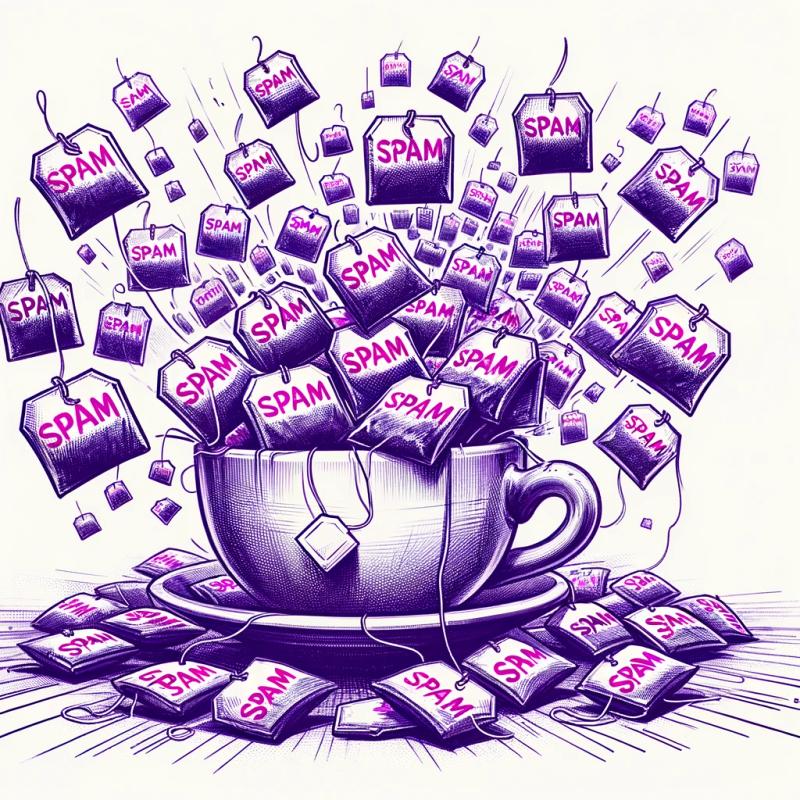
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
tsynamo
Advanced tools
Readme
Type-friendly DynamoDB query builder! Inspired by Kysely.
Usable with AWS SDK v3 DynamoDBDocumentClient
.
Available in NPM.
npm i tsynamo
pnpm install tsynamo
yarn add tsynamo
import { PartitionKey, SortKey } from "tsynamo";
export interface DDB {
UserEvents: {
userId: PartitionKey<string>;
eventId: SortKey<number>;
eventType: string;
userAuthenticated: boolean;
};
}
Notice that you can have multiple tables in the DDB schema. Nested attributes are supported too.
import { DynamoDBClient } from "@aws-sdk/client-dynamodb";
import { DynamoDBDocumentClient } from "@aws-sdk/lib-dynamodb";
const ddbClient = DynamoDBDocumentClient.from(
new DynamoDBClient({
/* Configure client... */
})
);
The document client must come from @aws-sdk/lib-dynamodb!
const tsynamoClient = new Tsynamo<DDB>({
ddbClient: dynamoDbDocumentClient,
});
await tsynamoClient
.getItem("UserEvents")
.keys({
userId: "123",
eventId: 222,
})
.attributes(["userId"])
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.keyCondition("eventId", "<", 1000)
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.filterExpression("eventType", "=", "LOG_IN_EVENT")
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.filterExpression("eventType", "begins_with", "LOG")
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.filterExpression("eventType", "begins_with", "LOG_IN")
.orFilterExpression("eventType", "begins_with", "SIGN_IN")
.execute();
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.filterExpression("eventType", "=", "LOG_IN")
.orFilterExpression((qb) =>
qb
.filterExpression("eventType", "=", "UNAUTHORIZED_ACCESS")
.filterExpression("userAuthenticated", "=", true)
)
.orFilterExpression("eventType", "begins_with", "SIGN_IN")
.execute();
This would compile as the following FilterExpression:
eventType = "LOG_IN" OR (eventType = "UNAUTHORIZED_ACCESS" AND userAuthenticated = true
)
await tsynamoClient
.query("UserEvents")
.keyCondition("userId", "=", "123")
.filterExpression("NOT", (qb) =>
qb.filterExpression("eventType", "=", "LOG_IN")
)
.execute();
This would compile as the following FilterExpression:
NOT eventType = "LOG_IN"
, i.e. return all events whose types is not "LOG_IN"
await tsynamoClient
.putItem("myTable")
.item({
userId: "123",
eventId: 313,
})
.execute();
await tsynamoClient
.putItem("myTable")
.item({
userId: "123",
eventId: 313,
})
.conditionExpression("userId", "attribute_not_exists")
.execute();
await tsynamoClient
.putItem("myTable")
.item({
userId: "123",
eventId: 313,
})
.conditionExpression("userId", "attribute_not_exists")
.orConditionExpression("eventType", "begins_with", "LOG_")
.execute();
await tsynamoClient
.deleteItem("myTable")
.keys({
userId: "123",
eventId: 313,
})
.execute();
await tsynamoClient
.deleteItem("myTable")
.keys({
userId: "123",
eventId: 313,
})
.conditionExpression("eventType", "attribute_not_exists")
.execute();
WIP
WIP
FAQs
Typed query builder for DynamoDB
The npm package tsynamo receives a total of 0 weekly downloads. As such, tsynamo popularity was classified as not popular.
We found that tsynamo demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.