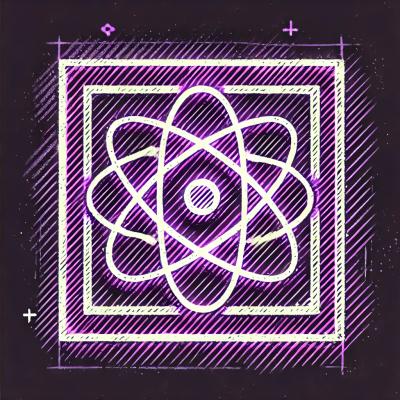
Security News
Create React App Officially Deprecated Amid React 19 Compatibility Issues
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
vue-cookie-next
Advanced tools
A simple Vue 3 plugin for handling browser cookies with typescript support
<html lang="en">
<head>
<script src="https://unpkg.com/vue/dist/vue.js"></script>
</head>
<body>
<div id="app"></div>
</body>
<script type="module">
import { VueCookieNext } from 'https://unpkg.com/vue-cookie-next@1.0.0/dist/vue-cookie-next.esm-bundler.js'
const CookieTest = {
mounted() {
this.$cookie.setCookie('username', 'user1')
console.log(this.$cookie.getCookie('username'))
},
}
Vue.createApp(CookieTest).use(VueCookieNext).mount('#app')
</script>
</html>
npm install vue-cookie-next
//or
yarn add vue-cookie-next
import { createApp } from 'vue'
import { VueCookieNext } from 'vue-cookie-next'
import App from 'App.vue'
const app = createApp(App)
app.use(VueCookieNext)
app.mount('#app')
// set default config
VueCookieNext.config({ expire: '7d' })
// set global cookie
VueCookieNext.setCookie('theme', 'default')
VueCookieNext.setCookie('hover-time', { expire: '1s' })
import { defineComponent } from 'vue'
import { useCookie } from 'vue-cookie-next'
defineComponent({
setup() {
const cookie = useCookie()
cookie.setCookie('theme', 'dark')
cookie.removeCookie('hover-time')
},
});
syntax format: [this | VueCookieNext].$cookie.[method]
VueCookieNext.config({
expire: '1d',
path: '/',
domain: '',
secure: '',
sameSite: '',
})
// default: expireTimes = 1d, path = '/', domain = '', secure = '', sameSite = 'Lax'
this.$cookie.setCookie(keyName, value, {
expire: '1d',
path: '/',
domain: '',
secure: '',
sameSite: '',
}) //return this
this.$cookie.getCookie(keyName) // return value
this.$cookie.removeCookie(keyName, {
path: '/',
domain: '',
}) // return this | false if key not found
cookie name
this.$cookie.isCookieAvailable(keyName) // return false or true
cookie name
this.$cookie.keys() // return a array string
import { VueCookieNext } from 'vue-cookie-next'
// 30 day after, expire
VueCookieNext.config({ expire: '30d' })
// set secure, only https works
VueCookieNext.config({ expire: '7d', secure: true })
// 2019-03-13 expire
VueCookieNext.config({ expire: new Date(2019, 03, 13).toUTCString() })
// 30 day after, expire, '' current path , browser default
VueCookieNext.config({ expire: 60 * 60 * 24 * 30 })
var user = {
user_id: 1,
name: 'Ben',
session: '75442486-0878-440c-9db1-a7006c25a39f',
session_start_time: new Date(),
}
this.$cookie.setCookie('user', user)
// print user name
console.log(this.$cookie.getCookieCookie('user').name)
Suppose the current time is : Sat, 11 Mar 2017 12:25:57 GMT
Following equivalence: 1 day after, expire
Support chaining sets together
// default expire time: 1 day
this.$cookie
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f')
// number + d , ignore case
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f', {
expire: '1d',
})
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f', {
expire: '1D',
})
// Base of second
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f', {
expire: 60 * 60 * 24,
})
// input a Date, + 1day
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f', {
expire: new Date(2017, 03, 12),
})
// input a date string, + 1day
.setCookie('user_session', '75442486-0878-440c-9db1-a7006c25a39f', {
expire: 'Sat, 13 Mar 2017 12:25:57 GMT',
})
this.$cookie.setCookie('default_unit_second', 'input_value', { expire: 1 }) // 1 second after, expire
this.$cookie.setCookie('default_unit_second', 'input_value', {
expire: 60 + 30,
}) // 1 minute 30 second after, expire
this.$cookie.setCookie('default_unit_second', 'input_value', {
expire: 60 * 60 * 12,
}) // 12 hour after, expire
this.$cookie.setCookie('default_unit_second', 'input_value', {
expire: 60 * 60 * 24 * 30,
}) // 1 month after, expire
this.$cookie.setCookie('default_unit_second', 'input_value', { expire: 0 }) // end of session - use 0 or "0"!
