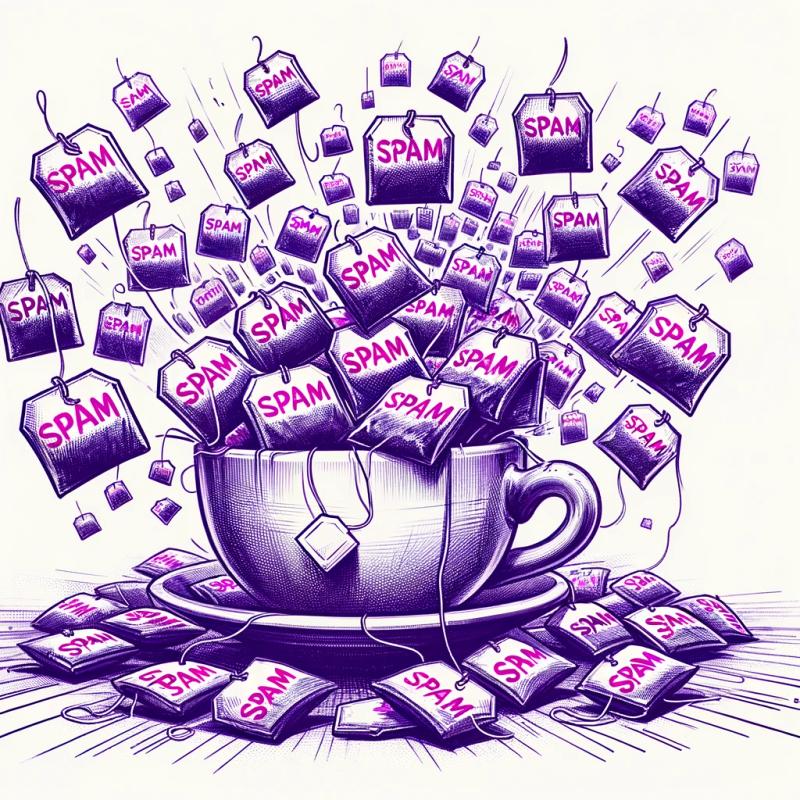
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
vue3-drr-grid-layout-2
Advanced tools
Readme
Grid layout for vue 3 with draggable, resize, responsive events
Module use source code from VueGridLayout
Rewrote to TypeScript, Composition API and migrated to Vue3
import { createApp } from 'vue'
import App from './App.vue'
import GridLayout from 'vue3-drr-grid-layout'
import 'vue3-drr-grid-layout/dist/style.css'
const app = createApp(App)
app.use(GridLayout)
app.mount('#app')
<template>
<grid-layout
v-model:layout="layout"
:col-num="12"
:row-height="30"
@resize="resize"
@move="move"
@moved="moved"
>
<template #item="{ item }">
{{ item.i }}
</template>
</grid-layout>
</template>
<script>
export default {
name: 'App',
data () {
return {
layout: [
{ x: 0, y: 0, w: 2, h: 2, i: 0 },
{ x: 2, y: 0, w: 2, h: 4, i: 1 },
{ x: 4, y: 0, w: 2, h: 5, i: 2 },
{ x: 6, y: 0, w: 2, h: 3, i: 3 },
{ x: 8, y: 0, w: 2, h: 3, i: 4 },
{ x: 8, y: 0, w: 2, h: 3, i: 5 },
{ x: 0, y: 5, w: 2, h: 5, i: 6 },
{ x: 2, y: 5, w: 2, h: 5, i: 7 },
{ x: 4, y: 5, w: 2, h: 5, i: 8 },
{ x: 6, y: 3, w: 2, h: 4, i: 9 }
]
}
}
}
</script>
or
<template>
<grid-layout
v-model:layout="layout"
:col-num="12"
:row-height="30"
>
<template #default="{ gridItemProps }">
<!-- | gridItemProps props from GridLayout | -->
<!--breakpointCols: props.cols-->
<!--colNum: props.colNum-->
<!--containerWidth: width.value-->
<!--isDraggable: props.isDraggable-->
<!--isResizable: props.isResizable-->
<!--lastBreakpoint: lastBreakpoint.value-->
<!--margin: props.margin-->
<!--maxRows: props.maxRows-->
<!--responsive: props.responsive-->
<!--rowHeight: props.rowHeight-->
<!--useCssTransforms: props.useCssTransforms-->
<!--width: width.value-->
<grid-item
v-for="item in layout"
:key="item.i"
v-bind="gridItemProps"
:x="item.x"
:y="item.y"
:w="item.w"
:h="item.h"
:i="item.i"
@resize="resize"
@move="move"
@moved="moved"
>
{{ item.i }}
</grid-item>
</template>
</grid-layout>
</template>
<script>
export default {
name: 'App',
data () {
return {
layout: [
{ x: 0, y: 0, w: 2, h: 2, i: 0 },
{ x: 2, y: 0, w: 2, h: 4, i: 1 },
{ x: 4, y: 0, w: 2, h: 5, i: 2 },
{ x: 6, y: 0, w: 2, h: 3, i: 3 },
{ x: 8, y: 0, w: 2, h: 3, i: 4 },
{ x: 8, y: 0, w: 2, h: 3, i: 5 },
{ x: 0, y: 5, w: 2, h: 5, i: 6 },
{ x: 2, y: 5, w: 2, h: 5, i: 7 },
{ x: 4, y: 5, w: 2, h: 5, i: 8 },
{ x: 6, y: 3, w: 2, h: 4, i: 9 }
]
}
}
}
</script>
//
// Required props
//
// This is grid layout for not responsive pages
layout: {
x: number
y: number
w: number
h: number
i: number
isDraggable?: boolean
