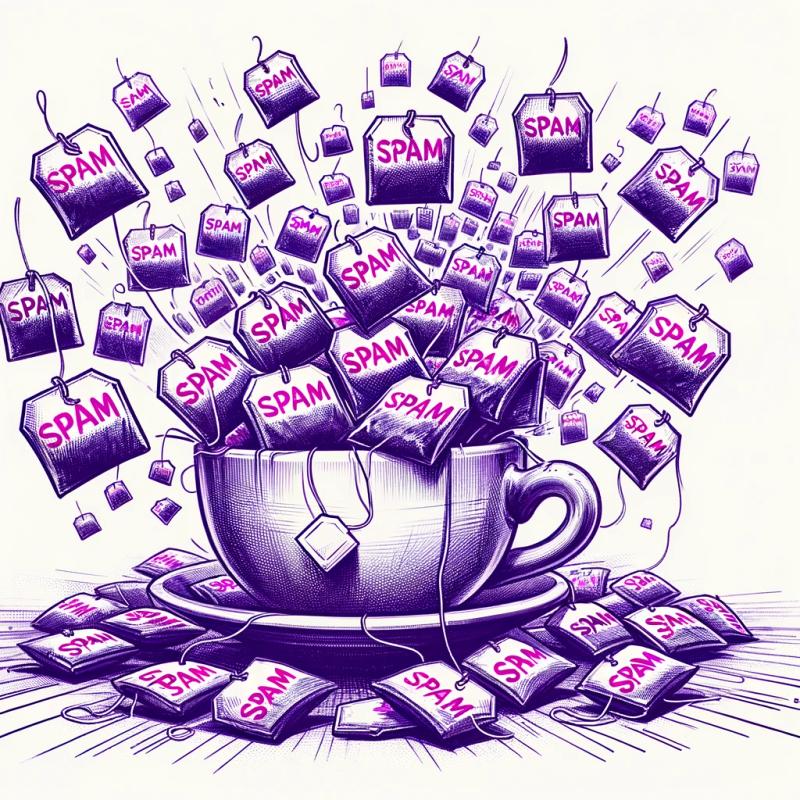
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
use-awaitable-component
Advanced tools
Readme
React hook for awaiting component callback.
npm
npm install use-awaitable-component
yarn
yarn add use-awaitable-component
import { useState } from "react";
import useAwaitableComponent from "use-awaitable-component";
import "./styles.css";
function Modal({ visible, onSubmit, onCancel }) {
const [text, setText] = useState("");
const display = visible ? "block" : "none";
const handleSubmit = () => {
onSubmit(text);
setText("");
};
const handleCancel = () => {
onCancel(":)");
};
return (
<div className="modal" style={{ display }}>
<div className="modal-head">This is a modal</div>
<div className="modal-body">
<input
placeholder="Type something here..."
value={text}
onChange={(e) => setText(e.target.value)}
/>
<button onClick={handleSubmit}>Submit</button>
</div>
<button className="modal-close" onClick={handleCancel}>
X
</button>
</div>
);
}
export default function App() {
const [status, execute, resolve, reject, reset] = useAwaitableComponent();
const showModal = status === "awaiting";
const handleAwaitModal = async () => {
try {
const value = await execute();
alert(`VALUE: ${value}`);
} catch (err) {
alert(`Canceled: ${err}`);
} finally {
reset();
}
};
return (
<div className="App">
<div className="button-container">
<button disabled={showModal} onClick={handleAwaitModal}>
{showModal ? "Waiting..." : "Show Modal"}
</button>
</div>
<Modal visible={showModal} onSubmit={resolve} onCancel={reject} />
</div>
);
}
See live demo on Codesandbox.
const [status, execute, resolve, reject, reset] = useAwaitableComponent()
Field | Type | Descriptions |
---|---|---|
status | 'idle' | 'awaiting' | 'resolved' | 'rejected' | Current awaitable component status |
execute | () => Promise | A function to start execution |
resolve | (data: any) => void | A callback to call after component completed without error |
reject | (reason: any) => void | A callback to call after component completed with error |
reset | () => void | A function to reset status back to idle . This function must be called before calling execute() again. |
FAQs
React hook for awaiting component callback.
The npm package use-awaitable-component receives a total of 767 weekly downloads. As such, use-awaitable-component popularity was classified as not popular.
We found that use-awaitable-component demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.