tsparticles-preset-fireworks
Advanced tools
Comparing version 2.0.6 to 2.1.0
@@ -0,1 +1,83 @@ | ||
import { rgbToHsl, setRangeValue, stringToRgb, } from "tsparticles-engine"; | ||
const fixRange = (value, min, max) => { | ||
const diffSMax = value.max > max ? value.max - max : 0; | ||
let res = setRangeValue(value); | ||
if (diffSMax) { | ||
res = setRangeValue(value.min - diffSMax, max); | ||
} | ||
const diffSMin = value.min < min ? value.min : 0; | ||
if (diffSMin) { | ||
res = setRangeValue(0, value.max + diffSMin); | ||
} | ||
return res; | ||
}; | ||
const fireworksOptions = ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"] | ||
.map((color) => { | ||
const rgb = stringToRgb(color); | ||
if (!rgb) { | ||
return undefined; | ||
} | ||
const hsl = rgbToHsl(rgb), sRange = fixRange({ min: hsl.s - 20, max: hsl.s + 20 }, 0, 100), lRange = fixRange({ min: hsl.l - 20, max: hsl.l + 20 }, 0, 100); | ||
return { | ||
color: { | ||
value: { | ||
h: hsl.h, | ||
s: sRange, | ||
l: lRange, | ||
}, | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max" /* StartValueType.max */, | ||
destroy: "min" /* DestroyType.min */, | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy" /* OutMode.destroy */, | ||
}, | ||
}; | ||
}) | ||
.filter((t) => t !== undefined); | ||
export const options = { | ||
@@ -8,3 +90,3 @@ detectRetina: true, | ||
emitters: { | ||
direction: "top" /* top */, | ||
direction: "top" /* MoveDirection.top */, | ||
life: { | ||
@@ -16,3 +98,3 @@ count: 0, | ||
rate: { | ||
delay: 0.5, | ||
delay: 0.25, | ||
quantity: 1, | ||
@@ -34,3 +116,3 @@ }, | ||
destroy: { | ||
mode: "split" /* split */, | ||
mode: "split" /* DestroyMode.split */, | ||
split: { | ||
@@ -42,60 +124,5 @@ count: 1, | ||
rate: { | ||
value: 100, | ||
value: 200, | ||
}, | ||
particles: { | ||
color: { | ||
value: ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"], | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max" /* max */, | ||
destroy: "min" /* min */, | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy" /* destroy */, | ||
}, | ||
}, | ||
particles: fireworksOptions, | ||
}, | ||
@@ -118,4 +145,4 @@ }, | ||
speed: 90, | ||
startValue: "max" /* max */, | ||
destroy: "min" /* min */, | ||
startValue: "max" /* StartValueType.max */, | ||
destroy: "min" /* DestroyType.min */, | ||
}, | ||
@@ -145,4 +172,4 @@ }, | ||
outModes: { | ||
default: "destroy" /* destroy */, | ||
top: "none" /* none */, | ||
default: "destroy" /* OutMode.destroy */, | ||
top: "none" /* OutMode.none */, | ||
}, | ||
@@ -149,0 +176,0 @@ trail: { |
"use strict"; | ||
Object.defineProperty(exports, "__esModule", { value: true }); | ||
exports.options = void 0; | ||
const tsparticles_engine_1 = require("tsparticles-engine"); | ||
const fixRange = (value, min, max) => { | ||
const diffSMax = value.max > max ? value.max - max : 0; | ||
let res = (0, tsparticles_engine_1.setRangeValue)(value); | ||
if (diffSMax) { | ||
res = (0, tsparticles_engine_1.setRangeValue)(value.min - diffSMax, max); | ||
} | ||
const diffSMin = value.min < min ? value.min : 0; | ||
if (diffSMin) { | ||
res = (0, tsparticles_engine_1.setRangeValue)(0, value.max + diffSMin); | ||
} | ||
return res; | ||
}; | ||
const fireworksOptions = ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"] | ||
.map((color) => { | ||
const rgb = (0, tsparticles_engine_1.stringToRgb)(color); | ||
if (!rgb) { | ||
return undefined; | ||
} | ||
const hsl = (0, tsparticles_engine_1.rgbToHsl)(rgb), sRange = fixRange({ min: hsl.s - 20, max: hsl.s + 20 }, 0, 100), lRange = fixRange({ min: hsl.l - 20, max: hsl.l + 20 }, 0, 100); | ||
return { | ||
color: { | ||
value: { | ||
h: hsl.h, | ||
s: sRange, | ||
l: lRange, | ||
}, | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}; | ||
}) | ||
.filter((t) => t !== undefined); | ||
exports.options = { | ||
@@ -18,3 +100,3 @@ detectRetina: true, | ||
rate: { | ||
delay: 0.5, | ||