Unit | full name |
---|---|
y | year |
m | month |
d | day |
h | hour |
min | minute |
s | second |
Unit Names Ignore Case
not support the combination
not support the double value
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '60s',
}) // 60 second after, expire
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '30MIN',
}) // 30 minute after, expire, ignore case
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '24d',
}) // 24 day after, expire
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '4m',
}) // 4 month after, expire
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '16h',
}) // 16 hour after, expire
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: '3y',
}) // 3 year after, expire
// input date string
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: new Date(2017, 3, 13).toUTCString(),
})
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: 'Sat, 13 Mar 2017 12:25:57 GMT ',
})
var date = new Date()
date.setDate(date.getDate() + 1)
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: date,
})
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', {
expire: Infinity,
}) // never expire
// never expire , only -1,Other negative Numbers are invalid
this.$cookie.setCookie('token', 'GH1.1.1689020474.1484362313', { expire: -1 })
this.$cookie.setCookie('token', 'value') // domain.com and *.doamin.com are readable
this.$cookie.removeCookie('token') // remove token of domain.com and *.doamin.com
this.$cookie.setCookie('token', value, { domain: 'domain.com' }) // only domain.com are readable
this.$cookie.removeCookie('token', { domain: 'domain.com' }) // remove token of domain.com
// set path
this.$cookie.setCookie('use_path_argument', 'value', {
expire: '1d',
path: '/app',
})
// set domain
this.$cookie.setCookie('use_path_argument', 'value', { domain: 'domain.com' }) // default 1 day after,expire
// set secure
this.$cookie.setCookie('use_path_argument', 'value', {
secure: true,
})
// set sameSite - should be one of `None`, `Strict` or `Lax`. Read more https://web.dev/samesite-cookies-explained/
this.$cookie.setCookie('use_path_argument', 'value', { sameSite: 'Lax' })
// check a cookie exist
this.$cookie.isCookieAvailable("user_session")
// get a cookie
this.$cookie.getCookie("user_session");
// remove a cookie
this.$cookie.removeCookie("user_session");
// get all cookie key names, line shows
this.$cookie.keys().join("\n");
// remove all cookie
this.$cookie.keys().forEach(cookie => this.$cookie.removeCookie(cookie))
// vue-cookie-next global
[this | VueCookieNext].$cookie.[method]
$cookie key names Cannot be set to ['expires','max-age','path','domain','secure','SameSite']
This project is heavily inspired by the following awesome projects.
Thanks!
FAQs
A simple Vue.js plugin for handling browser cookies
The npm package vue-cookie-next receives a total of 4,148 weekly downloads. As such, vue-cookie-next popularity was classified as popular.
We found that vue-cookie-next demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Create React App is officially deprecated due to React 19 issues and lack of maintenance—developers should switch to Vite or other modern alternatives.
Security News
Oracle seeks to dismiss fraud claims in the JavaScript trademark dispute, delaying the case and avoiding questions about its right to the name.
Security News
The Linux Foundation is warning open source developers that compliance with global sanctions is mandatory, highlighting legal risks and restrictions on contributions.