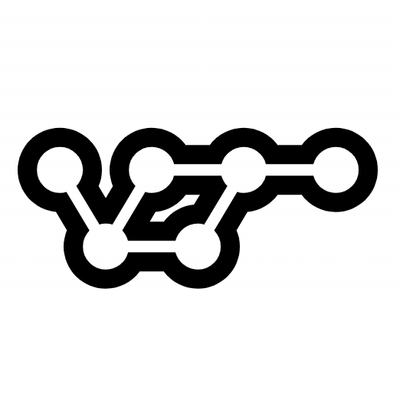
Security News
vlt Launches "reproduce": A New Tool Challenging the Limits of Package Provenance
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
vue-bootstrap-slider
Advanced tools
Easily use seiyria's Bootstrap Slider component in Vue.js
Install from npm with:
npm install --save vue-bootstrap-slider
Require or import like so for ES6:
import VueBootstrapSlider from 'vue-bootstrap-slider';
or like this for CommonJS:
var VueBootstrapSlider = require("vue-bootstrap-slider");
Next import the bootstrap-slider styles (or use less or sass):
import 'bootstrap-slider/dist/css/bootstrap-slider.css`
debounce
0
milliseconds
The events triggered by bootstrap-slider
originates from mouse-move events and can easily flood your listeners. Setting this value will debounce the events trigger. You can also just debounce or throttle your own listenerSee bootstrap-slider for a full list of options
<template>
<div>
<b-slider :value="value"/>
<p>Slider has value {{ value }}</p>
</div>
</template>
<script>
export default {
data () {
return {
value: 5
}
}
}
</script>
// range
<template>
<div>
<b-slider :value="range" range :min="config.min" :max="config.max" @change="change"/>
<p>Slider has value {{ range }}</p>
</div>
</template>
<script>
export default {
data () {
return {
config: {
min: 0,
max: 100
}
range: [ 12, 67 ]
}
},
methods: {
change ({ oldValue, newValue }) {
console.log('Slider changed from ', oldValue.join(','), ' to', newValue.join(','))
}
}
</script>
FAQs
Vue bindings for seiyria/bootstrap-slider
The npm package vue-bootstrap-slider receives a total of 356 weekly downloads. As such, vue-bootstrap-slider popularity was classified as not popular.
We found that vue-bootstrap-slider demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
vlt's new "reproduce" tool verifies npm packages against their source code, outperforming traditional provenance adoption in the JavaScript ecosystem.
Research
Security News
Socket researchers uncovered a malicious PyPI package exploiting Deezer’s API to enable coordinated music piracy through API abuse and C2 server control.
Research
The Socket Research Team discovered a malicious npm package, '@ton-wallet/create', stealing cryptocurrency wallet keys from developers and users in the TON ecosystem.