-comma": [
true,
{
"multiline": "never",
"singleline": "never"
}
]
no-cond-assign => no-conditional-assignment (native)
Description: disallow assignment in conditional expressions (recommended)
Usage
"no-conditional-assignment": true
no-console => no-console (native)
Description: disallow use of console
in the node environment (recommended)
Usage
"no-console": [
true,
"debug",
"info",
"time",
"timeEnd",
"trace"
]
no-constant-condition => no-constant-condition (tslint-eslint-rules)
Description: disallow use of constant expressions in conditions (recommended)
Usage
"no-constant-condition": true
no-control-regex => no-control-regex (tslint-eslint-rules)
Description: disallow control characters in regular expressions (recommended)
Usage
"no-control-regex": true
no-debugger => no-debugger (native)
Description: disallow use of debugger
(recommended)
Usage
"no-debugger": true
no-dupe-args => not applicable to TypeScript
no-dupe-keys => no-duplicate-key (native)
Description: disallow duplicate keys when creating object literals (recommended)
Usage
"no-duplicate-key": true
no-duplicate-case => no-duplicate-case (tslint-eslint-rules)
Description: disallow a duplicate case label. (recommended)
Usage
"no-duplicate-case": true
no-empty-character-class => no-empty-character-class (tslint-eslint-rules)
Description: disallow the use of empty character classes in regular expressions (recommended)
Usage
"no-empty-character-class": true
no-empty => no-empty (native)
Description: disallow empty statements (recommended)
Usage
"no-empty": true
no-ex-assign => no-ex-assign (tslint-eslint-rules)
Description: disallow assigning to the exception in a catch
block (recommended)
Usage
"no-ex-assign": true
no-extra-boolean-cast => no-extra-boolean-cast (tslint-eslint-rules)
Description: disallow double-negation boolean casts in a boolean context (recommended)
Usage
"no-extra-boolean-cast": true
no-extra-parens => no-extra-parens (tslint-eslint-rules) TODO (low priority)
Description: disallow unnecessary parentheses
Usage
"no-extra-parens": [
true,
"functions"
]
"no-extra-parens": [
true,
"all"
]
no-extra-semi => no-extra-semi (tslint-eslint-rules)
Description: disallow unnecessary semicolons (recommended)
Usage
"no-extra-semi": true
no-func-assign => not applicable to TypeScript
no-inner-declarations => no-inner-declarations (tslint-eslint-rules)
Description: disallow function or variable declarations in nested blocks (recommended)
Usage
"no-inner-declarations": [
true,
"functions"
]
"no-inner-declarations": [
true,
"both"
]
no-invalid-regexp => no-invalid-regex (tslint-eslint-rules)
Description: disallow invalid regular expression strings in the RegExp
constructor (recommended)
Usage
"no-invalid-regexp": true
no-irregular-whitespace => no-irregular-whitespace (tslint-eslint-rules)
Description: disallow irregular whitespace outside of strings and comments (recommended)
Usage
"no-irregular-whitespace": true
no-negated-in-lhs => not applicable to TypeScript
in
expression (recommended)no-obj-calls => not applicable to TypeScript
Math
and JSON
) as functions (recommended)no-regex-spaces => no-regex-spaces (tslint-eslint-rules)
Description: disallow multiple spaces in a regular expression literal (recommended)
Usage
"no-regex-spaces": true
no-sparse-arrays => no-sparse-arrays (tslint-eslint-rules)
Description: disallow sparse arrays (recommended)
Usage
"no-sparse-arrays": true
no-unexpected-multiline => no-unexpected-multiline (tslint-eslint-rules)
Description: Avoid code that looks like two expressions but is actually one
Usage
"no-unexpected-multiline": true
no-unreachable => no-unreachable (native)
Description: disallow unreachable statements after a return, throw, continue, or break statement (recommended)
Usage
"no-unreachable": true
use-isnan => use-isnan (tslint-eslint-rules)
Description: disallow comparisons with the value NaN
(recommended)
Usage
"use-isnan": true
valid-jsdoc => valid-jsdoc (tslint-eslint-rules)
Description: Ensure JSDoc comments are valid
Usage
"valid-jsdoc": [
true,
{
"prefer": {
"return": "returns"
},
"requireReturn": false,
"requireParamDescription": true,
"requireReturnDescription": true,
"matchDescription": "^[A-Z][A-Za-z0-9\\s]*[.]$"
}
]
valid-typeof => valid-typeof (tslint-eslint-rules)
Description: Ensure that the results of typeof are compared against a valid string (recommended)
Usage
"valid-typeof": true
These are rules designed to prevent you from making mistakes. They either prescribe a better way of doing something or help you avoid footguns.
