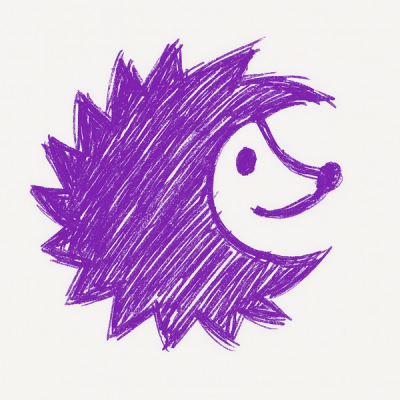
Security News
Browserslist-rs Gets Major Refactor, Cutting Binary Size by Over 1MB
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
web-api-hooks
Advanced tools
Essential set of React Hooks for convenient Web API consumption.
Being part of the @kripod/react-hooks project, this package is:
After installing the package, import individual hooks as shown below:
import { useGeolocation, useLocalStorage } from 'web-api-hooks';
š Explore the API with working examples
Tracks color scheme preference of the user.
function Component() {
const preferDarkMode = useColorSchemePreference() === 'dark';
// ...
}
Returns ("light"
| "dark"
) Preferred color scheme.
Tracks acceleration and rotation rate of the device.
function Component() {
const { acceleration, rotationRate, interval } = useDeviceMotion();
// ...
}
Returns EventArgs<DeviceMotionEvent> Own properties of the last corresponding event.
Tracks physical orientation of the device.
function Component() {
const { alpha, beta, gamma } = useDeviceOrientation();
// ...
}
Returns EventArgs<DeviceOrientationEvent> Own properties of the last corresponding event.
Tracks loading state of the page.
function Component() {
const documentReadiness = useDocumentReadiness();
if (documentReadiness === 'interactive') {
// You may interact with any element of the document from now
}
// ...
}
Returns DocumentReadyState Readiness of the document
, which is 'loading'
by default.
Tracks visibility of the page.
function Component() {
const documentVisibility = useDocumentVisibility();
if (documentVisibility === 'hidden') {
// Reduce resource utilization to aid background page performance
}
// ...
}
Returns VisibilityState Visibility state of the document
, which is 'visible'
by default.
Tracks focus state of an element.
function Component() {
const [isFocused, bindFocus] = useFocus();
// ...
return <ElementToObserve {...bindFocus} />;
}
Returns [boolean, Readonly<{onFocus: function (): void, onBlur: function (): void}>] Whether the element has focus, and props to be spread over the element under observation.
Tracks geolocation of the device.
options
PositionOptions? Additional watching options.errorCallback
function (error: PositionError): void? Method to execute in case of an error, e.g. when the user denies location sharing permissions.function Component() {
const geolocation = useGeolocation();
if (geolocation) {
const { coords } = geolocation;
}
// ...
}
Returns (Position | undefined) Locational data, or undefined
when unavailable.
Tracks hover state of an element.
disallowTouch
boolean Determines whether touch gestures should be ignored. (optional, default false
)function Component() {
const [isHovered, bindHover] = useHover();
// ...
return <ElementToObserve {...bindHover} />;
}
Returns [boolean, Readonly<{onMouseEnter: function (): void, onMouseLeave: function (): void, onTouchStart: function (): void, onTouchEnd: function (): void}>] Whether the element is hovered, and props to be spread over the element under observation.
Tracks language preferences of the user.
function Component() {
const preferredLanguages = useLanguagePreferences();
// ...
}
Returns ReadonlyArray<string> An array of BCP 47 language tags, ordered by preference with the most preferred language first.
Tracks match state of a media query.
query
string Media query to parse.function Component() {
const isWidescreen = useMedia('(min-aspect-ratio: 16/9)');
// ...
}
Returns boolean true
if the associated media query list matches the state of the document
, or false
otherwise.
Tracks motion intensity preference of the user.
function Component() {
const preferReducedMotion = useMotionPreference() === 'reduce';
// ...
}
Returns ("no-preference"
| "reduce"
) Preferred motion intensity.
Tracks mouse position.
function Component() {
const [mouseX, mouseY] = useMouseCoords();
// ...
}
Returns Readonly<[number, number]> Coordinates [x, y]
, falling back to [0, 0]
when unavailable.
Tracks information about the network's availability.
ā ļø This attribute is inherently unreliable. A computer can be connected to a network without having internet access.
function Component() {
const isOnline = useNetworkAvailability();
// ...
}
Returns boolean false
if the user agent is definitely offline, or true
if it might be online.
Tracks information about the device's network connection.
āļø The underlying technology is experimental. Please be aware about browser compatibility before using this in production.
function Component() {
const networkInformation = useNetworkInformation();
if (networkInformation) {
const { effectiveType, downlink, rtt, saveData } = networkInformation;
}
// ...
}
Returns (NetworkInformation | undefined) Connection data, or undefined
when unavailable.
Tracks size of an element.
