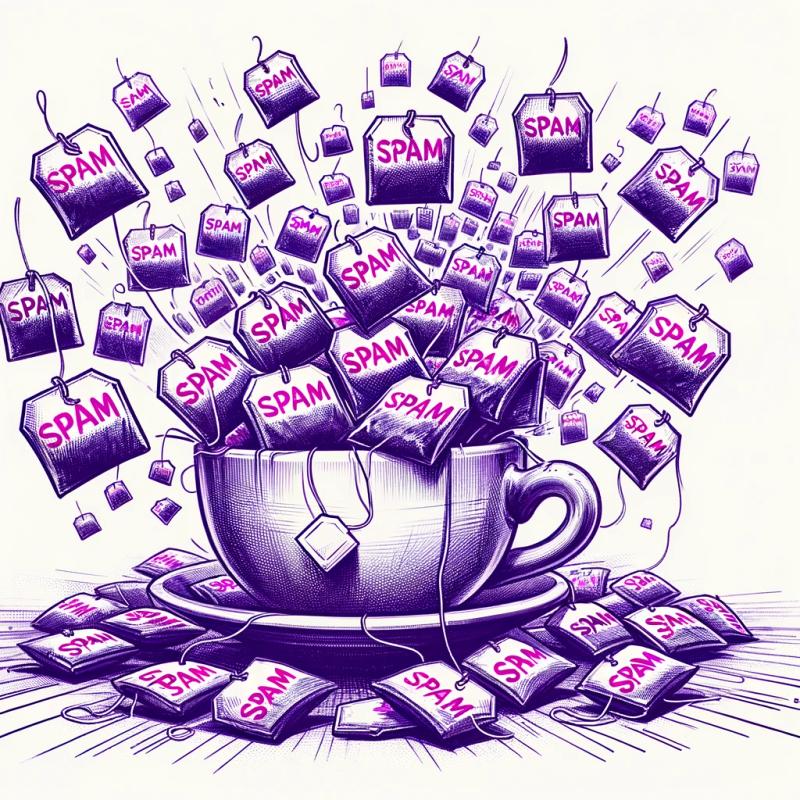
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
tssrp6a
Advanced tools
Readme
This library is a TypeScript implementation of Secure Remote Password SRP6a.
SRP allows a user to authenticate to a server without sending the password (zero-knowledge proof of password) using generated private/public keys.
See https://en.wikipedia.org/wiki/Secure_Remote_Password_protocol
https://tools.ietf.org/html/rfc5054
for all the details.
You can see a real-time demo here.
The target
of TypeScript output is es6
.
This package has zero dependencies. It only needs BigInt native support, or a polyfill which is not included.
Note: This module makes use of Crypto.subtle
and therefore only works on HTTPS.
The user requests a registration page, the browser will generate a salt and take the user's identity and password and generate a verifier.
The browser sends email, salt, verifier to server. The server saves this to storage.
Here is a complete example of signup:
import {
createVerifierAndSalt, SRPParameters, SRPRoutines,
} from "tssrp6a"
(async ()=> {
const srp6aNimbusRoutines = new SRPRoutines(new SRPParameters());
const userId = "hello@world.org";
const userPassword = "password";
const { s: salt, v: verifier } = await createVerifierAndSalt(
srp6aNimbusRoutines,
userId,
userPassword,
);
// store salt and verifier in a database
})()
The user starts an authentication session by entering his id and password.
The id is sent with a request to the server, which finds salt and verifier for that id. Server executes step1 to generate private key b
and public key B
, and responds to browser with salt
and B
.
The browser generates private key a
, public key A
and computes M1
. Browser makes requests with A
and M1
.
Server verifies that the credentials were correct with step2, using b
and M1
. If successful, it also takes A
and generates and responds with M2
.
Browser may additionally verify the authority of the server from M2
with step3.
Note: a
and b
are generated for one authentication "session" and discarded immediately.