isResizable?: boolean
maxH?: number
maxW?: number
minH?: number
minW?: number
moved?: boolean
static?: boolea
}
// Number of columns
colNum: number
//
// Optional props
//
autoSize: boolean = true
isDraggable: boolean = true
isDraggable: boolean = true
margin: [number, number] = [10, 10]
maxRows: number = Infinity
preventCollision: boolean = true
rowHeight: number = 150
useCssTransforms: boolean = true
verticalCompact: boolean = true
// swaps grid items standing next to each other when moving horizontally
horizontalShift: boolean = false
// To enable responsive mode, the responsive prop must be true.
responsive: boolean = false
// 6 display widths
// Window witch > lg component use layout prop
breakpoints: object = { lg: 1200, md: 996, sm: 768, xs: 480, xxs: 0 }
// colNum by display width
cols: object = { lg: 12, md: 10, sm: 6, xs: 4, xxs: 2 }
// Responsive layout is a { [keyof breakpoints]: layout }
// { lg: layout, md: layout, sm: layout, xs: layout, xss: layout }
responsiveLayouts: object = {}
// To enable intersection observer mode, the useObserver prop must be true.
useObserver: boolean = false
// Intersection observer config { ...propsConfig, ...defaultConfig }
intersectionObserverConfig: object = { root: null, rootMargin: '8px', threshold: 0.40 }
{
i: number // grid item index
h: number // grid item height from propss
w: number // grid item width from props
height: number // grid item height
width: number // grid item width
}
i: number // grid item index
h: number // grid item height from propss
w: number // grid item width from props
newHeight: number // grid item height
newWidth: number // grid item width
i: number // grid item index
h: number // grid item height from propss
w: number // grid item width from props
newHeight: number // grid item height
newWidth: number // grid item width
i: number // grid item index
x: number // grid item x position
y: number // grid item y position
i: number // grid item index
x: number // grid item x position
y: number // grid item y position
layout: Layout // see props
layout: Layout // see props
newBreakpoint: Breakpoints // see props
layout: Layout // see props
layout: Layout // see props
observeItems: number[] // grid items indexes
unobserveItems: number[] // grid items indexes
FAQs
Vue3 grid layout with resize, drag and responsive
The npm package vue3-drr-grid-layout-2 receives a total of 0 weekly downloads. As such, vue3-drr-grid-layout-2 popularity was classified as not popular.
We found that vue3-drr-grid-layout-2 demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.