delay: 0.25, | ||
quantity: 1, | ||
@@ -43,60 +125,5 @@ }, | ||
rate: { | ||
value: 100, | ||
value: 200, | ||
}, | ||
particles: { | ||
color: { | ||
value: ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"], | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}, | ||
particles: fireworksOptions, | ||
}, | ||
@@ -103,0 +130,0 @@ }, |
@@ -0,1 +1,83 @@ | ||
import { rgbToHsl, setRangeValue, stringToRgb, } from "tsparticles-engine"; | ||
const fixRange = (value, min, max) => { | ||
const diffSMax = value.max > max ? value.max - max : 0; | ||
let res = setRangeValue(value); | ||
if (diffSMax) { | ||
res = setRangeValue(value.min - diffSMax, max); | ||
} | ||
const diffSMin = value.min < min ? value.min : 0; | ||
if (diffSMin) { | ||
res = setRangeValue(0, value.max + diffSMin); | ||
} | ||
return res; | ||
}; | ||
const fireworksOptions = ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"] | ||
.map((color) => { | ||
const rgb = stringToRgb(color); | ||
if (!rgb) { | ||
return undefined; | ||
} | ||
const hsl = rgbToHsl(rgb), sRange = fixRange({ min: hsl.s - 20, max: hsl.s + 20 }, 0, 100), lRange = fixRange({ min: hsl.l - 20, max: hsl.l + 20 }, 0, 100); | ||
return { | ||
color: { | ||
value: { | ||
h: hsl.h, | ||
s: sRange, | ||
l: lRange, | ||
}, | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}; | ||
}) | ||
.filter((t) => t !== undefined); | ||
export const options = { | ||
@@ -15,3 +97,3 @@ detectRetina: true, | ||
rate: { | ||
delay: 0.5, | ||
delay: 0.25, | ||
quantity: 1, | ||
@@ -40,60 +122,5 @@ }, | ||
rate: { | ||
value: 100, | ||
value: 200, | ||
}, | ||
particles: { | ||
color: { | ||
value: ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"], | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}, | ||
particles: fireworksOptions, | ||
}, | ||
@@ -100,0 +127,0 @@ }, |
{ | ||
"name": "tsparticles-preset-fireworks", | ||
"version": "2.0.6", | ||
"version": "2.1.0", | ||
"description": "tsParticles fireworks preset", | ||
@@ -84,15 +84,15 @@ "homepage": "https://particles.js.org/", | ||
"dependencies": { | ||
"tsparticles-engine": "^2.0.6", | ||
"tsparticles-move-base": "^2.0.6", | ||
"tsparticles-plugin-emitters": "^2.0.6", | ||
"tsparticles-shape-circle": "^2.0.6", | ||
"tsparticles-shape-line": "^2.0.6", | ||
"tsparticles-updater-angle": "^2.0.6", | ||
"tsparticles-updater-color": "^2.0.6", | ||
"tsparticles-updater-life": "^2.0.6", | ||
"tsparticles-updater-opacity": "^2.0.6", | ||
"tsparticles-updater-out-modes": "^2.0.6", | ||
"tsparticles-updater-size": "^2.0.6", | ||
"tsparticles-updater-stroke-color": "^2.0.6" | ||
"tsparticles-engine": "^2.1.0", | ||
"tsparticles-move-base": "^2.1.0", | ||
"tsparticles-plugin-emitters": "^2.1.0", | ||
"tsparticles-shape-circle": "^2.1.0", | ||
"tsparticles-shape-line": "^2.1.0", | ||
"tsparticles-updater-angle": "^2.1.0", | ||
"tsparticles-updater-color": "^2.1.0", | ||
"tsparticles-updater-life": "^2.1.0", | ||
"tsparticles-updater-opacity": "^2.1.0", | ||
"tsparticles-updater-out-modes": "^2.1.0", | ||
"tsparticles-updater-size": "^2.1.0", | ||
"tsparticles-updater-stroke-color": "^2.1.0" | ||
} | ||
} |
@@ -28,4 +28,15 @@ [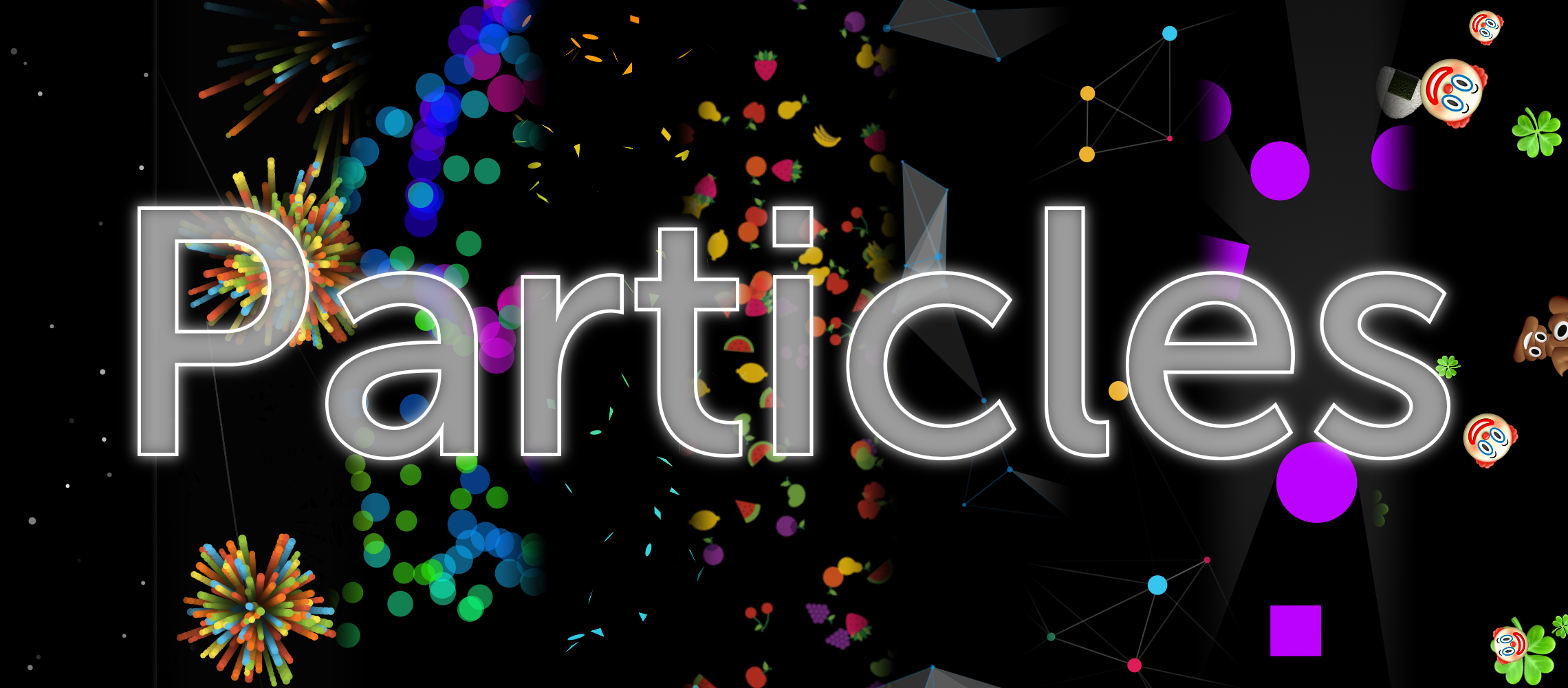](https://particles.js.org) | ||
```html | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles@1/tsparticles.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-preset-fireworks@1/tsparticles.preset.fireworks.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-engine@2/tsparticles.engine.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-move-base@2/tsparticles.move.base.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-plugin-emitters@2/tsparticles.plugin.emitters.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-shape-circle@2/tsparticles.shape.circle.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-shape-line@2/tsparticles.shape.line.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-angle@2/tsparticles.updater.angle.