accessor-pairs => accessor-pairs (tslint-eslint-rules) TODO
Description: Enforces getter/setter pairs in objects
Usage
"accessor-pairs": [
true,
{
"getWithoutSet" : true,
"setWithoutGet" : true
}
]
block-scoped-var => accessor-pairs (tslint-eslint-rules) TODO
Description: treat var
statements as if they were block scoped
Usage
"block-scoped-var": true
complexity => complexity (tslint-eslint-rules) TODO
Description: specify the maximum cyclomatic complexity allowed in a program
Usage
"complexity": [
true,
3
]
consistent-return => consistent-return (tslint-eslint-rules) TODO
Description: require return
statements to either always or never specify values
Usage
"consistent-return": true
curly => curly (native)
Description: specify curly brace conventions for all control statements
Usage
"curly": true
default-case => switch-default (native)
Description: require default
case in switch
statements
Usage
"default-case": true
dot-location => dot-location (tslint-eslint-rules) TODO
Description: enforces consistent newlines before or after dots
Usage
"dot-location": [
true,
"object"
]
"dot-location": [
true,
"property"
]
dot-notation => dot-notation (tslint-eslint-rules) TODO
Description: encourages use of dot notation whenever possible
Usage
"dot-notation": [
true,
{
"allowKeywords": true,
"allowPattern": ""
}
]
eqeqeq => triple-equals (native)
Description: require the use of ===
and !==
Usage
"eqeqeq": [
true,
"allow-null-check"
]
guard-for-in => forin (native)
Description: make sure for-in
loops have an if
statement
Usage
"forin": true
no-alert => no-alert (tslint-eslint-rules) TODO
Description: disallow the use of alert
, confirm
, and prompt
Usage
"no-alert": true
no-caller => no-arg (native)
Description: disallow use of arguments.caller
or arguments.callee
Usage
"no-arg": true
no-case-declarations => no-case-declarations (tslint-eslint-rules) TODO
Description: disallow lexical declarations in case clauses
Usage
"no-case-declarations": true
no-div-regex => no-div-regex (tslint-eslint-rules) TODO
Description: disallow division operators explicitly at beginning of regular expression
Usage
"no-div-regex": true
no-else-return => no-else-return (tslint-eslint-rules) TODO
Description: disallow else
after a return
in an if
Usage
"no-else-return": true
no-empty-label => no-empty-label (tslint-eslint-rules) TODO
Description: disallow use of labels for anything other than loops and switches
Usage
"no-empty-label": true
no-empty-pattern => no-empty-pattern (tslint-eslint-rules) TODO
Description: disallow use of empty destructuring patterns
Usage
"no-empty-pattern": true
no-eq-null => no-eq-null (tslint-eslint-rules) TODO
Description: disallow comparisons to null without a type-checking operator
Usage
"no-eq-null": true
no-eval => no-eval (native)
Description: disallow use of eval()
Usage
"no-eval": true
no-extend-native => no-extend-native (tslint-eslint-rules) TODO
Description: disallow adding to native types
Usage
"no-extend-native": [
true,
{
"exceptions": ["Object", "String"]
}
]
no-extra-bind => no-extra-bind (tslint-eslint-rules) TODO
Description: disallow unnecessary function binding
Usage
"no-extra-bind": true
no-fallthrough => no-switch-case-fall-through (native)
Description: disallow fallthrough of case
statements (recommended)
Usage
"no-fallthrough": true
no-floating-decimal => no-floating-decimal (tslint-eslint-rules) TODO
Description: disallow the use of leading or trailing decimal points in numeric literals
Usage
"no-floating-decimal": true
no-implicit-coercion => no-implicit-coercion (tslint-eslint-rules) TODO
Description: disallow the type conversions with shorter notations
Usage
"no-implicit-coercion": [
true,
{
"boolean": true,
"number": true,
"string": true
}
]
no-implied-eval => no-implied-eval (tslint-eslint-rules) TODO
Description: disallow use of eval()
-like methods
Usage
"no-implied-eval": true
no-invalid-this => no-invalid-this (tslint-eslint-rules) TODO
Description: disallow this
keywords outside of classes or class-like objects
Usage
"no-invalid-this": true
no-iterator => no-iterator (tslint-eslint-rules) TODO
Description: disallow Usage of __iterator__
property
Usage
"no-iterator": true
no-labels => no-labels (tslint-eslint-rules) TODO
Description: disallow use of labeled statements
Usage
"no-labels": true
no-lone-blocks => no-lone-blocks (tslint-eslint-rules) TODO
Description: disallow unnecessary nested blocks
Usage
"no-lone-blocks": true
no-loop-func => no-loop-func (tslint-eslint-rules) TODO
