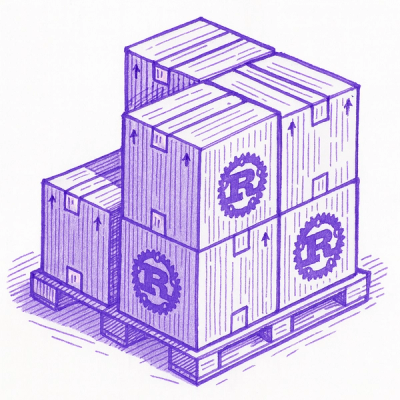
Security News
Crates.io Implements Trusted Publishing Support
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
web3-react
Advanced tools
Web3 React is a drop in solution for building Ethereum dApps in React. Yes, it uses Hooks!
The marquee features of Web3 React are:
A large and fully extensible collection of Connectors, which manage connectivity with the Ethereum blockchain and user account(s). Connectors make your dApp compatible with MetaMask, WalletConnect, Infura, Trust, and more.
A robust management framework for web3. The framework exposes the current account, the current network ID, and an instantiated web3.js instance via a React Context.
A collection of React Hooks that can be used to display ETH and ERC20 balances, sign messages, and more.
A number of utility functions that format Etherscan links, convert token balances, and more.
A complete solution for sending transactions that abstracts away from common annoyances like estimating gas usage, setting a gas price, and checking for transaction errors.
Many of the patterns and APIs described below are shown in this CodeSandbox demo:
Current projects using Web3 React include:
Open a PR to add your project to the list!
First, get the npm package:
npm install web3-react
Next, decide how you want users to interact with your dApp. This could be as simple as . Pick your connectors
Connect
Web3 React exports 3 objects:
Web3Provider
(default export)Web3Context
Provider that ensure children can access the Web3Context
. Web3Provider
takes 4 optional props.Web3Consumer
(named export)Web3Context
Consumer that enables children to access the Web3Context
via a render prop. Web3Consumer
takes 2 optional props.withWeb3
(named export)Web3Context
via an injected web3
prop. withWeb3
takes 1 optional argument.To take advantage of the Web3Context
, Components must have a Web3Provider
parent somewhere in their tree. It's recommended that the Web3Provider
be placed at the top level of your app.
import React from 'react'
import ReactDOM from 'react-dom'
import Web3Provider from 'web3-react'
function App () {
return (
<Web3Provider ...>
...
</Web3Provider>
)
}
const rootElement = document.getElementById("root")
ReactDOM.render(<App />, rootElement)
This ensures that your app will be able to access the Web3Context
, which consists of:
{
library: ...,
account: ...,
networkId: ...,
reRenderers: {...}
connectorName: ...,
activate: ...,
activateAccount: ...,
unsetConnector: ...
}
Note: Web3 React guarantees that while the children
of Web3Provider
are rendered, none of the these context elements are null
or undefined
.
web3js
: A web3.js instance instantiated with the current web3 provider.account
: The current active account.networkId
: The current active network ID.reRenderers
: Variables and functions to facilitate the re-rendering of data derived from account
or networkId
(such as a user's balance, which can change over time without their actual account
changing). For how to use these reRenderers, see src/web3Hooks.js. useAccountEffect
and useNetworkEffect
convenience wrappers for useEffect
are available as named exports of web3-react/hooks
; they take care of re-renders automatically.accountReRenderer
: Forces re-renders of account-derived data.forceAccountReRender
: A function that triggers a global re-render for Hooks depending on accountReRenderer
.networkReRenderer
: Forces re-renders of network-derived data.forceNetworkReRender
: A function that triggers a global re-render for Hooks depending on networkReRenderer
.The easiest way to use Web3 React is with Hooks! All Hooks exported by Web3 React are documented in docs/Hooks.md.
import React from 'react'
import { useWeb3Context, useAccountBalance } from 'web3-react/hooks'
function MyComponent () {
const context = useWeb3Context()
const balance = useAccountBalance()
return (
<>
<p>{context.account}</p>
<p>{balance}</p>
</>
)
}
To access the Web3Context
with a render prop, wrap Components in a Web3Consumer
.
import React from 'react'
import Web3Provider, { Web3Consumer } from 'web3-react'
function AccountComponent (props) {
const { account } = props
return <p>{account}</p>
}
function MyComponent () {
return (
<Web3Consumer>
{context =>
<AccountComponent account={context.account} />
}
</Web3Consumer>
)
}
Note: This pattern will work for arbitrarily deeply nested components. This means that the Web3Consumer
doesn't necessarily need to be at the top level of your app. There also won't be performance concerns if you choose to use multiple Web3Consumer
s at different nesting levels.
