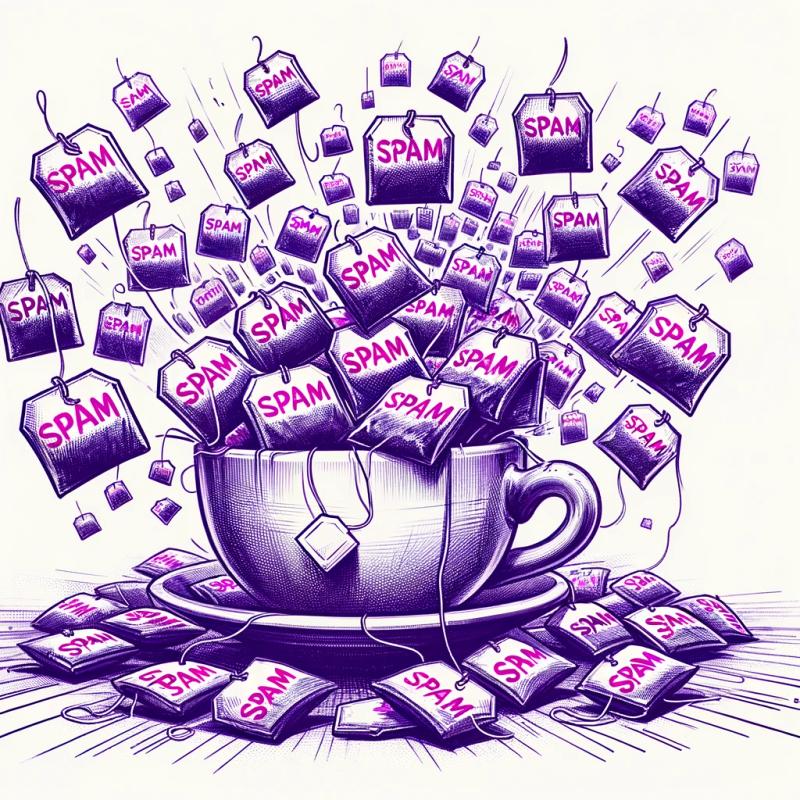
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
vantedb
Advanced tools
Readme
VanteDB is a lightweight Node.js database library that simplifies basic CRUD (Create, Read, Update, Delete) operations on JSON files. It's designed to be easy to use and suitable for small to medium-sized projects where a full-fledged database system might be overkill
const VanteDatabase = require('vantedb');
// Define a schema for a collection
const userSchema = {
username: { type: String, default: 'Kaan Karahanlı' },
age: Number,
email: { type: String, default: "hi@vante.dev" },
isAdmin: Boolean,
interests: Array,
daily: Number,
total: Number,
};
// Create a model for the "users" collection
const UserModel = VanteDatabase.model({
Collection: 'Users',
Folder: './Global/Database/',
Cluster: true,
Type: [],
}, userSchema);
// Create a model for the "settings" collection (this one is for the key chain dbs)
const SettingsModel = VanteDatabase.model({
Collection: 'Settings',
Folder: './Global/Database/',
Cluster: false,
Type: {},
}, userSchema);
// Example data
const userData = {
username: 'Kaan Karahanlı',
age: 19,
email: 'hi@vante.dev',
isAdmin: false,
interests: ['coding', 'reading', 'ertus-mom'],
};
// CRUD operations
(async () => {
try {
// Create a User
await UserModel.create(userData, { Cluster: "VANTE" });
// FIND USERS
// Find multiple users with conditions
const users = await UserModel.find({
$and: [
{ age: { $gte: 20, $lte: 30 } },
{ isAdmin: true }
]
}, { Cluster: 'VANTE' });
// Find a single user with conditions
const user = await UserModel.findOne({
$and: [
{ age: { $gte: 20, $lte: 30 } },
{ isAdmin: true },
]
}, { Cluster: 'VANTE' });
// UPDATE USER
// Update or create a user with upsert true
await UserModel.updateOne({ username: "kaanxsrd" }, { $inc: { daily: 1, total: 2 }}, { Cluster: 'VANTE', upsert: true });
// Update multiple users
await UserModel.updateMany({ age: 0 }, { $set: { age: 19 } }, { Cluster: 'VANTE' });
// DELETE USER
// Delete a single user
await UserModel.deleteOne({ username: "vantesex" }, { Cluster: 'VANTE' });
// Delete multiple users
await UserModel.deleteMany({ age: 19 }, { Cluster: 'VANTE' });
// Old key data version
// creata a new data
await settingsModel.create({}, { Cluster: 'VANTE' }); // not required
// Set a value in the settings
await SettingsModel.set("vante", "19");
// Get a value from the settings
await SettingsModel.get("vante");
// Get all settings
await SettingsModel.all();
// Push a value from an array in settings
await SettingsModel.push("interest", "sa");
// Pull a value from an array in settings
await SettingsModel.pull("interest", "sa");
// Check if a setting exists
await SettingsModel.has("vante");
// Add a value in settings
await SettingsModel.add("age", 35);
// Take a value from settings
await SettingsModel.take("age", 16);
// Delete a value from settings
await SettingsModel.delete("age");
} catch (error) {
console.error('Error performing CRUD operations:', error.message);
}
})();
Contributions are welcome! If you have any bug fixes, improvements, or new features to propose, please open an issue or submit a pull request.
VanteDB is licensed under the GPL-3.0 License. See the LICENSE file for details.
FAQs
VanteDB is a simple, lightweight database library for Node.js.
The npm package vantedb receives a total of 5 weekly downloads. As such, vantedb popularity was classified as not popular.
We found that vantedb demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.