Description: disallow creation of functions within loops
Usage
"no-loop-func": true
no-magic-numbers => no-magic-numbers (tslint-eslint-rules) TODO
Description: disallow the use of magic numbers
Usage
"no-magic-numbers": [
true,
{
"ignore": [0, 1, 2],
"enforceConst": false,
"detectObjects": false
}
]
no-multi-spaces => no-multi-spaces (tslint-eslint-rules) TODO
Description: disallow use of multiple spaces
Usage
"no-multi-spaces": [
true,
{
"exceptions": [ { "ImportDeclation": true }, { "Property": false } ]
}
]
no-multi-str => no-multi-str (tslint-eslint-rules) TODO
Description: disallow use of multiline strings
Usage
"no-multi-str": true
no-native-reassign => Not applicable to TypeScript
no-new-func => no-new-func (tslint-eslint-rules) TODO
Description: disallow use of new operator for Function
object
Usage
"no-new-func": true
no-new-wrappers => no-new-wrappers (tslint-eslint-rules) TODO // ou no-construct
Description: disallows creating new instances of String
,Number
, and Boolean
Usage
"no-new-wrappers": true
no-new => no-new (tslint-eslint-rules) TODO
Description: disallow use of the new
operator when not part of an assignment or comparison
Usage
"no-new": true
no-octal-escape => no-octal-escape (tslint-eslint-rules) TODO
Description: disallow use of octal escape sequences in string literals, such as var foo = "Copyright \251";
Usage
"no-octal-escape": true
no-octal => Not applicable to TypeScript
no-param-reassign => no-param-reassign (tslint-eslint-rules) TODO
Description: disallow reassignment of function parameters
Usage
"no-param-reassign": [
true,
{
"props": false
}
]
no-process-env => no-process-env (tslint-eslint-rules) TODO
Description: disallow use of process.env
Usage
"no-process-env": true
no-proto => no-proto (tslint-eslint-rules) TODO
Description: disallow Usage of __proto__
property
Usage
"no-proto": true
no-redeclare => no-duplicate-variable (native)
Description: disallow declaring the same variable more than once (http://eslint.org/docs/rules/recommended)
Usage
"no-duplicate-variable": true
no-return-assign => no-return-assign (tslint-eslint-rules) TODO
Description: disallow use of assignment in return
statement
Usage
"no-return-assign": [
true,
"except-parens"
]
"no-return-assign": [
true,
"always"
]
no-script-url => no-script-url (tslint-eslint-rules) TODO
Description: disallow use of javascript:
urls.
Usage
"no-script-url": true
no-self-compare => no-self-compare (tslint-eslint-rules) TODO
Description: disallow comparisons where both sides are exactly the same
Usage
"no-self-compare": true
no-sequences => no-sequences (tslint-eslint-rules) TODO
Description: disallow use of the comma operator
Usage
"no-sequences": true
no-throw-literal => no-throw-literal (tslint-eslint-rules) TODO
Description: restrict what can be thrown as an exception
Usage
"no-throw-literal": true
no-unused-expressions => no-unused-expression (native)
Description: disallow Usage of expressions in statement position
Usage
"no-unused-expressions": true
no-useless-call => no-useless-call (tslint-eslint-rules) TODO
Description: disallow unnecessary .call()
and .apply()
Usage
"no-useless-call": true
no-useless-concat => no-useless-concat (tslint-eslint-rules) TODO
Description: disallow unnecessary concatenation of literals or template literals
Usage
"no-useless-concat": true
no-void => no-void (tslint-eslint-rules) TODO
Description: disallow use of the void
operator
Usage
"no-void":true
no-warning-comments => no-warning-comments (tslint-eslint-rules) TODO
Description: disallow Usage of configurable warning terms in comments e.g. TODO
or FIXME
Usage
"no-warning-comments": [
true,
{
"terms": ["todo", "fixme", "xxx"],
"location": "start"
}
]
no-with => no-with (tslint-eslint-rules) TODO
Description: disallow use of the with
statement
Usage
"no-with": true
radix => radix (native)
Description: require use of the second argument for parseInt()
Usage
"radix": true
vars-on-top => vars-on-top (tslint-eslint-rules) TODO
Description: require declaration of all vars at the top of their containing scope
Usage
"vars-on-top": true
wrap-iife => wrap-iife (tslint-eslint-rules) TODO
Description: require immediate function invocation to be wrapped in parentheses
Usage
"wrap-iife": [
true,
"inside"
]
"wrap-iife": [
true,
"outside"
]
"wrap-iife": [
true,
"any"
]
yoda => yoda (tslint-eslint-rules) TODO
Description: require or disallow Yoda conditions
Usage
"yoda": [
true,
"never"
]
"yoda": [
true,
"always"
]
These rules relate to using strict mode.