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-color@2/tsparticles.updater.color.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-life@2/tsparticles.updater.life.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-opacity@2/tsparticles.updater.opacity.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-out-modes@2/tsparticles.updater.out-modes.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-size@2/tsparticles.updater.size.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-updater-stroke-color@2/tsparticles.updater.stroke-color.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-preset-fireworks@2/tsparticles.preset.fireworks.min.js"></script> | ||
``` | ||
@@ -40,3 +51,3 @@ | ||
```html | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-preset-fireworks@1/tsparticles.preset.fireworks.bundle.min.js"></script> | ||
<script src="https://cdn.jsdelivr.net/npm/tsparticles-preset-fireworks@2/tsparticles.preset.fireworks.bundle.min.js"></script> | ||
``` | ||
@@ -49,7 +60,9 @@ | ||
```javascript | ||
loadFireworksPreset(tsParticles); | ||
(async () => { | ||
await loadFireworksPreset(tsParticles); // this is required only if you are not using the bundle script | ||
tsParticles.load("tsparticles", { | ||
preset: "fireworks", | ||
}); | ||
await tsParticles.load("tsparticles", { | ||
preset: "fireworks", | ||
}); | ||
})(); | ||
``` | ||
@@ -66,3 +79,3 @@ | ||
shape: { | ||
type: "square", | ||
type: "square", // starting from v2, this require the square shape script | ||
}, | ||
@@ -109,6 +122,10 @@ }, | ||
```vue | ||
<Particles id="tsparticles" :particlesInit="particlesInit" url="http://foo.bar/particles.json" /> | ||
<Particles id="tsparticles" :particlesInit="particlesInit" :options="particlesOptions" /> | ||
``` | ||
```js | ||
```ts | ||
const particlesOptions = { | ||
preset: "fireworks", | ||
}; | ||
async function particlesInit(engine: Engine): Promise<void> { | ||
@@ -122,11 +139,10 @@ await loadFireworksPreset(engine); | ||
```html | ||
<ng-particles | ||
[id]="id" | ||
[options]="particlesOptions" | ||
(particlesLoaded)="particlesLoaded($event)" | ||
(particlesInit)="particlesInit($event)" | ||
></ng-particles> | ||
<ng-particles [id]="id" [options]="particlesOptions" [particlesInit]="particlesInit"></ng-particles> | ||
``` | ||
```ts | ||
const particlesOptions = { | ||
preset: "fireworks", | ||
}; | ||
async function particlesInit(engine: Engine): Promise<void> { | ||
@@ -143,4 +159,4 @@ await loadFireworksPreset(engine); | ||
id="tsparticles" | ||
url="http://foo.bar/particles.json" | ||
on:particlesInit="{onParticlesInit}" | ||
options={particlesOptions} | ||
particlesInit={particlesInit} | ||
/> | ||
@@ -150,6 +166,9 @@ ``` | ||
```js | ||
let onParticlesInit = (event) => { | ||
const main = event.detail; | ||
loadFireworksPreset(main); | ||
let particlesOptions = { | ||
preset: "fireworks", | ||
}; | ||
let particlesInit = async (engine) => { | ||
await loadFireworksPreset(engine); | ||
}; | ||
``` |
@@ -7,3 +7,3 @@ /*! | ||
* How to use? : Check the GitHub README | ||
* v2.0.6 | ||
* v2.1.0 | ||
*/ | ||
@@ -194,3 +194,3 @@ (function webpackUniversalModuleDefinition(root, factory) { | ||
function updateColorValue(delta, value, valueAnimation, max, decrease) { | ||
var _a; | ||
var _a, _b; | ||
@@ -203,4 +203,5 @@ const colorValue = value; | ||
const offset = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.randomInRange)(valueAnimation.offset); | ||
const velocity = ((_a = value.velocity) !== null && _a !== void 0 ? _a : 0) * delta.factor + offset * 3.6; | ||
const offset = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.randomInRange)(valueAnimation.offset), | ||
velocity = ((_a = value.velocity) !== null && _a !== void 0 ? _a : 0) * delta.factor + offset * 3.6, | ||
decay = (_b = value.decay) !== null && _b !== void 0 ? _b : 1; | ||
