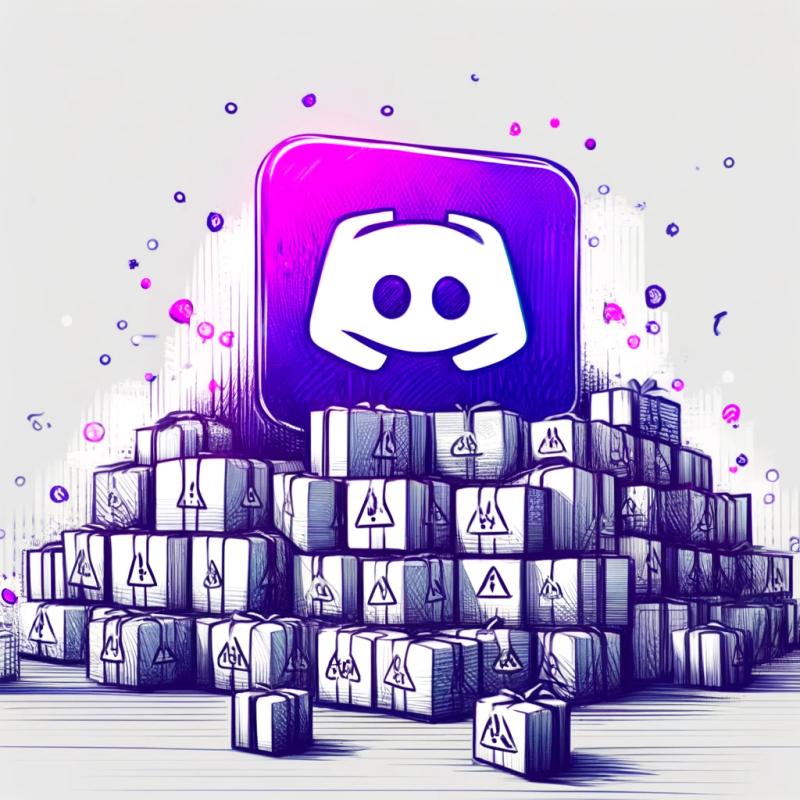
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
use-tiny-state-machine
Advanced tools
Readme
A tiny (~700 bytes) react hook to help you write finite state machines
Read the documentation for more information
yarn add use-tiny-state-machine
import useTinyStateMachine from 'use-tiny-state-machine';
const stateChart = {
id: 'traffixLight',
initial: 'green',
states: {
green: { on: { NEXT: 'red' } },
orange: { on: { NEXT: 'green' } },
red: { on: { NEXT: 'orange' } },
},
};
export default function ManualTrafficLights() {
const { cata, state, dispatch } = useTinyStateMachine(stateChart);
return (
<Fragment>
<div
className="trafficLight"
style={{
backgroundColor: cata({
green: '#51e980',
red: '#e74c3c',
orange: '#ffa500',
}),
}}
>
The light is {state}
</div>
<button onClick={() => dispatch('NEXT')}>Next light</button>
</Fragment>
);
};
onEntry
is called every time you enter a given state. onEntry
is called with the current state machine instance.
import useTinyStateMachine from 'use-tiny-state-machine';
const stateChart = {
id: "traffixLight",
initial: "green",
states: {
green: {
onEntry: waitForNextLight,
on: {
NEXT: "red"
}
},
orange: {
onEntry: waitForNextLight,
on: {
NEXT: "green"
}
},
red: {
onEntry: waitForNextLight,
on: {
NEXT: "orange"
}
}
}
};
function waitForNextLight({ dispatch }) {
const timer = setTimeout(() => dispatch('NEXT'), 1000);
return () => clearTimeout(timer);
}
function TrafficLights() {
const { cata, state, dispatch } = useTinyStateMachine(stateChart);
return (
<Fragment>
<div
style={{
width: "30px",
height: "30px",
backgroundColor: cata({
green: "#51e980",
red: "#e74c3c",
orange: "#ffa500"
})
}}
>
The light is {state}
</div>
<button onClick={() => dispatch("NEXT")}>Force next light</button>
</Fragment>
);
}
You can use context to store any data associated with a state.
const stateChart = {
id: 'userData',
initial: 'idle',
context: {
data: null,
error: null,
},
states: {
idle: {
on: {
FETCH: {
target: 'pending',
action: ({ dispatch }, userId) => {
fetchUser(userId)
.then(user => dispatch('SUCCESS', user))
.catch(error => dispatch('FAILURE', error));
},
},
},
},
pending: {
on: {
SUCCESS: {
target: 'success',
beforeStateChange: ({ updateContext }, data) => updateContext(c => ({ ...c, data })),
},
FAILURE: {
target: 'failure',
beforeStateChange: ({ updateContext }, error) => updateContext(c => ({ ...c, error })),
},
},
},
},
};
const UserData = () => {
const { context, dispatch, cata } = useTinyStateMachine(stateChart);
return (
<div>
{cata({
idle: () => (
<button onClick={() => dispatch('FETCH')}>
Fetch user data
</button>
),
pending: () => <Spinner />,
success: () => `Hi ${context.data.name}`,
failure: () => `Error: ${context.error.message}`,
})}
</div>
);
};
FAQs
A tiny (~700 bytes) react hook to help you write finite state machines
We found that use-tiny-state-machine demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.