Description: controls location of Use Strict Directives
Usage
"strict": [
true,
"function"
]
"strict": [
true,
"global"
]
"strict": [
true,
"never"
]
These rules have to do with variable declarations.
init-declarations => init-declarations (tslint-eslint-rules) TODO
Description: enforce or disallow variable initializations at definition
Usage
"init-declarations": [
true,
"always"
{
"ignoreForLoopInit": false
}
]
"init-declarations": [
true,
"never"
{
"ignoreForLoopInit": false
}
]
no-catch-shadow => no-catch-shadow (tslint-eslint-rules) TODO
Description: disallow the catch clause parameter name being the same as a variable in the outer scope
Usage
"no-catch-shadow": true
no-delete-var => not applicable to TypeScript
no-label-var => no-label-var (tslint-eslint-rules) TODO
Description: disallow labels that share a name with a variable
Usage
"no-label-var": true
no-shadow-restricted-names => no-shadow-restricted-names (tslint-eslint-rules) TODO
Description: disallow shadowing of names such as arguments
Usage
"no-shadow-restricted-names": true
no-shadow => no-shadowed-variable (native)
Description: disallow declaration of variables already declared in the outer scope
Usage
"no-shadowed-variable": true
no-undef-init => no-undef-init (tslint-eslint-rules) TODO
Description: disallow use of undefined when initializing variables
Usage
"no-undef-init": true
no-undef => not applicable to TypeScript
/*global */
block (recommended)no-undefined => no-undefined (tslint-eslint-rules) TODO
Description: disallow use of undefined
variable
Usage
"no-undefined": true
no-unused-vars => no-unused-variable (native)
Description: disallow declaration of variables that are not used in the code (recommended)
Usage
"no-unused-variable": true
no-use-before-define => no-use-before-define (native)
Description: disallow use of variables before they are defined
Usage
"no-use-before-define": true
These rules are specific to JavaScript running on Node.js or using CommonJS in the browser.
callback-return => callback-return (tslint-eslint-rules) TODO
Description: enforce return
after a callback
Usage
"callback-return": [
true,
[
"callback",
"cb",
"next"
]
]
global-require => global-require (tslint-eslint-rules) TODO
Description: enforce require()
on top-level module scope
Usage
"global-require": true
handle-callback-err => handle-callback-err (tslint-eslint-rules) TODO
Description: enforce error handling in callbacks
Usage
"handle-callback-err": [
true,
"^(err|error|anySpecificError)$"
]
no-mixed-requires => no-mixed-requires (tslint-eslint-rules) TODO
Description: disallow mixing regular variable and require declarations
Usage
"no-mixed-requires": [
true,
{
"grouping": false
}
]
no-new-require => no-new-require (tslint-eslint-rules) TODO
Description: disallow use of new
operator with the require
function
Usage
"no-new-require": true
no-path-concat => no-path-concat (tslint-eslint-rules) TODO
Description: disallow string concatenation with __dirname
and __filename
Usage
"no-path-concat": true
no-process-exit => no-process-exit (tslint-eslint-rules) TODO
Description: disallow process.exit()
Usage
"no-process-exit": true
no-restricted-modules => no-restricted-modules (tslint-eslint-rules) TODO
Description: restrict Usage of specified node modules
Usage
"no-restricted-modules": [
true,
[
"fs",
"cluster",
"moduleName"
]
]
no-sync => no-sync (tslint-eslint-rules) TODO
Description: disallow use of synchronous methods
Usage
"no-sync": true
These rules are purely matters of style and are quite subjective.