@@ -223,2 +224,6 @@ if (!decrease || colorValue.status === 0) { | ||
if (colorValue.velocity && decay !== 1) { | ||
colorValue.velocity *= decay; | ||
} | ||
if (colorValue.value > max) { | ||
@@ -236,4 +241,4 @@ colorValue.value %= max; | ||
const animationOptions = particle.stroke.color.animation; | ||
const h = (_c = (_b = particle.strokeColor) === null || _b === void 0 ? void 0 : _b.h) !== null && _c !== void 0 ? _c : (_d = particle.color) === null || _d === void 0 ? void 0 : _d.h; | ||
const animationOptions = particle.stroke.color.animation, | ||
h = (_c = (_b = particle.strokeColor) === null || _b === void 0 ? void 0 : _b.h) !== null && _c !== void 0 ? _c : (_d = particle.color) === null || _d === void 0 ? void 0 : _d.h; | ||
@@ -268,3 +273,3 @@ if (h) { | ||
particle.strokeWidth = particle.stroke.width * container.retina.pixelRatio; | ||
const strokeHslColor = (_a = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.colorToHsl)(particle.stroke.color)) !== null && _a !== void 0 ? _a : particle.getFillColor(); | ||
const strokeHslColor = (_a = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.rangeColorToHsl)(particle.stroke.color)) !== null && _a !== void 0 ? _a : particle.getFillColor(); | ||
@@ -298,2 +303,104 @@ if (strokeHslColor) { | ||
;// CONCATENATED MODULE: ./dist/browser/options.js | ||
const fixRange = (value, min, max) => { | ||
const diffSMax = value.max > max ? value.max - max : 0; | ||
let res = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.setRangeValue)(value); | ||
if (diffSMax) { | ||
res = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.setRangeValue)(value.min - diffSMax, max); | ||
} | ||
const diffSMin = value.min < min ? value.min : 0; | ||
if (diffSMin) { | ||
res = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.setRangeValue)(0, value.max + diffSMin); | ||
} | ||
return res; | ||
}; | ||
const fireworksOptions = ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"].map(color => { | ||
const rgb = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.stringToRgb)(color); | ||
if (!rgb) { | ||
return undefined; | ||
} | ||
const hsl = (0,external_commonjs_tsparticles_engine_commonjs2_tsparticles_engine_amd_tsparticles_engine_root_window_.rgbToHsl)(rgb), | ||
sRange = fixRange({ | ||
min: hsl.s - 20, | ||
max: hsl.s + 20 | ||
}, 0, 100), | ||
lRange = fixRange({ | ||
min: hsl.l - 20, | ||
max: hsl.l + 20 | ||
}, 0, 100); | ||
return { | ||
color: { | ||
value: { | ||
h: hsl.h, | ||
s: sRange, | ||
l: lRange | ||
} | ||
}, | ||
stroke: { | ||
width: 0 | ||
}, | ||
number: { | ||
value: 0 | ||
}, | ||
collisions: { | ||
enable: false | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1 | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max" | ||
/* StartValueType.max */ | ||
, | ||
destroy: "min" | ||
/* DestroyType.min */ | ||
} | ||
}, | ||
shape: { | ||
type: "circle" | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false | ||
} | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2 | ||
} | ||
} | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy" | ||
/* OutMode.destroy */ | ||
} | ||
}; | ||
}).filter(t => t !== undefined); | ||
const options = { | ||
@@ -307,3 +414,3 @@ detectRetina: true, | ||
direction: "top" | ||
/* top */ | ||
/* MoveDirection.top */ | ||
, | ||
@@ -316,3 +423,3 @@ life: { | ||
rate: { | ||
delay: 0.5, | ||
delay: 0.25, | ||
quantity: 1 | ||
@@ -335,3 +442,3 @@ }, | ||
mode: "split" | ||
/* split */ | ||
/* DestroyMode.split */ | ||
, | ||
@@ -344,66 +451,5 @@ split: { | ||
rate: { | ||
value: 100 | ||
value: 200 | ||
}, | ||
particles: { | ||
color: { | ||
value: ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"] | ||
}, | ||
stroke: { | ||
width: 0 | ||
}, | ||
number: { | ||
value: 0 | ||
}, | ||
collisions: { | ||
enable: false | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1 | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max" | ||
/* max */ | ||
