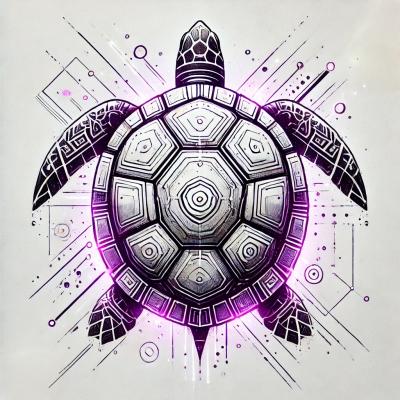
Security Fundamentals
Turtles, Clams, and Cyber Threat Actors: Shell Usage
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
twitchclient
Advanced tools
Lightweight (2kb gzipped) Twitch client the Browser to connect to the chat and interact with it. Can also do some Api calls like following/unfollowing channels.
Lightweight 2.5kb gzipped Twitch client for the Browser to connect to the chat and interact with it. Supports third party emotes from BetterTTV & FrankerFaceZ.
npm i twitchclient
let message = {
raw: '@badge-info=subscriber/1;badges=subscriber/0;color=#1E90FF;display-name=BDJoe_;emotes=120232:13-19;flags=;id=5a253ad7-7e42-4021-a364-44d0c9af7821;mod=0;room-id=22484632;subscriber=1;tmi-sent-ts=1568822980541;turbo=0;user-id=453114423;user-type= :bdjoe_!bdjoe_@bdjoe_.tmi.twitch.tv PRIVMSG #forsen :TriHard Kapp forsenPls forsenPls test',
msg_type: 'PRIVMSG',
modified_irc: '@badge-info=subscriber/1;badges=subscriber/0;color=#1E90FF;display-name=BDJoe_;emotes=120232:13-19;flags=;id=5a253ad7-7e42-4021-a364-44d0c9af7821;mod=0;room-id=22484632;subscriber=1;tmi-sent-ts=1568822980541;turbo=0;user-id=453114423;user-type=;msg-before=bdjoe_!bdjoe_@bdjoe_.tmi.twitch.tv;msg-type=PRIVMSG;channel=#forsen;msg-content=TriHard Kapp forsenPls forsenPls test',
badge_info: 'subscriber/1',
badges: 'subscriber/0',
color: '#1E90FF',
display_name: 'BDJoe_',
emotes: '120232:13-19',
flags: '',
id: '5a253ad7-7e42-4021-a364-44d0c9af7821',
mod: '0',
room_id: '22484632',
subscriber: '1',
tmi_sent_ts: '1568822980541',
turbo: '0',
user_id: '453114423',
user_type: '',
msg_before: 'bdjoe_!bdjoe_@bdjoe_.tmi.twitch.tv',
channel: '#forsen',
msg_content: 'TriHard Kapp forsenPls forsenPls test',
parsed_emotes: {"Twitch":[{"name":"TriHard","urls":["https://static-cdn.jtvnw.net/emoticons/v1/120232/1.0","https://static-cdn.jtvnw.net/emoticons/v1/120232/2.0","https://static-cdn.jtvnw.net/emoticons/v1/120232/3.0"],"host":"Twitch","indexes":"0-6,"}],"BTTV":[{"name":"forsenPls","urls":["https://cdn.betterttv.net/emote/55e2096ea6fa8b261f81b12a/1x","https://cdn.betterttv.net/emote/55e2096ea6fa8b261f81b12a/2x","https://cdn.betterttv.net/emote/55e2096ea6fa8b261f81b12a/3x"],"host":"BTTV","indexes":"13-23,23-33,"}],"FFZ":[{"name":"Kapp","urls":["https://cdn.frankerfacez.com/93d93138f176a98e4b09c81e8de56372.PNG","https://cdn.frankerfacez.com/6e50ed6052f4398ffc67a92ee782d79d.PNG","https://cdn.frankerfacez.com/f92ee27b25b813e2618b0d34a96402b5.png"],"host":"FFZ","indexes":"8-13,"}]},
msg_html: '<img src=\"https://static-cdn.jtvnw.net/emoticons/v1/120232/1.0\"> <img src=\"https://cdn.frankerfacez.com/93d93138f176a98e4b09c81e8de56372.PNG\"> <img src=\"https://cdn.betterttv.net/emote/55e2096ea6fa8b261f81b12a/1x\"> <img src=\"https://cdn.betterttv.net/emote/55e2096ea6fa8b261f81b12a/1x\"> test ',
}
<html>
<head>
<!-- <script src="https://unpkg.com/twitchclient@0.0.7/lib/twitchclient.browser.js"></script> -->
<script src="https://unpkg.com/twitchclient/lib/twitchclient.browser.js"></script>
<script>