array-bracket-spacing => array-bracket-spacing (tslint-eslint-rules) TODO
Description: enforce spacing inside array brackets
Usage
"array-bracket-spacing": [
true,
"always",
{
"singleValue": true,
"objectsInArrays": true,
"arraysInArrays": true
}
]
"array-bracket-spacing": [
true,
"never",
{
"singleValue": false,
"objectsInArrays": false,
"arraysInArrays": false
}
]
block-spacing => block-spacing (tslint-eslint-rules) TODO
Description: disallow or enforce spaces inside of single line blocks
Usage
"block-spacing": [
true,
"always"
]
"block-spacing": [
true,
"never"
]
brace-style => brace-style (tslint-eslint-rules) TODO
Description: enforce one true brace style
Usage
"brace-style": [
true,
"1tbs",
{
"allowSingleLine": true
}
]
"brace-style": [
true,
"stroustrup",
{
"allowSingleLine": true
}
]
"brace-style": [
true,
"allman",
{
"allowSingleLine": true
}
]
camelcase => variable-name (native)
Description: require camel case names
Usage
"variable-name": [
true,
"check-format"
]
comma-spacing => comma-spacing (tslint-eslint-rules) TODO
Description: enforce spacing before and after comma
Usage
"comma-spacing": [
true,
{
"before": false,
"after": true
}
]
comma-style => comma-style (tslint-eslint-rules) TODO
Description: enforce one true comma style
Usage
"comma-style": [
true,
"first"
]
"comma-style": [
true,
"last"
]
computed-property-spacing => computed-property-spacing (tslint-eslint-rules) TODO
Description: require or disallow padding inside computed properties
Usage
"computed-property-spacing": [
true,
"always"
]
"computed-property-spacing": [
true,
"never"
]
consistent-this => consistent-this (tslint-eslint-rules) TODO
Description: enforce consistent naming when capturing the current execution context
Usage
"consistent-this": [
true,
"self"
]
eol-last => eol-last (tslint-eslint-rules) TODO
Description: enforce newline at the end of file, with no multiple empty lines
Usage
"eol-last": [
true,
"unix"
]
"eol-last": [
true,
"windows"
]
func-names => func-names (tslint-eslint-rules) TODO
Description: require function expressions to have a name
Usage
"func-names": true
func-style => func-style (tslint-eslint-rules) TODO
Description: enforce use of function declarations or expressions
Usage
"func-style": [
true,
"declaration"
{
"allowArrowFunctions": true
}
]
"func-style": [
true,
"expression"
{
"allowArrowFunctions": true
}
]
id-length => id-length (tslint-eslint-rules) TODO
Description: this option enforces minimum and maximum identifier lengths (variable names, property names etc.)
Usage
"id-length": [
true,
{
"min": 2,
"max": 10,
"properties": "always",
"exceptions": [ "x", "bolinha" ]
}
]
"id-length": [
true,
{
"min": 2,
"max": 10,
"properties": "never",
"exceptions": [ "x", "bolinha" ]
}
]
id-match => id-match (tslint-eslint-rules) TODO
Description: require identifiers to match the provided regular expression
Usage
"id-match": [
true,
"^[a-z]+([A-Z][a-z]+)*$",
{
"properties": false
}
]
indent => indent (native)
Description: specify tab or space width for your code
Usage
"indent": [
true,
"spaces"
]
"indent": [
true,
"tabs"
]
jsx-quotes => jsx-quotes (tslint-eslint-rules) TODO
Description: specify whether double or single quotes should be used in JSX attributes
Usage
"jsx-quotes": [
true,
"prefer-double"
]
"jsx-quotes": [
true,
"prefer-single"
]
key-spacing => key-spacing (tslint-eslint-rules) TODO
Description: enforce spacing between keys and values in object literal properties
Usage
"key-spacing": [
true,
{
"align": "value",
"beforeColon": false,
"afterColon": true,
"mode": "minimum"
}
]
linebreak-style => linebreak-style (tslint-eslint-rules) TODO
Description: disallow mixed 'LF' and 'CRLF' as linebreaks
Usage
"linebreak-style": [
true,
"unix"
]
"linebreak-style": [
true,
"windows"
]
lines-around-comment => lines-around-comment (tslint-eslint-rules) TODO
Description: enforce empty lines around comments
Usage
"lines-around-comment": [
true,
{
"beforeBlockComment": true,
"afterBlockComment": false,
"beforeLineComment": false,
"afterLineComment": false,
"allowBlockStart": false,
"allowBlockEnd": false,
"allowObjectStart": false,
"allowObjectEnd": false,
"allowArrayStart": false,
"allowArrayEnd": false
}
]
max-nested-callbacks => max-nested-callbacks (tslint-eslint-rules) TODO
Description: specify the maximum depth callbacks can be nested
Usage
"max-nested-callbacks": [
true,
3
]
new-cap => Not applicable to TypeScript
new-parens => new-parens (tslint-eslint-rules) TODO
Description: disallow the omission of parentheses when invoking a constructor with no arguments
Usage
"new-parens": true
newline-after-var => newline-after-var (tslint-eslint-rules) TODO
Description: require or disallow an empty newline after variable declarations
Usage
"newline-after-var": [
true,
"never"
]
"newline-after-var": [
true,
"always"
]
no-array-constructor => no-array-constructor (tslint-eslint-rules) TODO
Description: disallow use of the Array
constructor
Usage
"no-array-constructor": true
no-continue => no-continue (tslint-eslint-rules) TODO
Description: disallow use of the continue
statement
Usage
"no-continue": true
no-inline-comments => no-inline-comments (tslint-eslint-rules) TODO
Description: disallow comments inline after code
Usage
"no-inline-comments": true
no-lonely-if => no-lonely-if (tslint-eslint-rules) TODO
Description: disallow if
as the only statement in an else
block
Usage
"no-lonely-if": true
no-mixed-spaces-and-tabs => ident (native)
Description: disallow mixed spaces and tabs for indentation (recommended)
Usage
"ident": "spaces"
"ident": "tabs"
Note: When using TSLint ident
rule, it will enforce the consistent use of the chosen identation. The ESLint rule allows an option for Smart Tabs, but there are some open issues, and we're not going to support this.