, | ||
destroy: "min" | ||
/* min */ | ||
} | ||
}, | ||
shape: { | ||
type: "circle" | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false | ||
} | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2 | ||
} | ||
} | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy" | ||
/* destroy */ | ||
} | ||
} | ||
particles: fireworksOptions | ||
} | ||
@@ -427,6 +473,6 @@ }, | ||
startValue: "max" | ||
/* max */ | ||
/* StartValueType.max */ | ||
, | ||
destroy: "min" | ||
/* min */ | ||
/* DestroyType.min */ | ||
@@ -458,6 +504,6 @@ } | ||
default: "destroy" | ||
/* destroy */ | ||
/* OutMode.destroy */ | ||
, | ||
top: "none" | ||
/* none */ | ||
/* OutMode.none */ | ||
@@ -464,0 +510,0 @@ }, |
@@ -1,2 +0,2 @@ | ||
/*! tsParticles Fireworks Preset v2.0.6 by Matteo Bruni */ | ||
!function(e,t){if("object"==typeof exports&&"object"==typeof module)module.exports=t(require("tsparticles-updater-angle"),require("tsparticles-move-base"),require("tsparticles-shape-circle"),require("tsparticles-updater-color"),require("tsparticles-plugin-emitters"),require("tsparticles-updater-life"),require("tsparticles-shape-line"),require("tsparticles-updater-opacity"),require("tsparticles-updater-out-modes"),require("tsparticles-updater-size"),require("tsparticles-engine"));else if("function"==typeof define&&define.amd)define(["tsparticles-updater-angle","tsparticles-move-base","tsparticles-shape-circle","tsparticles-updater-color","tsparticles-plugin-emitters","tsparticles-updater-life","tsparticles-shape-line","tsparticles-updater-opacity","tsparticles-updater-out-modes","tsparticles-updater-size","tsparticles-engine"],t);else{var o="object"==typeof exports?t(require("tsparticles-updater-angle"),require("tsparticles-move-base"),require("tsparticles-shape-circle"),require("tsparticles-updater-color"),require("tsparticles-plugin-emitters"),require("tsparticles-updater-life"),require("tsparticles-shape-line"),require("tsparticles-updater-opacity"),require("tsparticles-updater-out-modes"),require("tsparticles-updater-size"),require("tsparticles-engine")):t(e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window);for(var r in o)("object"==typeof exports?exports:e)[r]=o[r]}}(this,(function(e,t,o,r,i,a,n,s,l,u,c){return function(){"use strict";var d={818:function(e){e.exports=c},919:function(e){e.exports=t},949:function(e){e.exports=i},941:function(e){e.exports=o},45:function(e){e.exports=n},1:function(t){t.exports=e},841:function(e){e.exports=r},489:function(e){e.exports=a},838:function(e){e.exports=s},364:function(e){e.exports=l},328:function(e){e.exports=u}},p={};function v(e){var t=p[e];if(void 0!==t)return t.exports;var o=p[e]={exports:{}};return d[e](o,o.exports,v),o.exports}v.d=function(e,t){for(var o in t)v.o(t,o)&&!v.o(e,o)&&Object.defineProperty(e,o,{enumerable:!0,get:t[o]})},v.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},v.r=function(e){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})};var f={};return function(){v.r(f),v.d(f,{loadFireworksPreset:function(){return w}});var e=v(1),t=v(919),o=v(941),r=v(841),i=v(949),a=v(489),n=v(45),s=v(838),l=v(364),u=v(328),c=v(818);function d(e,t,o,r,i){var a;const n=t;if(!n||!n.enable)return;const s=(0,c.randomInRange)(o.offset),l=(null!==(a=t.velocity)&&void 0!==a?a:0)*e.factor+3.6*s;i&&0!==n.status?(n.value-=l,n.value<0&&(n.status=0,n.value+=n.value)):(n.value+=l,i&&n.value>r&&(n.status=1,n.value-=n.value%r)),n.value>r&&(n.value%=r)}class p{constructor(e){this.container=e}init(e){var t,o;const r=this.container;e.stroke=e.options.stroke instanceof Array?(0,c.itemFromArray)(e.options.stroke,e.id,e.options.reduceDuplicates):e.options.stroke,e.strokeWidth=e.stroke.width*r.retina.pixelRatio;const i=null!==(t=(0,c.colorToHsl)(e.stroke.color))&&void 0!==t?t:e.getFillColor();i&&(e.strokeColor=(0,c.getHslAnimationFromHsl)(i,null===(o=e.stroke.color)||void 0===o?void 0:o.animation,r.retina.reduceFactor))}isEnabled(e){var t,o,r,i;const a=null===(t=e.stroke)||void 0===t?void 0:t.color;return!e.destroyed&&!e.spawning&&!!a&&(void 0!