If you really have to, you can give Components access to the Web3Context
via a web3
prop by wrapping them with withWeb3
.
import React from 'react'
import { withWeb3 } from 'web3-react'
function MyComponent (props) {
const { web3 } = props
const { account } = web3
return <p>{account}</p>
}
const MyComponentWrapped = withWeb3(MyComponent)
Note: The high-level component which includes your Web3Provider
Component cannot be wrapped with withWeb3
.
Documentation for the utility functions exported by Web3 React can be found separately in docs/Utilities.md.
Documentation for the Hooks exported by Web3 React can be found separately in docs/Hooks.md.
Web3Provider
propsThe Web3Provider
Component takes 5 optional props:
screens
: React Components which will be displayed according to the web3 status of a user's browser. To see the default screens, play around with the CodeSandbox sample app or check out the implementation. Note that these screens are lazily loaded with React.lazy, and therefore are not SSR-compatible.Initializing
: Shown when Web3 React is being initialized. This screen is typically displayed only very briefly, or while requesting one-time account permission.
NoWeb3
: Shown when no injected web3
variable is found.
PermissionNeeded
: Shown when users deny your dApp permission to access their account. Denying access disables the entire dApp unless the accountRequired
flag is set to false
.
UnlockNeeded
: Shown when no account is available.
PermissionNeeded
Component (suggestions welcome).UnsupportedNetwork
: Shown when the user is on a network not in the supportedNetworks
list. The list of supported network IDs is passed to this component in a supportedNetworkIds
prop.
supportedNetworks
list.Web3Error
: Shown whenever an error occurs in the polling process for account and network changes. The error is passed to this Component as an error
prop.
pollTime
: The poll interval for account and network changes (in milliseconds). The current recommendation is to poll for account and network changes.1000
supportedNetworks
: Enforces that the web3 instance is connected to a particular network. If the detected network ID is not in the passed list, the UnsupportedNetwork
screen will be shown. Supported network IDs are: 1
(Mainnet), 3
(Ropsten), 4
(Rinkeby), and 42
(Kovan).[1, 3, 4, 42]
accountRequired
: If false, does not show the UnlockNeeded
screen if a user does not expose any of their accounts.true
children
: React Components to be rendered upon successful Web3 React initialization.Web3Consumer
PropsrecreateOnNetworkChange
. A flag that controls whether child components are freshly re-initialized upon network changes.true
recreateOnAccountChange
. A flag that controls whether child components are freshly re-initialized upon account changes.true
Re-initialization is often the desired behavior, though properly written Hooks should not require these flags to be set.
withWeb3
ArgumentsFlags that can be passed in the second argument to withWeb3
:
recreateOnNetworkChange
. See above.recreateOnAccountChange
. See above.withWeb3(..., { ... })
Prior art for Web3 React includes:
A pure Javascript implementation with some of the same goals: web3-webpacked.
A non-Hooks port of web3-webpacked to React that had some problems: web3-webpacked-react.
A React library with some of the same goals but that unfortunately uses the deprecated React Context API and does not use Hooks: react-web3.
Right now, Web3 React is opinionated in the sense that it relies on web3.js in lieu of possible alternatives like ethjs or ethers.js. In the future, it's possible that this restriction will be relaxed. It's not clear how this would best be achieved, however, so ideas and comments are welcome. Open an issue or PR!
FAQs
A simple, powerful framework for building modern Ethereum dApps using React.
The npm package web3-react receives a total of 108 weekly downloads. As such, web3-react popularity was classified as not popular.
We found that web3-react demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.
Research
/Security News
North Korean threat actors deploy 67 malicious npm packages using the newly discovered XORIndex malware loader.