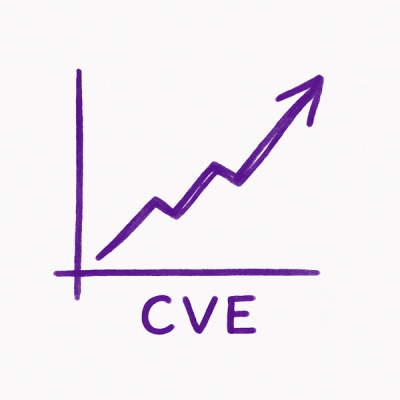
Security News
New CVE Forecasting Tool Predicts 47,000 Disclosures in 2025
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
vue-jest is a Jest transformer for Vue single-file components (SFCs). It allows you to use Jest to test Vue components by transforming the .vue files into a format that Jest can understand.
Transform Vue SFCs
This feature allows Jest to transform Vue single-file components (.vue files) so that they can be tested. The code sample shows how to configure Jest to use vue-jest for transforming .vue files.
module.exports = { transform: { '^.+\.vue$': 'vue-jest' } };
Mocking Vue Components
This feature allows you to mock Vue components and their dependencies during testing. The code sample demonstrates how to use vue-jest with @vue/test-utils to shallow mount a component and mock a translation function.
import { shallowMount } from '@vue/test-utils';
import MyComponent from '@/components/MyComponent.vue';
test('renders a message', () => {
const wrapper = shallowMount(MyComponent, {
mocks: {
$t: msg => msg
}
});
expect(wrapper.text()).toContain('Hello World');
});
Snapshot Testing
This feature allows you to perform snapshot testing on Vue components. The code sample shows how to use vue-jest with @vue/test-utils to create a snapshot of a component's rendered HTML and compare it to a saved snapshot.
import { shallowMount } from '@vue/test-utils';
import MyComponent from '@/components/MyComponent.vue';
test('matches snapshot', () => {
const wrapper = shallowMount(MyComponent);
expect(wrapper.html()).toMatchSnapshot();
});
vue-test-utils is the official testing utility library for Vue.js. It provides methods to mount and interact with Vue components in tests. While vue-jest focuses on transforming .vue files for Jest, vue-test-utils provides a more comprehensive set of utilities for testing Vue components.
jest-vue-preprocessor is another Jest transformer for Vue single-file components. It is similar to vue-jest in that it transforms .vue files for Jest, but it is less commonly used and lacks some of the additional features and community support that vue-jest offers.
babel-jest is a Jest transformer that uses Babel to transform JavaScript files. While it is not specific to Vue, it can be used in conjunction with other tools to transform Vue components. It is more general-purpose compared to vue-jest, which is specifically designed for Vue SFCs.
Jest Vue transformer with source map support
NOTE: This is documentation for
vue-jest@3.x
. View the vue-jest@2.x documentation
npm install --save-dev vue-jest
To define vue-jest
as a transformer for your .vue
files, map them to the vue-jest
module:
{
"jest": {
"transform": {
"^.+\\.vue$": "vue-jest"
}
}
A full config will look like this.
{
"jest": {
"moduleFileExtensions": [
"js",
"json",
"vue"
],
"transform": {
"^.+\\.js$": "babel-jest",
"^.+\\.vue$": "vue-jest"
}
}
}
If you're on a version of Jest older than 22.4.0, you need to set mapCoverage
to true
in order to use source maps.
Example repositories testing Vue components with jest and vue-jest:
vue-jest compiles the script and template of SFCs into a JavaScript file that Jest can run. Currently, SCSS, SASS and Stylus are the only style languages that are compiled.
lang="ts"
, lang="typescript"
)lang="coffee"
, lang="coffeescript"
)You can change the behavior of vue-jest
by using jest.globals
.
Tip: Need programmatic configuration? Use the --config option in Jest CLI, and export a
.js
file
Provide babelConfig
in one of the following formats:
<Boolean>
<Object>
<String>
true
- Enable Babel processing. vue-jest
will try to find Babel configuration using find-babel-config.This is the default behavior if babelConfig is not defined.
false
- Skip Babel processing entirely:{
"jest": {
"globals": {
"vue-jest": {
"babelConfig": false
}
}
}
}
Provide inline Babel options:
{
"jest": {
"globals": {
"vue-jest": {
"babelConfig": {
"presets": [
[
"env",
{
"useBuiltIns": "entry",
"shippedProposals": true
}
]
],
"plugins": [
"syntax-dynamic-import"
],
"env": {
"test": {
"plugins": [
"dynamic-import-node"
]
}
}
}
}
}
}
}
If a string is provided, it will be an assumed path to a babel configuration file (e.g. .babelrc
, .babelrc.js
).
{
"jest": {
"globals": {
"vue-jest": {
"babelConfig": "path/to/.babelrc.js"
}
}
}
}
To use the Config Function API, use inline options instead. i.e.:
{
"jest": {
"globals": {
"vue-jest": {
"babelConfig": {
"configFile": "path/to/babel.config.js"
}
}
}
}
}
Provide tsConfig
in one of the following formats:
<Boolean>
<Object>
<String>
true
- Process TypeScript files using custom configuration. vue-jest
will try to find TypeScript configuration using tsconfig.loadSync.This is the default behavior if tsConfig is not defined.
false
- Process TypeScript files using the default configuration provided by vue-jest.Provide inline TypeScript compiler options:
{
"jest": {
"globals": {
"vue-jest": {
"tsConfig": {
"importHelpers": true
}
}
}
}
}
If a string is provided, it will be an assumed path to a TypeScript configuration file:
{
"jest": {
"globals": {
"vue-jest": {
"tsConfig": "path/to/tsconfig.json"
}
}
}
}
pug (lang="pug"
)
{
"jest": {
"globals": {
"vue-jest": {
"pug": {
"basedir": "mybasedir"
}
}
}
}
}
jade (lang="jade"
)
haml (lang="haml"
)
lang="stylus"
, lang="styl"
)lang="sass"
)
lang="scss"
)
The SCSS compiler supports jest's moduleNameMapper which is the suggested way of dealing with Webpack aliases.
To import globally included files (ie. variables, mixins, etc.), include them in the Jest configuration at jest.globals['vue-jest'].resources.scss
:
{
"jest": {
"globals": {
"vue-jest": {
"resources": {
"scss": [
"./node_modules/package/_mixins.scss",
"./src/assets/css/globals.scss"
]
}
}
}
}
}
lang="pcss"
, lang="postcss"
)experimentalCSSCompile
: Boolean
Default true. Turn off CSS compilation
hideStyleWarn
: Boolean
Default false. Hide warnings about CSS compilation
resources
:
{
"jest": {
"globals": {
"vue-jest": {
"hideStyleWarn": true,
"experimentalCSSCompile": true
}
}
}
}
FAQs
Jest Vue transform
The npm package vue-jest receives a total of 348,318 weekly downloads. As such, vue-jest popularity was classified as popular.
We found that vue-jest demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 4 open source maintainers collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
CVEForecast.org uses machine learning to project a record-breaking surge in vulnerability disclosures in 2025.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.