==(null===(o=e.strokeColor)||void 0===o?void 0:o.h.value)&&a.animation.h.enable||void 0!==(null===(r=e.strokeColor)||void 0===r?void 0:r.s.value)&&a.animation.s.enable||void 0!==(null===(i=e.strokeColor)||void 0===i?void 0:i.l.value)&&a.animation.l.enable)}update(e,t){this.isEnabled(e)&&function(e,t){var o,r,i,a,n,s,l,u,c,p;if(!(null===(o=e.stroke)||void 0===o?void 0:o.color))return;const v=e.stroke.color.animation,f=null!==(i=null===(r=e.strokeColor)||void 0===r?void 0:r.h)&&void 0!==i?i:null===(a=e.color)||void 0===a?void 0:a.h;f&&d(t,f,v.h,360,!1);const m=null!==(s=null===(n=e.strokeColor)||void 0===n?void 0:n.s)&&void 0!==s?s:null===(l=e.color)||void 0===l?void 0:l.s;m&&d(t,m,v.s,100,!0);const w=null!==(c=null===(u=e.strokeColor)||void 0===u?void 0:u.l)&&void 0!==c?c:null===(p=e.color)||void 0===p?void 0:p.l;w&&d(t,w,v.l,100,!0)}(e,t)}}const m={detectRetina:!0,background:{color:"#000"},fpsLimit:120,emitters:{direction:"top",life:{count:0,duration:.1,delay:.1},rate:{delay:.5,quantity:1},size:{width:100,height:0},position:{y:100,x:50}},particles:{number:{value:0},destroy:{mode:"split",split:{count:1,factor:{value:.333333},rate:{value:100},particles:{color:{value:["#ff595e","#ffca3a","#8ac926","#1982c4","#6a4c93"]},stroke:{width:0},number:{value:0},collisions:{enable:!1},opacity:{value:{min:.1,max:1},animation:{enable:!0,speed:.7,sync:!1,startValue:"max",destroy:"min"}},shape:{type:"circle"},size:{value:2,animation:{enable:!1}},life:{count:1,duration:{value:{min:1,max:2}}},move:{enable:!0,gravity:{enable:!1},speed:2,direction:"none",random:!0,straight:!1,outModes:"destroy"}}}},life:{count:1},shape:{type:"line"},size:{value:{min:.1,max:50},animation:{enable:!0,sync:!0,speed:90,startValue:"max",destroy:"min"}},stroke:{color:{value:"#ffffff"},width:1},rotate:{path:!0},move:{enable:!0,gravity:{acceleration:15,enable:!0,inverse:!0,maxSpeed:100},speed:{min:10,max:20},outModes:{default:"destroy",top:"none"},trail:{fillColor:"#000",enable:!0,length:10}}}};async function w(c){await(0,t.loadBaseMover)(c),await(0,i.loadEmittersPlugin)(c),await(0,o.loadCircleShape)(c),await(0,n.loadLineShape)(c),await(0,e.loadAngleUpdater)(c),await(0,r.loadColorUpdater)(c),await(0,a.loadLifeUpdater)(c),await(0,s.loadOpacityUpdater)(c),await(0,l.loadOutModesUpdater)(c),await(0,u.loadSizeUpdater)(c),await async function(e){await e.addParticleUpdater("strokeColor",(e=>new p(e)))}(c),await c.addPreset("fireworks",m)}}(),f}()})); | ||
/*! tsParticles Fireworks Preset v2.1.0 by Matteo Bruni */ | ||
!function(e,t){if("object"==typeof exports&&"object"==typeof module)module.exports=t(require("tsparticles-updater-angle"),require("tsparticles-move-base"),require("tsparticles-shape-circle"),require("tsparticles-updater-color"),require("tsparticles-plugin-emitters"),require("tsparticles-updater-life"),require("tsparticles-shape-line"),require("tsparticles-updater-opacity"),require("tsparticles-updater-out-modes"),require("tsparticles-updater-size"),require("tsparticles-engine"));else if("function"==typeof define&&define.amd)define(["tsparticles-updater-angle","tsparticles-move-base","tsparticles-shape-circle","tsparticles-updater-color","tsparticles-plugin-emitters","tsparticles-updater-life","tsparticles-shape-line","tsparticles-updater-opacity","tsparticles-updater-out-modes","tsparticles-updater-size","tsparticles-engine"],t);else{var o="object"==typeof exports?t(require("tsparticles-updater-angle"),require("tsparticles-move-base"),require("tsparticles-shape-circle"),require("tsparticles-updater-color"),require("tsparticles-plugin-emitters"),require("tsparticles-updater-life"),require("tsparticles-shape-line"),require("tsparticles-updater-opacity"),require("tsparticles-updater-out-modes"),require("tsparticles-updater-size"),require("tsparticles-engine")):t(e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window,e.window);for(var i in o)("object"==typeof exports?exports:e)[i]=o[i]}}(this,(function(e,t,o,i,r,a,n,s,l,u,c){return function(){"use strict";var d={818:function(e){e.exports=c},919:function(e){e.exports=t},949:function(e){e.exports=r},941:function(e){e.exports=o},45:function(e){e.exports=n},1:function(t){t.exports=e},841:function(e){e.exports=i},489:function(e){e.exports=a},838:function(e){e.exports=s},364:function(e){e.exports=l},328:function(e){e.exports=u}},p={};function v(e){var t=p[e];if(void 0!