let twitchClient = new TwitchClient("gzipped", "fw7kd79xuzpjwu8qbl4c18we78fssd", true);
twitchClient.joinChannel("forsen");
twitch.on("LOG", log => {
console.log(log);
})
twitch.on("PRIVMSG", privmsg => {
document.querySelector("body").innerHTML += `<p>[${privmsg.channel}] ${privmsg.display_name}: ${privmsg.msg_html}</p>`;
})
</script>
</head>
<body>
</body>
</html>
TwitchClient()
Creates a new TwitchClient().
let twitchClient = new TwitchClient(); // Anon
let twitchClient = new TwitchClient("forsen", "oauthtokenwithscope", true); // Username - OAuth Token - Third party emote parsing
// You can get the OAuth token from https://twitchapps.com/tmi/ for example or create your own https://dev.twitch.tv/docs/authentication/getting-tokens-oauth/
joinChannel()
Joins a Twitch channel.
TwitchClient.joinChannel("forsen")
leaveChannel()
Leaves a Twitch channel.
TwitchClient.leaveChannel("forsen")
parseMessage()
Parses a raw IRC message to an object.
TwitchClient.parseMessage("@badge-info=subscriber/1;badges=subscriber/0;color=#1E90FF;dis...")
sendMessage()
Sends a message to a specific channel.
TwitchClient.sendMessage("forsen", "TriHard")
on("LOG")
This event is emitted when something happens what could be important for debugging.
TwitchClient.on("LOG", log => { . . .});
on("PRIVMSG")
This event is emitted when a message has been sent.
TwitchClient.on("PRIVMSG", privmsg => { . . .});
on("CLEARCHAT")
This event is emitted when a user gets timeouted or banned.
TwitchClient.on("CLEARCHAT", clearchat => { . . .});
on("CLEARMSG")
This event is emitted when a single message got deleted via /delete .
TwitchClient.on("CLEARMSG", clearmsg => { . . .});
on("GLOBALUSERSTATE")
This event is emitted when a message has been sent.
TwitchClient.on("GLOBALUSERSTATE", globaluserstate => { . . .});
on("ROOMSTATE")
This event is emitted when a user joins the chat or room setting got changed.
TwitchClient.on("ROOMSTATE", roomstate => { . . .});
on("USERNOTICE")
This event is emitted when any of several events occurs.
TwitchClient.on("USERNOTICE", usernotice => { . . .});
on("USERSTATE")
This event is emitted when a user joins a channel or sends a PRIVMSG to a channel.
TwitchClient.on("USERSTATE", userstate => { . . .});
FAQs
Lightweight (2kb gzipped) Twitch client the Browser to connect to the chat and interact with it. Can also do some Api calls like following/unfollowing channels.
The npm package twitchclient receives a total of 20 weekly downloads. As such, twitchclient popularity was classified as not popular.
We found that twitchclient demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security Fundamentals
The Socket Threat Research Team uncovers how threat actors weaponize shell techniques across npm, PyPI, and Go ecosystems to maintain persistence and exfiltrate data.
Security News
At VulnCon 2025, NIST scrapped its NVD consortium plans, admitted it can't keep up with CVEs, and outlined automation efforts amid a mounting backlog.
Product
We redesigned our GitHub PR comments to deliver clear, actionable security insights without adding noise to your workflow.