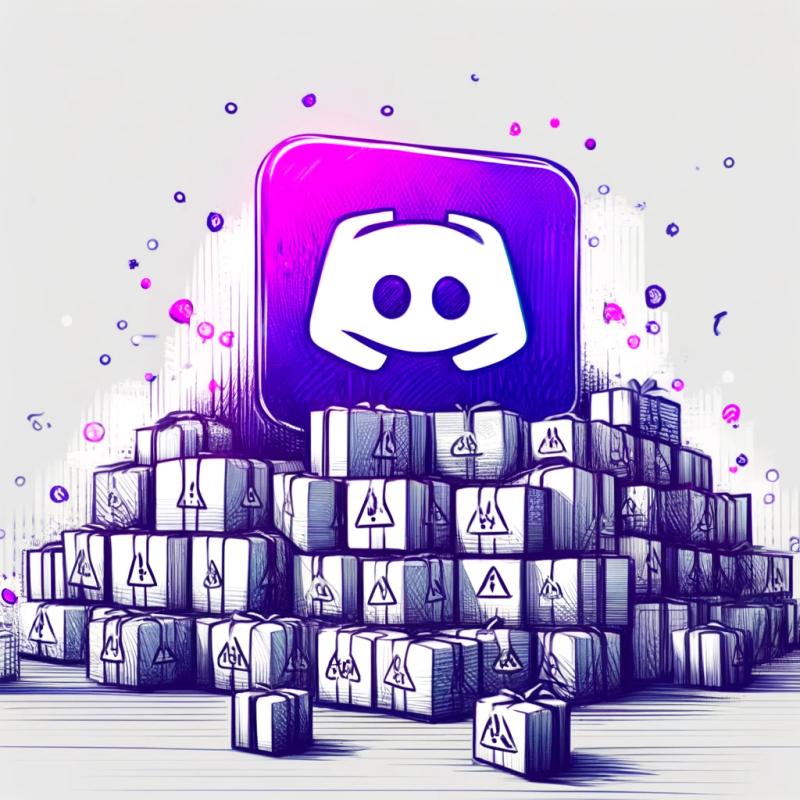
Research
Recent Trends in Malicious Packages Targeting Discord
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
w-comor-socketio
Advanced tools
Readme
A http and websocket communicator in nodejs and browser. Mapping functions from nodejs to other end points, like a simple RPC.
To view documentation or get support, visit docs.
w-comor-socketio
includes 2 parts:
w-comor-socketio-server
: for nodejs serverw-comor-socketio-client
: for nodejs and browser clientNote:
w-comor-socketio-server
is mainly dependent on@hapi/hapi
,@hapi/inert
andsocket.io
.
Note:
w-comor-socketio-client
is mainly dependent onsocket.io-client
.
npm i w-comor-socketio
w-comor-socketio-server
:Link: [dev source code]
import WComorSocketioServer from 'w-comor-socketio/dist/w-comor-socketio-server.umd.js'
function random(min, max) {
return Math.floor(Math.random() * max) + min
}
let opt = {
port: 8080,
authenticate: function(token) {
//authenticate user by token
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(true)
}, 1000)
})
},
filterFuncs: function(token, funcs) {
//resolve funcs by authenticating user
return new Promise(function(resolve, reject) {
funcs = funcs.filter(function(v) {
return v.indexOf('Hide') < 0
})
resolve(funcs)
})
},
onClientChange: function(clients, opt) {
console.log(`Server[port:${opt.port}] now clients: ${clients.length}`)
},
funcs: {
'group.plus': function({ p1, p2 }) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(p1 * p2)
}, random(100, 3000))
})
},
'group.div': function({ p1, p2 }) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(p1 / p2)
}, random(100, 3000))
})
},
'add': function({ p1, p2 }) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(p1 + p2)
}, random(100, 3000))
})
},
'addHide': function({ p1, p2 }) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(p1 + p2)
}, random(100, 3000))
})
},
'minu': function({ p1, p2 }) {
return new Promise(function(resolve, reject) {
setTimeout(function() {
resolve(p1 - p2)
}, random(100, 3000))
})
},
},
}
new WComorSocketioServer(opt)
w-comor-socketio-client
:Link: [dev source code]
import WComorSocketioClient from 'w-comor-socketio/dist/w-comor-socketio-client.umd.js'
//opt
let opt = {
url: 'http://localhost:8080',
token: '*',
open: function() {
console.log('client nodejs: open')
},
close: function() {
console.log('client nodejs: close')
},
error: function(err) {
console.log('client nodejs: error:', err)
},
reconn: function() {
console.log('client nodejs: reconn')
},
}
//WComorSocketioClient
new WComorSocketioClient(opt)
.then(function(wo) {
console.log('client nodejs: funcs: ', wo)
function core(ps) {
wo.group.plus(ps)
.then(function(r) {
console.log('client nodejs: plus(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client nodejs: plus: catch: ', err)
})
wo.group.div(ps)
.then(function(r) {
console.log('client nodejs: div(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client nodejs: div: catch: ', err)
})
wo.add(ps)
.then(function(r) {
