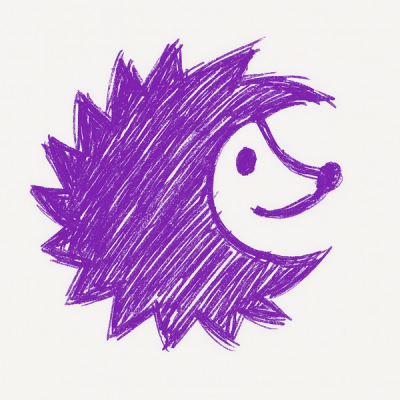
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
vue3-form-validation
Advanced tools
Easy to use Form Validation for Vue 3
Note this is still WIP! But a working prototype is currently available and can be used.
npm i vue3-form-validation
Validation is async and is utilising Promise.allSettled
, which has not yet reached cross-browser stability.
Example usage can be found in this Code Sandbox.
Currently this package exports two functions provideBaseForm
and useBaseForm
, plus some type definitions when using TypeScript (see vue3-form-validation/composable/useForm.ts
for all exports).
Both functions are meant to be used inside of base form components. They make use of provide
and inject
to communicate data, which means that provideBaseForm
has to be called in a component that is higher up in the tree than useBaseForm
.
SomeForm.vue
<template>
<BaseForm> <-- provideBaseForm()
<BaseInput /> <-- useBaseForm()
<BaseSelect /> <-- useBaseForm()
</BaseForm>
</template>
const { onSubmit } = provideBaseForm();
provideBaseForm
exposes the following methods:Signature | Returns | Description |
---|---|---|
onSubmit() | Promise<boolean> | Call this when submitting the form. It validates all fields and returns a boolean value that indicates whether or not there are errors. |
const { uid, onBlur, errors, validating } = useBaseForm(modelValue, rules);
useBaseForm
takes the following parameters:Parameters | Type | Required | Description |
---|---|---|---|
modelValue | Ref<unknown> | true | A ref that holds the current form field value. |
rules | Ref<(function | object)[]> | true | A ref with an array of rules. |
useBaseForm
exposes the following state:State | Type | Description |
---|---|---|
uid | number | Unique id of the form field. |
errors | Ref<string[]> | Validation errors. |
validating | Ref<boolean> | True while atleast one rule is validating. |
useBaseForm
exposes the following methods:Signature | Returns | Description |
---|---|---|
onBlur() | void | Call this when the form field blur event fires. |
Rules are async
functions that should return a string
when the validation fails. They can be written purely as a function or together with a key
property in an object.
Typing:
type SimpleRule = (value: unknown) => Promise<unknown>;
type KeyedRule = { key: string; rule: SimpleRule };
type Rule = SimpleRule | KeyedRule;
For now rules are meant to be passed as props
to your base form components, where you then use them as a parameter for useBaseForm
. KeyedRules that share the same key will be executed together, this can be useful in a situation where rules are dependent on another. For example the Password
and Repeat password
fields in a Login Form.
Rules will always be called with the latest modelValue
, to determine if a call should result in an error, it will check if the rule's return value is of type string
.
vue3-form-validation/Form.ts
let error: unknown;
// ...
error = await rule(formField.modelValue);
// ...
if (typeof error === 'string') {
// report validation error
}
// ...
This allows you to write many rules in one line:
const required = async value => !value && "This field is required";
const min = async value => value.length > 3 || "This field has to be longer than 3 characters";
const max = async value => value.length < 7 || "This field is too long (maximum is 6 characters)";
Of course you can also return a Promise
directly or perform network requests, for example checking if a username already exists in the database.
FAQs
Vue composition function for form validation
The npm package vue3-form-validation receives a total of 315 weekly downloads. As such, vue3-form-validation popularity was classified as not popular.
We found that vue3-form-validation demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.