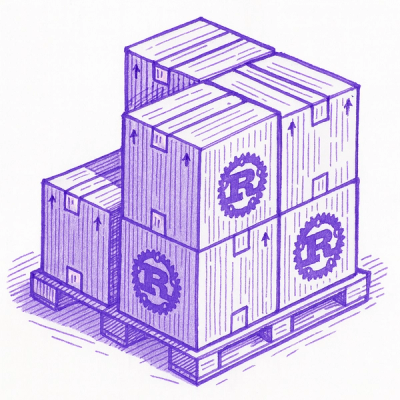
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
vue-onboarding-tour
Advanced tools
**VueOnboardingTour** is a Vue.js component that creates guided, step-by-step onboarding tours to help users navigate your app intuitively.
VueOnboardingTour is a Vue.js component that creates guided, step-by-step onboarding tours to help users navigate your app intuitively.
Using npm:
npm install @acTechWorld/vue-onboarding-tour
Or, using yarn:
yarn add @acTechWorld/vue-onboarding-tour
After installation, import vue-onboarding-tour in your main JavaScript file and register it as a Vue plugin.
// main.js
import Vue from 'vue'
import App from './App.vue'
import VueOnboardingTour from '@acTechWorld/vue-onboarding-tour'
Vue.use(VueOnboardingTour)
new Vue({
render: (h) => h(App),
}).$mount('#app')
// main.js
import { createApp } from 'vue'
import App from './App.vue'
import VueOnboardingTour from '@acTechWorld/vue-onboarding-tour'
const app = createApp(App)
app.use(VueOnboardingTour)
app.mount('#app')
The VueOnboardingTour
component allows for creating an interactive, step-by-step tour within a Vue application. Here is a detailed breakdown of the props to customize your tour.
tourId
Type: String | Number
Required: true
Description: A unique identifier for the tour. If cookieStorage
is enabled, this ID is used to store a cookie, ensuring the tour is only shown once per user.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" />
defaultTemplate
Type: Boolean
Default: true
Description: Controls whether the default tour template is used. Set to false
to design a custom template for the tour steps.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" :defaultTemplate="false" />
overlay
Type: Boolean
Default: true
Description: Enables or disables the overlay mask that highlights the area around the target element during each step.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" :overlay="false" />
startEvent
String
undefined
<VueOnboardingTour :tourId="123" :steps="steps" startEvent="showTour" />
To start the tour from a parent component, you can emit a custom event and pass the event name to the startEvent
prop. Below is an example of how to trigger the tour using the custom event.
Parent Component (ParentComponent.vue):
<template>
<div>
<button @click="startTour">Start Tour</button>
<VueOnboardingTour
:tourId="123"
:steps="steps"
startEvent="showTour"
labelTerminate="Finish Tour"
/>
<div id="step1"></div>
<div id="step2"></div>
</div>
</template>
<script lang="ts">
export default {
data() {
return {
steps: [
{
target: '#step1',
title: 'Welcome',
description: 'This is the first step of the tour.',
},
{
target: '#step2',
title: 'Features',
description: 'This is where you can find features overview.',
},
],
}
},
methods: {
startTour() {
// Emit the custom event to start the tour
window.dispatchEvent(new CustomEvent('showTour'))
},
},
}
</script>
In this example, the ParentComponent
contains a button that triggers the startTour
method when clicked. This method emits the showTour
event to start the onboarding tour in the VueOnboardingTour
component.
scrollableContainerSelector
Type: String
Default: undefined
Description: A CSS selector for a scrollable container within which the target elements are located. If set, the component will scroll this container to bring target elements into view instead of scrolling the entire page.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" scrollableContainerSelector="#main-container" />
cookieStorage
Type: Boolean
Default: false
Description: Determines whether to store a cookie once the tour is completed, so it only shows once per user.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" :cookieStorage="true" />
endDate
Type: Date
Default: undefined
Description: The endDate
prop allows you to set an expiration date for the tour. After this date, the tour will not be shown again, even if the cookie is not present. Additionally, all cookies set by this component (such as those used for cookieStorage
) will have an expiration date equal to this endDate
. As a result, these cookies will be automatically cleared from the user's browser once the expiration date is reached.