no-multiple-empty-lines => no-multiple-empty-lines (tslint-eslint-rules) TODO
Description: disallow multiple empty lines
Usage
"no-multiple-empty-lines": [
true,
{
"max": 2,
"maxEOF": 1
}
]
no-negated-condition => no-negated-condition (tslint-eslint-rules) TODO
Description: disallow negated conditions
Usage
"no-negated-condition": true
no-nested-ternary => no-nested-ternary (tslint-eslint-rules) TODO
Description: disallow nested ternary expressions
Usage
"no-nested-ternary": true
no-new-object => no-new-object (tslint-eslint-rules) TODO
Description: disallow the use of the Object
constructor
Usage
"no-new-object": true
no-restricted-syntax => no-restricted-syntax (tslint-eslint-rules) TODO
Description: disallow use of certain syntax in code
Usage
"no-restricted-syntax": [
true,
"FunctionExpression",
"WithStatement"
]
no-spaced-func => no-spaced-func (tslint-eslint-rules) TODO
Description: disallow space between function identifier and application
Usage
"no-spaced-func": true
no-ternary => no-ternary (tslint-eslint-rules) TODO
Description: disallow the use of ternary operators
Usage
"no-ternary": true
no-trailing-spaces => no-trailing-whitespace (native)
Description: disallow trailing whitespace at the end of lines
Usage
"no-trailing-whitespace": true
no-underscore-dangle => no-underscore-dangle (tslint-eslint-rules) TODO
Description: disallow dangling underscores in identifiers
Usage
"no-underscore-dangle": [
true,
{
"allow": ["foo_", "_bar"]
}
]
no-unneeded-ternary => no-unneeded-ternary (tslint-eslint-rules) TODO
Description: disallow the use of ternary operators when a simpler alternative exists
Usage
"no-unneeded-ternary": [
true,
{
"defaultAssignment": true
}
]
object-curly-spacing => object-curly-spacing (tslint-eslint-rules) TODO
Description: require or disallow padding inside curly braces
Usage
"object-curly-spacing": [
true,
"always"
]
"object-curly-spacing": [
true,
"never"
]
one-var => one-var (tslint-eslint-rules) TODO
Description: require or disallow one variable declaration per function
Usage
"one-var": [
true,
"always"
]
"one-var": [
true,
"never"
]
operator-assignment => operator-assignment (tslint-eslint-rules) TODO
Description: require assignment operator shorthand where possible or prohibit it entirely
Usage
"operator-assignment": [
true,
"always"
]
"operator-assignment": [
true,
"never"
]
operator-linebreak => operator-linebreak (tslint-eslint-rules) TODO
Description: enforce operators to be placed before or after line breaks
Usage
"operator-linebreak": [
true,
"before",
{
"overrides": { "?": "after"}
}
]
"operator-linebreak": [
true,
"after",
{
"overrides": { "?": "after"}
}
]
"operator-linebreak": [
true,
"none",
{
"overrides": { "?": "none", "+=": "none"}
}
]
padded-blocks => padded-blocks (tslint-eslint-rules) TODO
Description: enforce padding within blocks
Usage
"padded-blocks": [
true,
"always"
]
"padded-blocks": [
true,
"never"
]
quote-props => quote-props (tslint-eslint-rules) TODO
Description: require quotes around object literal property names
Usage
"quote-props": [
true,
"always"
]
"quote-props": [
true,
"as-needed"
]
"quote-props": [
true,
"consistent"
]
"quote-props": [
true,
"consistent-as-needed"
]
quotes => quote-props (tslint-eslint-rules) TODO
Description: specify whether backticks, double or single quotes should be used
Usage
"quotes": [
true,
"single"
]
"quotes": [
true,
"single",