āļø The underlying technology is experimental. Please be aware about browser compatibility before using this in production.
ref
React.RefObject<HTMLElement> Attribute attached to the element under observation.ResizeObserverOverride
TypeOf<ResizeObserver> Replacement for window.ResizeObserver
, e.g. a polyfill.function Component() {
const ref = useRef < HTMLElement > null;
const [width, height] = useSize(ref);
// ...
return <ElementToObserve ref={ref} />;
}
Returns Readonly<[number, number]> Dimensions [width, height]
, falling back to [0, 0]
when unavailable.
Tracks visual viewport scale.
āļø The underlying technology is experimental. Please be aware about browser compatibility before using this in production.
function Component() {
const viewportScale = useViewportScale();
// ...
}
Returns number Pinch-zoom scaling factor, falling back to 0
when unavailable.
Tracks visual viewport scroll position.
āļø The underlying technology is experimental. Please be aware about browser compatibility before using this in production.
function Component() {
const [viewportScrollX, viewportScrollY] = useViewportScrollCoords();
// ...
}
Returns Readonly<[number, number]> Coordinates [x, y]
, falling back to [0, 0]
when unavailable.
Tracks visual viewport size.
āļø The underlying technology is experimental. Please be aware about browser compatibility before using this in production.
function Component() {
const [viewportWidth, viewportHeight] = useViewportSize();
// ...
}
Returns Readonly<[number, number]> Dimensions [width, height]
, falling back to [0, 0]
when unavailable.
Tracks window scroll position.
function Component() {
const [windowScrollX, windowScrollY] = useWindowScrollCoords();
// ...
}
Returns Readonly<[number, number]> Coordinates [x, y]
, falling back to [0, 0]
when unavailable.
Tracks window size.
function Component() {
const [windowWidth, windowHeight] = useWindowSize();
// ...
}
Returns Readonly<[number, number]> Dimensions [width, height]
, falling back to [0, 0]
when unavailable.
useState
hook, which exposes a similar interfaceStores a key/value pair statefully in localStorage
.
key
string Identifier to associate the stored value with.initialValue
(T | function (): T | null) Value used when no item exists with the given key. Lazy initialization is available by using a function which returns the desired value. (optional, default null
)errorCallback
function (error: (DOMException | TypeError)): void? Method to execute in case of an error, e.g. when the storage quota has been exceeded or trying to store a circular data structure.function Component() {
const [visitCount, setVisitCount] =
useLocalStorage < number > ('visitCount', 0);
useEffect(() => {
setVisitCount((count) => count + 1);
}, []);
// ...
}
Returns [T, React.Dispatch<React.SetStateAction<T>>] A statefully stored value, and a function to update it.
useState
hook, which exposes a similar interfaceStores a key/value pair statefully in sessionStorage
.
key
string Identifier to associate the stored value with.initialValue
(T | function (): T | null) Value used when no item exists with the given key. Lazy initialization is available by using a function which returns the desired value. (optional, default null
)errorCallback
function (error: (DOMException | TypeError)): void? Method to execute in case of an error, e.g. when the storage quota has been exceeded or trying to store a circular data structure.function Component() {
const [name, setName] = useSessionStorage < string > ('name', 'Anonymous');
// ...
}
Returns [T, React.Dispatch<React.SetStateAction<T>>] A statefully stored value, and a function to update it.
Listens to an event while the enclosing component is mounted.
target
EventTarget Target to listen on, possibly a DOM element or a remote service connector.type
string Name of event (case-sensitive).callback
EventListener Method to execute whenever the event fires.options
AddEventListenerOptions? Additional listener characteristics.function Component() {
useEventListener(window, 'error', () => {
console.log('A resource failed to load.');
});
// ...
}
Returns void
Repeatedly calls a function with a fixed time delay between each call.
š Timings may be inherently inaccurate, due to the implementation of setInterval
under the hood.
callback
function (): void Method to execute periodically.delayMs
(number | null) Time, in milliseconds, to wait between executions of the specified function. Set to null
for pausing.function Component() {
useInterval(() => {
// Custom logic to execute each second
}, 1000);
// ...
}
Returns void
Avoid layout thrashing by debouncing or throttling high frequency events, e.g. scrolling or mouse movements
Move non-primitive hook parameters to an outer scope or memoize them with useMemo
, e.g.:
const geolocationOptions = { enableHighAccuracy: true };
function Component() {
const geolocation = useGeolocation(geolocationOptions);
// ...
}
FAQs
Essential set of React Hooks for convenient Web API consumption.
The npm package web-api-hooks receives a total of 1,217 weekly downloads. As such, web-api-hooks popularity was classified as popular.
We found that web-api-hooks demonstrated a not healthy version release cadence and project activity because the last version was released a year ago.Ā It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Browserslist-rs now uses static data to reduce binary size by over 1MB, improving memory use and performance for Rust-based frontend tools.
Research
Security News
Eight new malicious Firefox extensions impersonate games, steal OAuth tokens, hijack sessions, and exploit browser permissions to spy on users.
Security News
The official Go SDK for the Model Context Protocol is in development, with a stable, production-ready release expected by August 2025.