Usage:
<VueOnboardingTour
:tourId="123"
:steps="steps"
:cookieStorage="true"
:endDate="new Date('2025-01-01')"
/>
labelTerminate
Type: String
Default: 'close'
Description: The label for the button or text used to close the tour at the last step. Customize this label to suit your app's language or tone.
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" labelTerminate="Finish" />
steps
Type: Array
Required: true
Description: An array of objects defining each step in the tour. Each step object has the following fields:
target
(String): A CSS selector for the element to highlight.title
(String, optional): The title text for the step.description
(String, optional): A description for the step.tag
(String, optional): Additional text or label to be shown in the step (e.g., a category or type label).beforeScript
(Function, optional): A function to be executed before showing the step.afterScript
(Function, optional): A function to be executed after displaying the step.Example Steps Array:
const steps = [
{
target: '#step1',
title: 'Welcome',
description: 'This is the starting point of the tour.',
tag: 'Step 1',
beforeScript: () => console.log('Preparing for Step 1'),
afterScript: () => console.log('Completed Step 1'),
},
{
target: '#step2',
title: 'Feature Overview',
description: 'Here you can learn about the main features of our app.',
tag: 'Step 2',
},
]
Usage:
<VueOnboardingTour :tourId="123" :steps="steps" />
startTour()
Purpose:
Starts the onboarding tour programmatically.
Usage:
Trigger the tour when needed,
e.g., after a button click or other user action.
this.$refs.onboardingTour.startTour()
endTour()
Purpose:
Ends the onboarding tour programmatically.
Usage:
Call this method to finish the tour prematurely.
this.$refs.onboardingTour.endTour()
goNextStep()
Purpose:
Moves to the next step of the tour.
Usage:
Use this method to skip to the next tour step programmatically.
this.$refs.onboardingTour.goNextStep()
goPreviousStep()
Purpose:
Moves to the previous step of the tour.
Usage:
Use this method to go back to the previous step in the tour.
this.$refs.onboardingTour.goPreviousStep()
setStep(index)
Purpose:
Sets the tour to a specific step based on its index.
Usage:
Programmatically jump to a specific step.
this.$refs.onboardingTour.setStep(2) // Jumps to the third step
The cookieStorage
prop allows you to persist the state of the tour using cookies in the user's browser. When enabled, it ensures that the onboarding tour is only shown to the user once. After the user completes the tour, a cookie is set on their device, marking the tour as completed. The next time the user visits the site, the tour will not be displayed unless the cookie expires or is cleared.
cookieStorage
is enabled, a cookie with the name vue_onboarding_tour_{tourId}
is created. The value of the cookie is a JSON object that stores the date the tour was completed.endDate
prop allows you to set an expiration date for the tour. After this date, the tour will not be shown again, even if the cookie is not present. Additionally, all cookies set by this component (such as those used for cookieStorage
) will have an expiration date equal to this endDate
. As a result, these cookies will be automatically cleared from the user's browser once the expiration date is reached.The cookie stored is a JSON object with the following format:
{
"tourId": "123",
"completedAt": "2024-11-12T12:00:00Z"
}
Where:
tourId
: The unique ID of the tour (matching the tourId
prop).completedAt
: The date and time when the tour was completed, stored in ISO 8601 format.To enable cookie storage and set an expiration date, you can use the following configuration:
<VueOnboardingTour
:tourId="123"
:steps="steps"
:cookieStorage="true"
:endDate="new Date('2025-01-01')"
/>
In this example:
cookieStorage
prop is set to true
, so a cookie will be stored when the tour is completed.endDate
is set to January 1, 2025. After this date, the cookie will be considered expired, and the tour will no longer appear, regardless of the user's previous completion.FAQs
**VueOnboardingTour** is a Vue.js component that creates guided, step-by-step onboarding tours to help users navigate your app intuitively.
The npm package vue-onboarding-tour receives a total of 244 weekly downloads. As such, vue-onboarding-tour popularity was classified as not popular.
We found that vue-onboarding-tour demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.