==t)return t.exports;var o=p[e]={exports:{}};return d[e](o,o.exports,v),o.exports}v.d=function(e,t){for(var o in t)v.o(t,o)&&!v.o(e,o)&&Object.defineProperty(e,o,{enumerable:!0,get:t[o]})},v.o=function(e,t){return Object.prototype.hasOwnProperty.call(e,t)},v.r=function(e){"undefined"!=typeof Symbol&&Symbol.toStringTag&&Object.defineProperty(e,Symbol.toStringTag,{value:"Module"}),Object.defineProperty(e,"__esModule",{value:!0})};var f={};return function(){v.r(f),v.d(f,{loadFireworksPreset:function(){return y}});var e=v(1),t=v(919),o=v(941),i=v(841),r=v(949),a=v(489),n=v(45),s=v(838),l=v(364),u=v(328),c=v(818);function d(e,t,o,i,r){var a,n;const s=t;if(!s||!s.enable)return;const l=(0,c.randomInRange)(o.offset),u=(null!==(a=t.velocity)&&void 0!==a?a:0)*e.factor+3.6*l,d=null!==(n=t.decay)&&void 0!==n?n:1;r&&0!==s.status?(s.value-=u,s.value<0&&(s.status=0,s.value+=s.value)):(s.value+=u,r&&s.value>i&&(s.status=1,s.value-=s.value%i)),s.velocity&&1!==d&&(s.velocity*=d),s.value>i&&(s.value%=i)}class p{constructor(e){this.container=e}init(e){var t,o;const i=this.container;e.stroke=e.options.stroke instanceof Array?(0,c.itemFromArray)(e.options.stroke,e.id,e.options.reduceDuplicates):e.options.stroke,e.strokeWidth=e.stroke.width*i.retina.pixelRatio;const r=null!==(t=(0,c.rangeColorToHsl)(e.stroke.color))&&void 0!==t?t:e.getFillColor();r&&(e.strokeColor=(0,c.getHslAnimationFromHsl)(r,null===(o=e.stroke.color)||void 0===o?void 0:o.animation,i.retina.reduceFactor))}isEnabled(e){var t,o,i,r;const a=null===(t=e.stroke)||void 0===t?void 0:t.color;return!e.destroyed&&!e.spawning&&!!a&&(void 0!==(null===(o=e.strokeColor)||void 0===o?void 0:o.h.value)&&a.animation.h.enable||void 0!==(null===(i=e.strokeColor)||void 0===i?void 0:i.s.value)&&a.animation.s.enable||void 0!==(null===(r=e.strokeColor)||void 0===r?void 0:r.l.value)&&a.animation.l.enable)}update(e,t){this.isEnabled(e)&&function(e,t){var o,i,r,a,n,s,l,u,c,p;if(!(null===(o=e.stroke)||void 0===o?void 0:o.color))return;const v=e.stroke.color.animation,f=null!==(r=null===(i=e.strokeColor)||void 0===i?void 0:i.h)&&void 0!==r?r:null===(a=e.color)||void 0===a?void 0:a.h;f&&d(t,f,v.h,360,!1);const m=null!==(s=null===(n=e.strokeColor)||void 0===n?void 0:n.s)&&void 0!==s?s:null===(l=e.color)||void 0===l?void 0:l.s;m&&d(t,m,v.s,100,!0);const w=null!==(c=null===(u=e.strokeColor)||void 0===u?void 0:u.l)&&void 0!==c?c:null===(p=e.color)||void 0===p?void 0:p.l;w&&d(t,w,v.l,100,!0)}(e,t)}}const m=(e,t,o)=>{const i=e.max>o?e.max-o:0;let r=(0,c.setRangeValue)(e);i&&(r=(0,c.setRangeValue)(e.min-i,o));const a=e.min<t?e.min:0;return a&&(r=(0,c.setRangeValue)(0,e.max+a)),r},w={detectRetina:!0,background:{color:"#000"},fpsLimit:120,emitters:{direction:"top",life:{count:0,duration:.1,delay:.1},rate:{delay:.25,quantity:1},size:{width:100,height:0},position:{y:100,x:50}},particles:{number:{value:0},destroy:{mode:"split",split:{count:1,factor:{value:.333333},rate:{value:200},particles:["#ff595e","#ffca3a","#8ac926","#1982c4","#6a4c93"].map((e=>{const t=(0,c.stringToRgb)(e);if(!t)return;const o=(0,c.rgbToHsl)(t),i=m({min:o.s-20,max:o.s+20},0,100),r=m({min:o.l-20,max:o.l+20},0,100);return{color:{value:{h:o.h,s:i,l:r}},stroke:{width:0},number:{value:0},collisions:{enable:!1},opacity:{value:{min:.1,max:1},animation:{enable:!0,speed:.7,sync:!1,startValue:"max",destroy:"min"}},shape:{type:"circle"},size:{value:2,animation:{enable:!1}},life:{count:1,duration:{value:{min:1,max:2}}},move:{enable:!0,gravity:{enable:!1},speed:2,direction:"none",random:!0,straight:!1,outModes:"destroy"}}})).filter((e=>void 0!