"avoid-escape"
]
"quotes": [
true,
"double"
]
"quotes": [
true,
"double",
"avoid-escape"
]
"quotes": [
true,
"backtick"
]
"quotes": [
true,
"backtick",
"avoid-escape"
]
require-jsdoc => require-jsdoc (tslint-eslint-rules) TODO
Description: Require JSDoc comment
Usage
"require-jsdoc": [
true,
{
"require":
{
"FunctionDeclaration": true,
"MethodDefinition": false,
"ClassDeclaration": false
}
}
]
semi-spacing => semi-spacing (tslint-eslint-rules) TODO
Description: enforce spacing before and after semicolons
Usage
"semi-spacing": [
true,
{
"before": false,
"after": true
}
]
semi => semi (tslint-eslint-rules) TODO
Description: require or disallow use of semicolons instead of ASI
Usage
"semi": [
true,
"always"
]
"semi": [
true,
"never"
]
sort-vars => sort-vars (tslint-eslint-rules) TODO
Description: sort variables within the same declaration block
Usage
"sort-vars": [
true,
{
"ignoreCase": false
}
]
space-after-keywords => space-after-keywords (tslint-eslint-rules) TODO
Description: require a space after certain keywords
Usage
"space-after-keywords": [
true,
"always"
]
"space-after-keywords": [
true,
"never"
]
space-before-blocks => space-before-blocks (tslint-eslint-rules) TODO
Description: require or disallow a space before blocks
Usage
"space-before-blocks": [
true,
"always"
]
"space-before-blocks": [
true,
"never"
]
"space-before-blocks": [
true,
{
"functions": "never",
"keywords": "always"
}
]
space-before-function-paren => space-before-function-paren (tslint-eslint-rules) TODO
Description: require or disallow a space before function opening parenthesis
Usage
"space-before-function-paren": [
true,
"always"
]
"space-before-function-paren": [
true,
"never"
]
"space-before-function-paren": [
true,
{
"anonymous": "always",
"named": "never"
}
]
space-before-keywords => space-before-keywords (tslint-eslint-rules) TODO
Description: require a space before certain keywords
Usage
"space-before-keywords": [
true,
"always"
]
"space-before-keywords": [
true,
"never"
]
space-in-parens => space-in-parens (tslint-eslint-rules) TODO
Description: require or disallow spaces inside parentheses
Usage
"space-in-parens": [
true,
"always"
]
"space-in-parens": [
true,
"never"
]
space-infix-ops => space-infix-ops (tslint-eslint-rules) TODO
Description: require spaces around operators
Usage
"space-infix-ops": [
true,
{
"int32Hint": false
}
]
space-return-throw-case => space-return-throw-case (tslint-eslint-rules) TODO
Description: require a space after return
, throw
, and case
Usage
"space-return-throw-case": true
space-unary-ops => space-unary-ops (tslint-eslint-rules) TODO
Description: require or disallow spaces before/after unary operators
Usage
"space-unary-ops": [
true,
{
"words": true,
"nonwords": false
}
]
spaced-comment => spaced-comment (tslint-eslint-rules) TODO
Description: require or disallow a space immediately following the //
or /*
in a comment
Usage
"spaced-comment": [
true,
"always"
]
"spaced-comment": [
true,
"never"
]
"spaced-comment": [
true,
"always",
{
"exceptions": ["-", "+"]
}
]
"spaced-comment": [
true,
"always",
{
"line": {
"markers": ["/"]
"exceptions": ["-", "+"]
},
"block": {
"markers": ["/"]
"exceptions": ["-", "+"]
}
}
]
wrap-regex => wrap-regex (tslint-eslint-rules) TODO
Description: require regex literals to be wrapped in parentheses
Usage
"wrap-regex": true
These rules are only relevant to ES6 environments.
