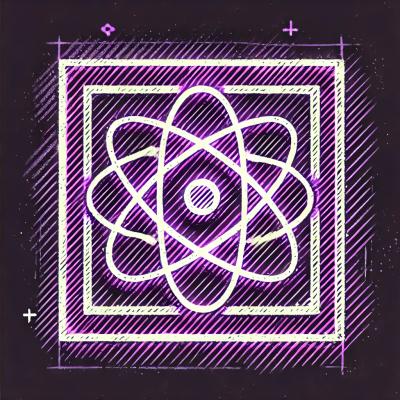
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
vis-network
Advanced tools
The vis-network npm package is a dynamic, browser-based visualization library that allows you to create, manipulate, and interact with network graphs. It is highly customizable and supports a wide range of features for visualizing complex networks.
Create a Basic Network
This code demonstrates how to create a basic network with nodes and edges using vis-network. The nodes and edges are defined using DataSet objects, and the network is rendered in a container element.
const vis = require('vis-network');
const nodes = new vis.DataSet([
{ id: 1, label: 'Node 1' },
{ id: 2, label: 'Node 2' },
{ id: 3, label: 'Node 3' },
{ id: 4, label: 'Node 4' },
{ id: 5, label: 'Node 5' }
]);
const edges = new vis.DataSet([
{ from: 1, to: 3 },
{ from: 1, to: 2 },
{ from: 2, to: 4 },
{ from: 2, to: 5 }
]);
const container = document.getElementById('mynetwork');
const data = { nodes: nodes, edges: edges };
const options = {};
const network = new vis.Network(container, data, options);
Customize Node and Edge Styles
This code sample shows how to customize the styles of nodes and edges in a vis-network graph. Nodes can have different colors, shapes, and font sizes, while edges can have different colors and styles (e.g., dashed lines).
const vis = require('vis-network');
const nodes = new vis.DataSet([
{ id: 1, label: 'Node 1', color: '#ff0000' },
{ id: 2, label: 'Node 2', shape: 'box' },
{ id: 3, label: 'Node 3', font: { size: 20 } }
]);
const edges = new vis.DataSet([
{ from: 1, to: 2, color: '#00ff00' },
{ from: 2, to: 3, dashes: true }
]);
const container = document.getElementById('mynetwork');
const data = { nodes: nodes, edges: edges };
const options = {};
const network = new vis.Network(container, data, options);
Add Interaction Events
This code demonstrates how to add interaction events to a vis-network graph. In this example, a click event is added to the network, which triggers an alert displaying the ID of the clicked node.
const vis = require('vis-network');
const nodes = new vis.DataSet([
{ id: 1, label: 'Node 1' },
{ id: 2, label: 'Node 2' }
]);
const edges = new vis.DataSet([
{ from: 1, to: 2 }
]);
const container = document.getElementById('mynetwork');
const data = { nodes: nodes, edges: edges };
const options = {};
const network = new vis.Network(container, data, options);
network.on('click', function (params) {
alert('Clicked node: ' + params.nodes);
});
Cytoscape.js is a graph theory library for visualizing and analyzing graphs. It is highly customizable and supports a wide range of graph layouts and styles. Compared to vis-network, Cytoscape.js offers more advanced graph analysis features and a larger set of layout algorithms.
Sigma.js is a JavaScript library dedicated to graph drawing. It is designed to handle large graphs efficiently and provides a variety of layout algorithms and interaction features. Sigma.js is more focused on performance and scalability compared to vis-network.
D3-force is a module of the D3.js library that provides force-directed graph layout algorithms. It allows for the creation of dynamic and interactive network visualizations. While D3-force offers powerful layout capabilities, it requires more manual setup and customization compared to vis-network.
Network is a visualization to display networks and networks consisting of nodes and edges. The visualization is easy to use and supports custom shapes, styles, colors, sizes, images, and more. The network visualization works smooth on any modern browser for up to a few thousand nodes and edges. To handle a larger amount of nodes, Network has clustering support. Network uses HTML canvas for rendering.
Install via npm:
$ npm install vis-network
A basic example on loading a Network is shown below. More examples can be found in the examples directory of the project.
<!DOCTYPE html>
<html lang="en">
<head>
<title>Network</title>
<script
type="text/javascript"
src="https://unpkg.com/vis-network/standalone/umd/vis-network.min.js"
></script>
<style type="text/css">
#mynetwork {
width: 600px;
height: 400px;
border: 1px solid lightgray;
}
</style>
</head>
<body>
<div id="mynetwork"></div>
<script type="text/javascript">
// create an array with nodes
var nodes = new vis.DataSet([
{ id: 1, label: "Node 1" },
{ id: 2, label: "Node 2" },
{ id: 3, label: "Node 3" },
{ id: 4, label: "Node 4" },
{ id: 5, label: "Node 5" },
]);
// create an array with edges
var edges = new vis.DataSet([
{ from: 1, to: 3 },
{ from: 1, to: 2 },
{ from: 2, to: 4 },
{ from: 2, to: 5 },
{ from: 3, to: 3 },
]);
// create a network
var container = document.getElementById("mynetwork");
var data = {
nodes: nodes,
edges: edges,
};
var options = {};
var network = new vis.Network(container, data, options);
</script>
</body>
</html>
To build the library from source, clone the project from github
$ git clone git://github.com/visjs/vis-network.git
The source code uses the module style of node (require and module.exports) to
organize dependencies. To install all dependencies and build the library,
run npm install
in the root of the project.
$ cd vis-network
$ npm install
Then, the project can be build running:
$ npm run build
To test the library, install the project dependencies once:
$ npm install
Then run the tests:
$ npm run test
Contributions to the vis.js library are very welcome! We can't do this alone!
Thank you to all our backers! 🙏
Support this project by becoming a sponsor. Your logo will show up here with a link to your website.
Copyright (C) 2010-2018 Almende B.V. and Contributors Copyright (c) 2018-2021 Vis.js contributors
Vis.js is dual licensed under both
and
Vis.js may be distributed under either license.
FAQs
A dynamic, browser-based visualization library.
The npm package vis-network receives a total of 89,409 weekly downloads. As such, vis-network popularity was classified as popular.
We found that vis-network demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.