Here is a complete example of authentication session:
import {
createVerifierAndSalt, SRPClientSession, SRPParameters, SRPRoutines,
SRPServerSession
} from "tssrp6a"
(async ()=> {
const srp6aNimbusRoutines = new SRPRoutines(new SRPParameters());
const username = "hello@world.org";
let password = "password";
// Sign up
const {s: salt, v: verifier} = await createVerifierAndSalt(
srp6aNimbusRoutines,
username,
password,
);
// Sign in
const srp6aNimbusClient = new SRPClientSession(srp6aNimbusRoutines);
await srp6aNimbusClient.step1(username, password);
// erase password at this point, it is no longer stored
password = ""
const server = new SRPServerSession(srp6aNimbusRoutines);
// server gets identifier from client, salt+verifier from db (from signup)
const B = await server.step1(username, salt, verifier);
// client gets challenge B from server step1 and sends prove M1 to server
const {A, M1} = await srp6aNimbusClient.step2(salt, B);
// servers checks client prove M1 and sends server prove M2 to client
const M2 = await server.step2(A, M1);
// client ensures server identity
await srp6aNimbusClient.step3(M2);
})()
SRP alone only prevents a man-in-the-middle attack from reading the password, but such an attack could also inject code into the browser to hijack the password.
Always use SRP in combination with HTTPS. Browsers can be vulnerable to: having malicious certificates installed beforehand, rogue certificates in the wild, server misconfiguration, bugs like the heartbleed attack, servers leaking password into errors and logs. SRP in the browser offers an additional hurdle and may prevent some mistakes from escalating.
The client can choose to exclude the identity of its computations or not. If excluded, the id cannot be changed. But this problem is better solved by an application schema that separates "identity" from "authentication", so that one identity can have multiple authentications. This allows to switch identity + password, and also to user more than one way of logging in (think "login with email+password, google, or facebook").
The SRP protocol and therefore this library is stateful. Each step sets various internal state. Due to the randomness of some of this state (namely the public and private values), repeating the step methods with the same arguments is unlikely (almost definitely) to result in the same state. This proves to be an issue when using a stateless protocol such as HTTP (as opposed to websockets). The server "session" state (the server step 1 state) might not be easily kept in memory. Therefore, we provide a way to serialize and deserialize the step classes in order to restore state. serialize.test.ts shows some examples here's an explanation of how it works:
const serverStep1 = await new SRPServerSession(TEST_ROUTINES).step1(...); // Each step returns a class, in this case .step1 returns SRPServerSessionStep1
const serializedServerStep1 = JSON.stringify(serverStep1); // Some of the step methods (see below for which ones) have a .toJSON method that returns the internal state. JSON.stringify calls .toJSON
// you can now store serializedServerStep1 in a database or elsewhere. There are security implications, see below.
// when you are ready to restore the state, call fromState on the same step class used to serialize the data (in this case SRPServerSessionStep1) to deserialize
const deserializedServerStep1 = SRPServerSessionStep1.fromState(
TEST_ROUTINES, // first param is the routines
JSON.parse(serializedServerStep1),
);
// deserializedServerStep1 is now functionally equivilent as serverStep1 because it contains the same state
Supported steps/classes for serialization are:
SRPServerSessionStep1
SRPClientSessionStep1
SRPClientSessionStep2
While the password is never kept directly in the state, hashes of it are. If an adversary is able to access the serialized state it will likely open you up to some kind of MITM attack and depending on the step, may allow an attacker to perform a bruteforce and/or dictionary attack to retrieve the password. Do not expose the serialized data. For clients, this means do not send it over the network and be careful where you store it. For servers, only send it in encrypted form to parties you trust (such as your database). If you believe state at anytime may have been exposed, it is suggested you change passwords as soon as possible.
This package's default configuration matches the following Java's Nimbus SRP configuration:
SRP6CryptoParams.getInstance(2048, "SHA-512")
The default routines does not strictly follow SRP6a RFC because user identity is NOT included in the verifier generation. This makes possible for malicious server to detect if two users share the same password but also allows client to change it "identity" without regenerating password.
This example shows how to make SRP client strictly compliant with SRP6a specification.
FAQs
SRP6a client and server lib
The npm package tssrp6a receives a total of 97 weekly downloads. As such, tssrp6a popularity was classified as not popular.
We found that tssrp6a demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.