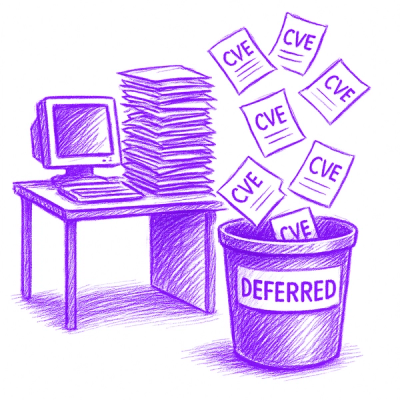
Security News
NVD Quietly Sweeps 100K+ CVEs Into a “Deferred” Black Hole
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
web3-react
Advanced tools
A simple, powerful framework for building modern Ethereum dApps using React.
Documentation for web3-react
is available on Gitbook.
A live demo of web3-react
is available on CodeSandbox.
web3-react
is a simple, powerful framework for building modern Ethereum dApps using React. Its marquee features are:
Full support for commonly used web3 providers, including MetaMask/Trust/Tokenary, Infura/QuikNode, Trezor/Ledger, WalletConnect, Fortmatic/Portis, and more.
A dev-friendly context containing an instantiated ethers.js or web3.js instance, the current account and network id, and more, available globally throughout your dApp via a React Context.
The ability to write custom, fully featured Connectors that manage every aspect of your dApp's connectivity with the Ethereum blockchain and user accounts.
To jump straight into code, check out the CodeSandbox demo!
Ensure you're using the latest react
and react-dom
versions (or anything ^18
):
yarn add react@latest react-dom@latest
Next, you'll have to install ethers.js. If you'd like to use web3.js instead, you can additionally install it (note that ethers.js is still required, as it's an internal dependency to the library).
# required
yarn add ethers
# optional
yarn add web3
Finally you're ready to use web3-react
:
yarn add web3-react@unstable
Now, you'll need to decide how you want users to interact with your dApp. This is almost always with some combination of MetaMask, Infura, Trezor/Ledger, WalletConnect, Fortmatic/Portis, etc. For more details on each of these options, see Connectors.md.
import { Connectors } from 'web3-react'
const { InjectedConnector, NetworkOnlyConnector } = Connectors
const MetaMask = new InjectedConnector({ supportedNetworks: [1, 4] })
const Infura = new NetworkOnlyConnector({
providerURL: 'https://mainnet.infura.io/v3/...'
})
const connectors = { MetaMask, Infura }
Web3Provider
The next step is to setup a Web3Provider
at the root of your dApp. This ensures that children components are able to take advantage of the web3-react
context.
import React from 'react'
import Web3Provider from 'web3-react'
export default function App () {
return (
<Web3Provider
connectors={...}
libraryName={'ethers.js'|'web3.js'|null}
...
>
...
</Web3Provider>
)
}
The Web3Provider
takes 3 props:
connectors: any
(required): An object mapping arbitrary string
connector names to Connector objects (see the previous section for more detail).
libraryName: string
(required): ethers.js
|web3.js
|null
depending on which library you wish to use in your dApp. Passing null
will expose the low-level provider object (you probably don't want this).
web3Api: any
(optional): If you use web3.js
, this prop must be defined, with the value of the default export of web3
(e.g. import Web3 from 'web3'
).
Now, you need to decide how/when you would like to activate your Connectors. For all options, please see the manager functions section. The example code below attempts to automatically activate MetaMask, and falls back to infura.
import React, { useEffect } from 'react'
import { useWeb3Context } from 'web3-react'
// This component must be a child of <App> to have access to the appropriate context
export default function MyComponent () {
const context = useWeb3Context()
useEffect(() => {
context.setFirstValidConnector(['MetaMask', 'Infura'])
}, [])
if (!context.active && !context.error) {
// loading
return ...
} else if (context.error) {
//error
return ...
} else {
// success
return ...
