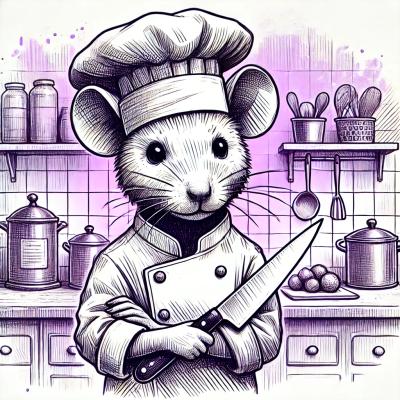
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
universalify
Advanced tools
Make a callback- or promise-based function support both promises and callbacks.
The universalify npm package is designed to convert callback-based functions to ones that return promises, allowing for both callback and promise-based usage. This is particularly useful for supporting legacy code while taking advantage of the benefits of promises in newer code.
fromCallback
Converts a function that uses a callback to one that returns a promise, but still supports the callback interface.
const universalify = require('universalify');
const fs = require('fs');
const readFileAsync = universalify.fromCallback(fs.readFile);
// Using as a promise
readFileAsync('example.txt', 'utf8').then(contents => {
console.log(contents);
}).catch(error => {
console.error('Error reading file:', error);
});
// Using with a callback
readFileAsync('example.txt', 'utf8', (error, contents) => {
if (error) {
console.error('Error reading file:', error);
return;
}
console.log(contents);
});
fromPromise
Converts a promise-returning function to one that supports both promises and callbacks.
const universalify = require('universalify');
const fsPromises = require('fs').promises;
const readFile = universalify.fromPromise(fsPromises.readFile);
// Using as a promise
readFile('example.txt', 'utf8').then(contents => {
console.log(contents);
}).catch(error => {
console.error('Error reading file:', error);
});
// Using with a callback
readFile('example.txt', 'utf8', (error, contents) => {
if (error) {
console.error('Error reading file:', error);
return;
}
console.log(contents);
});
Pify is a package that promisifies functions. It converts functions that use node-style callbacks into functions that return promises. Unlike universalify, pify does not maintain callback support once promisified.
Bluebird is a comprehensive promise library that includes a promisify feature to convert callback-based functions into promise-returning functions. It is more feature-rich than universalify but also larger in size and does not support callbacks after promisification.
Util.promisify is a built-in Node.js utility function that converts a callback-based function into a promise-based one. It is similar to universalify but is limited to Node.js and does not support the original callback interface after conversion.
Make a callback- or promise-based function support both promises and callbacks.
Uses the native promise implementation.
npm install universalify
universalify.fromCallback(fn)
Takes a callback-based function to universalify, and returns the universalified function.
Function must take a callback as the last parameter that will be called with the signature (error, result)
. universalify
does not support calling the callback with three or more arguments, and does not ensure that the callback is only called once.
function callbackFn (n, cb) {
setTimeout(() => cb(null, n), 15)
}
const fn = universalify.fromCallback(callbackFn)
// Works with Promises:
fn('Hello World!')
.then(result => console.log(result)) // -> Hello World!
.catch(error => console.error(error))
// Works with Callbacks:
fn('Hi!', (error, result) => {
if (error) return console.error(error)
console.log(result)
// -> Hi!
})
universalify.fromPromise(fn)
Takes a promise-based function to universalify, and returns the universalified function.
Function must return a valid JS promise. universalify
does not ensure that a valid promise is returned.
function promiseFn (n) {
return new Promise(resolve => {
setTimeout(() => resolve(n), 15)
})
}
const fn = universalify.fromPromise(promiseFn)
// Works with Promises:
fn('Hello World!')
.then(result => console.log(result)) // -> Hello World!
.catch(error => console.error(error))
// Works with Callbacks:
fn('Hi!', (error, result) => {
if (error) return console.error(error)
console.log(result)
// -> Hi!
})
MIT
FAQs
Make a callback- or promise-based function support both promises and callbacks.
The npm package universalify receives a total of 32,712,302 weekly downloads. As such, universalify popularity was classified as popular.
We found that universalify demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.