arrow-body-style => arrow-body-style (tslint-eslint-rules) TODO
Description: require braces in arrow function body
Usage
"arrow-body-style": [
true,
"as-needed"
]
"arrow-body-style": [
true,
"always"
]
arrow-parens => arrow-parens (tslint-eslint-rules) TODO
Description: require parens in arrow function arguments
Usage
"arrow-parens": [
true,
"as-needed"
]
"arrow-parens": [
true,
"always"
]
arrow-spacing => arrow-spacing (tslint-eslint-rules) TODO
Description: require space before/after arrow function's arrow
Usage
"arrow-spacing": [
true,
{
"before": true,
"after": true
}
]
constructor-super => constructor-super (tslint-eslint-rules) TODO
Description: verify calls of super()
in constructors
Usage
"constructor-super": true
generator-star-spacing => generator-star-spacing (tslint-eslint-rules) TODO
Description: enforce spacing around the *
in generator functions
Usage
"generator-star-spacing": [
true,
{
"before": true,
"after": true
}
]
no-arrow-condition => no-arrow-condition (tslint-eslint-rules) TODO
Description: disallow arrow functions where a condition is expected
Usage
"no-arrow-condition": true
no-class-assign => no-class-assign (tslint-eslint-rules) TODO
Description: disallow modifying variables of class declarations
Usage
"no-class-assign": true
no-const-assign => no-const-assign (tslint-eslint-rules) TODO
Description: disallow modifying variables that are declared using const
Usage
"no-const-assign": true
no-dupe-class-members => Not applicable to TypeScript
no-this-before-super => no-this-before-super (tslint-eslint-rules) TODO
Description: disallow use of this
/super
before calling super()
in constructors.
Usage
"no-this-before-super": true
no-var => no-var-keyword (native)
Description: require let
or const
instead of var
Usage
"no-var-keyword": true
object-shorthand => object-shorthand (tslint-eslint-rules) TODO
Description: require method and property shorthand syntax for object literals
Usage
"object-shorthand": [
true,
"always"
]
"object-shorthand": [
true,
"methods"
]
"object-shorthand": [
true,
"properties"
]
"object-shorthand": [
true,
"never"
]
prefer-arrow-callback => prefer-arrow-callback (tslint-eslint-rules) TODO
Description: suggest using arrow functions as callbacks
Usage
"prefer-arrow-callback": true
prefer-const => prefer-const (tslint-eslint-rules) TODO
Description: suggest using const
declaration for variables that are never modified after declared
Usage
"prefer-const": true
prefer-reflect => prefer-reflect (tslint-eslint-rules) TODO
Description: suggest using Reflect methods where applicable
Usage
"prefer-reflect": [
true,
{
"exceptions": ["apply", "call", "defineProperty", "getOwnPropertyDescriptor", "getPrototypeOf", "setPrototypeOf", "isExtensible", "getOwnPropertyNames", "preventExtensions", "delete"]
}
]
prefer-spread => prefer-spread (tslint-eslint-rules) TODO
Description: suggest using the spread operator instead of .apply()
.
Usage
"prefer-spread": true
prefer-template => prefer-template (tslint-eslint-rules) TODO
Description: suggest using template literals instead of strings concatenation
Usage
"prefer-template": true
require-yield => require-yield (tslint-eslint-rules) TODO
Description: disallow generator functions that do not have yield
Usage
"require-yield": true
Bugs, rules requests, doubts etc., open a Github Issue.
If you didn't find the rule, you can also create an ESLint custom rule for TSLint:
npm install
gulp
to run the tests and watch for file changes./src/test/rules
and your rule in ./src/rules
with the convetion:
Rule
suffix, e.g: noIfUsageRule.ts)RuleTests
suffix, e.g: noIfUsageRuleTests.ts)[feat] added use-isnan rule (closes #20)
You can also contribute with PRs for fixing bugs, or improving documentation, performance. The commit convention for these are, respectively:
[bug] fixed no-constant-condition rule (closes #9)
[docs] improved README.md file (closes #32)
[perf] improved valid-typeof rule (closes #48)
MIT
[v1.0.0] - 2015/12/14 17:14 +00:00
FAQs
Improve your TSLint with the missing ESLint Rules
The npm package tslint-eslint-rules receives a total of 291,751 weekly downloads. As such, tslint-eslint-rules popularity was classified as popular.
We found that tslint-eslint-rules demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 2 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Django has updated its security policies to reject AI-generated vulnerability reports that include fabricated or unverifiable content.
Security News
ECMAScript 2025 introduces Iterator Helpers, Set methods, JSON modules, and more in its latest spec update approved by Ecma in June 2025.
Security News
A new Node.js homepage button linking to paid support for EOL versions has sparked a heated discussion among contributors and the wider community.