}
}
web3-react
Finally, you're ready to use web3-react
!
The easiest way to use web3-react
is with the useWeb3Context
hook.
import React from 'react'
import { useWeb3Context } from 'web3-react'
function MyComponent() {
const context = useWeb3Context()
return <p>{context.account}</p>
}
To use web3-react
with render props, wrap Components in a Web3Consumer
.
import React from 'react'
import { Web3Consumer } from 'web3-react'
function MyComponent() {
return <Web3Consumer>{context => <p>{context.account}</p>}</Web3Consumer>
}
The component takes 2 props:
recreateOnNetworkChange: boolean
(optional, default true
). A flag that controls whether child components are completely re-initialized upon network changes.
recreateOnAccountChange: boolean
(optional, default true
). A flag that controls whether child components are completely re-initialized upon account changes.
If you must, you can use web3-react
with an HOC.
import React from 'react'
import { withWeb3 } from 'web3-react'
function MyComponent({ web3 }) {
return <p>{web3.account}</p>
}
export default withWeb3(MyComponent)
withWeb3
takes an optional second argument, an object that can set the flags defined above in the render props section.
Regardless of how you access the web3-react
context, it will look like:
{
active: boolean
connectorName?: string
connector?: any
library?: any
networkId?: number
account?: string | null
error: Error | null
setConnector: (connectorName: string, options?: SetConnectorOptions) => Promise<void>
setFirstValidConnector: (connectorNames: string[], options?: SetFirstValidConnectorOptions) => Promise<void>
unsetConnector: () => void
setError: (error: Error, options?: SetFirstValidConnectorOptions) => void
}
active
: A flag indicating whether web3-react
currently has an connector set.connectorName
: The name of the currently active connector.connector
: The currently active connector object.library
: An instantiated ethers.js or web3.js instance (or the low-level provider object).networkId
: The current active network ID.account
: The current active account if one exists.error
: The current active error if one exists.setConnector(connectorName: string, { suppressAndThrowErrors?: boolean, networkId?: number })
: Activates a connector by name. The optional second argument has two keys: suppressAndThrowErrors
(false
by default) that controls whether errors, instead of bubbling up to context.error
, are instead thrown by this function, and networkId
, an optional manual network id passed to the getProvider
method of the connector.setFirstValidConnector(connectorNames: string[], { suppressAndThrowErrors?: boolean, networkIds?: number[] })
: Tries to activate each connector in turn by name. The optional second argument has two keys: suppressAndThrowErrors
(false
by default) that controls whether errors, instead of bubbling up to context.error
, are instead thrown by this function, and networkIds
, optional manual network ids passed to the getProvider
method of the connector in turn.unsetConnector()
: Unsets the currently active connector.setError: (error: Error, { preserveConnector?: boolean, connectorName?: string }) => void
: Sets context.error
, optionally preserving the current connector if preserveConnector
is true
(default true
), or setting a connectorName
(note that if you're doing this, preserveConnector
is ignored).Projects using web3-react
include:
Open a PR to add your project to the list! If you're interested in contributing, check out Contributing-Guidelines.md.
Prior art for web3-react
includes:
A pure Javascript implementation with some of the same goals: web3-webpacked.
A non-Hooks React port of web3-webpacked that had some problems: web3-webpacked-react.
A React library with some of the same goals but that uses the deprecated React Context API and does not use hooks: react-web3.
FAQs
A simple, powerful framework for building modern Ethereum dApps using React.
We found that web3-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
NVD now marks all pre-2018 CVEs as "Deferred," signaling it will no longer enrich older vulnerabilities, further eroding trust in its data.
Research
Security News
Lazarus-linked threat actors expand their npm malware campaign with new RAT loaders, hex obfuscation, and over 5,600 downloads across 11 packages.
Security News
Safari 18.4 adds support for Iterator Helpers and two other TC39 JavaScript features, bringing full cross-browser coverage to key parts of the ECMAScript spec.