console.log('client nodejs: add(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client nodejs: add: catch: ', err)
})
wo.minu(ps)
.then(function(r) {
console.log('client nodejs: minu(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client nodejs: minu: catch: ', err)
})
}
let i = 100
setInterval(function() {
i += 1
core({
p1: i,
p2: 10,
})
}, 1000)
})
.catch(function(err) {
console.log('client nodejs: catch', err)
})
Note:
w-comor-socketio-client
does't depend on any package in browser.
[Optional] Add script with nomodule for IE11.
<script nomodule src="https://cdn.jsdelivr.net/npm/@babel/polyfill/dist/polyfill.min.js"></script>
[Necessary] Add script for w-comor-socketio-client.
<script src="https://cdn.jsdelivr.net/npm/w-comor-socketio@1.0.18/dist/w-comor-socketio-client.umd.js"></script>
w-comor-socketio-client
:Link: [dev source code]
//opt
let opt = {
url: 'http://localhost:8080',
token: '*',
open: function() {
console.log('client web: open')
},
close: function() {
console.log('client web: close')
},
error: function(err) {
console.log('client web: error:', err)
},
reconn: function() {
console.log('client web: reconn')
},
}
//WComorSocketioClient
let WComorSocketioClient = window['w-comor-socketio-client']
new WComorSocketioClient(opt)
.then(function(wo) {
console.log('client web: funcs: ', wo)
function core(ps) {
wo.group.plus(ps)
.then(function(r) {
console.log('client web: plus(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client web: plus: catch: ', err)
})
wo.group.div(ps)
.then(function(r) {
console.log('client web: div(' + JSON.stringify(ps) + ')=' + r)
})
.catch(function(err) {
console.log('client web: div: catch: ', err)
})
wo.add(ps)
.then(function(r) {
console.log('client web: add('+JSON.stringify(ps)+')='+r)
})
.catch(function(err) {
console.log('client web: add: catch: ', err)
})
wo.minu(ps)
.then(function(r) {
console.log('client web: minu('+JSON.stringify(ps)+')='+r)
})
.catch(function(err) {
console.log('client web: minu: catch: ', err)
})
}
let i = 100
setInterval(function() {
i += 1
core({
p1: i,
p2: 10,
})
}, 1000)
})
.catch(function(err) {
console.log('client web: catch: ', err)
})
FAQs
A http and websocket communicator in nodejs and browser. Mapping functions from nodejs to other end points, like a simple RPC.
We found that w-comor-socketio demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
The Socket research team breaks down a sampling of malicious packages that download and execute files, among other suspicious behaviors, targeting the popular Discord platform.
Security News
Socket CEO Feross Aboukhadijeh joins a16z partners to discuss how modern, sophisticated supply chain attacks require AI-driven defenses and explore the challenges and solutions in leveraging AI for threat detection early in the development life cycle.
Security News
NIST's new AI Risk Management Framework aims to enhance the security and reliability of generative AI systems and address the unique challenges of malicious AI exploits.