==e))}},life:{count:1},shape:{type:"line"},size:{value:{min:.1,max:50},animation:{enable:!0,sync:!0,speed:90,startValue:"max",destroy:"min"}},stroke:{color:{value:"#ffffff"},width:1},rotate:{path:!0},move:{enable:!0,gravity:{acceleration:15,enable:!0,inverse:!0,maxSpeed:100},speed:{min:10,max:20},outModes:{default:"destroy",top:"none"},trail:{fillColor:"#000",enable:!0,length:10}}}};async function y(c){await(0,t.loadBaseMover)(c),await(0,r.loadEmittersPlugin)(c),await(0,o.loadCircleShape)(c),await(0,n.loadLineShape)(c),await(0,e.loadAngleUpdater)(c),await(0,i.loadColorUpdater)(c),await(0,a.loadLifeUpdater)(c),await(0,s.loadOpacityUpdater)(c),await(0,l.loadOutModesUpdater)(c),await(0,u.loadSizeUpdater)(c),await async function(e){await e.addParticleUpdater("strokeColor",(e=>new p(e)))}(c),await c.addPreset("fireworks",w)}}(),f}()})); |
@@ -7,3 +7,3 @@ (function (factory) { | ||
else if (typeof define === "function" && define.amd) { | ||
define(["require", "exports"], factory); | ||
define(["require", "exports", "tsparticles-engine"], factory); | ||
} | ||
@@ -14,2 +14,84 @@ })(function (require, exports) { | ||
exports.options = void 0; | ||
const tsparticles_engine_1 = require("tsparticles-engine"); | ||
const fixRange = (value, min, max) => { | ||
const diffSMax = value.max > max ? value.max - max : 0; | ||
let res = (0, tsparticles_engine_1.setRangeValue)(value); | ||
if (diffSMax) { | ||
res = (0, tsparticles_engine_1.setRangeValue)(value.min - diffSMax, max); | ||
} | ||
const diffSMin = value.min < min ? value.min : 0; | ||
if (diffSMin) { | ||
res = (0, tsparticles_engine_1.setRangeValue)(0, value.max + diffSMin); | ||
} | ||
return res; | ||
}; | ||
const fireworksOptions = ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"] | ||
.map((color) => { | ||
const rgb = (0, tsparticles_engine_1.stringToRgb)(color); | ||
if (!rgb) { | ||
return undefined; | ||
} | ||
const hsl = (0, tsparticles_engine_1.rgbToHsl)(rgb), sRange = fixRange({ min: hsl.s - 20, max: hsl.s + 20 }, 0, 100), lRange = fixRange({ min: hsl.l - 20, max: hsl.l + 20 }, 0, 100); | ||
return { | ||
color: { | ||
value: { | ||
h: hsl.h, | ||
s: sRange, | ||
l: lRange, | ||
}, | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}; | ||
}) | ||
.filter((t) => t !== undefined); | ||
exports.options = { | ||
@@ -29,3 +111,3 @@ detectRetina: true, | ||
rate: { | ||
delay: 0.5, | ||
delay: 0.25, | ||
quantity: 1, | ||
@@ -54,60 +136,5 @@ }, | ||
rate: { | ||
value: 100, | ||
value: 200, | ||
}, | ||
particles: { | ||
color: { | ||
value: ["#ff595e", "#ffca3a", "#8ac926", "#1982c4", "#6a4c93"], | ||
}, | ||
stroke: { | ||
width: 0, | ||
}, | ||
number: { | ||
value: 0, | ||
}, | ||
collisions: { | ||
enable: false, | ||
}, | ||
opacity: { | ||
value: { | ||
min: 0.1, | ||
max: 1, | ||
}, | ||
animation: { | ||
enable: true, | ||
speed: 0.7, | ||
sync: false, | ||
startValue: "max", | ||
destroy: "min", | ||
}, | ||
}, | ||
shape: { | ||
type: "circle", | ||
}, | ||
size: { | ||
value: 2, | ||
animation: { | ||
enable: false, | ||
}, | ||
}, | ||
life: { | ||
count: 1, | ||
duration: { | ||
value: { | ||
min: 1, | ||
max: 2, | ||
}, | ||
}, | ||
}, | ||
move: { | ||
enable: true, | ||
gravity: { | ||
enable: false, | ||
}, | ||
speed: 2, | ||
direction: "none", | ||
random: true, | ||
straight: false, | ||
outModes: "destroy", | ||
}, | ||
}, | ||
particles: fireworksOptions, | ||
}, | ||
@@ -114,0 +141,0 @@ }, |
Sorry, the diff of this file is not supported yet
Sorry, the diff of this file is too big to display
Sorry, the diff of this file is too big to display
834705
9817
167
Updatedtsparticles-engine@^2.1.0
Updatedtsparticles-move-base@^2.1.0