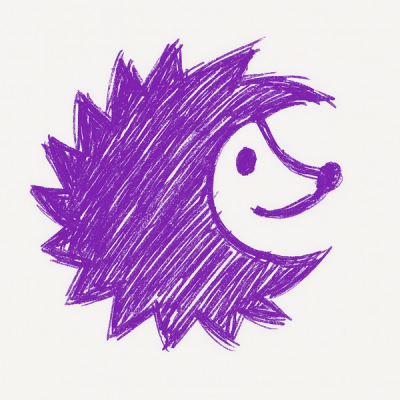
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
twitter-client-typescript
Advanced tools
Python client for interacting with Twitter V2 API available at https://rapidapi.com/datarise-datarise-default/api/twitter-x
This is a TypeScript package that provides an asynchronous client for interacting with the Twitter V2 API via the RapidAPI platform. This client provides various methods for accessing Twitter data.
To install the package, use npm or yarn:
npm install twitter-client-typescript
or
yarn add twitter-client-typescript
To use the client, you will need to create an instance of the AsyncTwitterClient
class and provide your RapidAPI key:
import { AsyncTwitterClient } from 'twitter-client-typescript';
const client = new AsyncTwitterClient({ apiKey: "YOUR_API_KEY" });
// Use the client to make API calls
// Search for tweets
client.search('elon musk').then((response) => {
result = response.data;
console.log(result);
}).catch((error) => {
console.error(error);
});
// Get user details
client.userDetails('elonmusk').then((response) => {
result = response.data;
console.log(result);
}).catch((error) => {
console.error(error);
});
// Get user tweets
client.userTweets('elonmusk').then((response) => {
result = response.data;
console.log(result);
}).catch((error) => {
console.error(error);
});
AsyncTwitterClient class supports batch requests. You can make multiple requests in parallel and get the results in a single response. Here is an example:
import { AsyncTwitterClient } from 'twitter-client-typescript';
const client = new AsyncTwitterClient({ apiKey: "YOUR_API_KEY", timeout: 10000 });
const users = ['elonmusk', 'BillGates', 'JeffBezos', 'tim_cook', 'satyanadella'];
// Create an array of promises
const promises = users.map((user) => client.userDetails(user));
// Make the requests in parallel
Promise.all(promises).then((responses) => {
responses.forEach((response, index) => {
console.log(users[index], response.data);
});
}).catch((error) => {
console.error(error);
});
You can check the rate limit of the API using the rateLimit
method. AsyncTwitterClient
has a rateLimit
attribute that returns the rate limit details. It's updated after each request.
import { AsyncTwitterClient } from 'twitter-client-typescript';
const client = new AsyncTwitterClient({apiKey: 'YOUR_RAPID_API_KEY'});
// Get user details
client.userDetails('elonmusk').then((response) => {
result = response.data;
console.log(result);
}).catch((error) => {
console.error(error);
});
// Check rate limit
console.log(`Limit : ${client.rateLimit.limit}`);
console.log(`Remaining requests: ${client.rateLimit.remaining}`);
console.log(`Reset time: ${client.rateLimit.reset}`);
This project is licensed under the MIT License - see the LICENSE file for details.
If you have any questions or feedback, feel free to reach out to us at contact [at] datarise [dot] ma.
FAQs
Python client for interacting with Twitter V2 API available at https://rapidapi.com/datarise-datarise-default/api/twitter-x
The npm package twitter-client-typescript receives a total of 0 weekly downloads. As such, twitter-client-typescript popularity was classified as not popular.
We found that twitter-client-typescript demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.