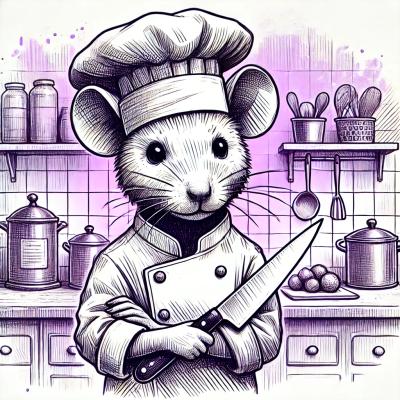
Research
Security News
Quasar RAT Disguised as an npm Package for Detecting Vulnerabilities in Ethereum Smart Contracts
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
which-module
Advanced tools
The which-module npm package is designed to help developers identify the module object for a given request. This is particularly useful in scenarios where you need to know which module is calling a function or when debugging to understand the module dependency tree. It provides a straightforward API to get the module based on a reference to it.
Identifying the module for a given reference
This feature allows developers to find out the module object for a specific reference. In the code sample, `which-module` is used to identify the module object for 'some-module' by accessing it through `require.cache` and `require.resolve`. This can be particularly useful for debugging purposes or when implementing custom module loaders or handlers.
const whichModule = require('which-module');
const moduleInfo = whichModule(require.cache[require.resolve('some-module')]);
console.log(moduleInfo);
The 'resolve' package is similar to 'which-module' in that it helps with module resolution. However, 'resolve' focuses more on asynchronously resolving the path of a module according to the Node.js `require.resolve` algorithm, rather than identifying the module object itself. It's more about finding where a module is located rather than identifying the module's object.
The 'caller' package provides functionality to identify the caller of a function, which can indirectly help in understanding module interactions. While 'which-module' directly identifies the module object for a given reference, 'caller' helps in tracing back the call stack to see which module or function made the current call. This can be useful for debugging or tracking function calls across modules.
Find the module object for something that was require()d
Find the module
object in require.cache
for something that was require()
d
or import
ed - essentially a reverse require()
lookup.
Useful for libs that want to e.g. lookup a filename for a module or submodule
that it did not require()
itself.
npm install --save which-module
const whichModule = require('which-module')
console.log(whichModule(require('something')))
// Module {
// id: '/path/to/project/node_modules/something/index.js',
// exports: [Function],
// parent: ...,
// filename: '/path/to/project/node_modules/something/index.js',
// loaded: true,
// children: [],
// paths: [ '/path/to/project/node_modules/something/node_modules',
// '/path/to/project/node_modules',
// '/path/to/node_modules',
// '/path/node_modules',
// '/node_modules' ] }
whichModule(exported)
Return the module
object,
if any, that represents the given argument in the require.cache
.
exported
can be anything that was previously require()
d or import
ed as a
module, submodule, or dependency - which means exported
is identical to the
module.exports
returned by this method.
If exported
did not come from the exports
of a module
in require.cache
,
then this method returns null
.
ISC © Contributors
2.0.1 (2023-04-21)
<a name="2.0.0"></a>
FAQs
Find the module object for something that was require()d
The npm package which-module receives a total of 8,797,368 weekly downloads. As such, which-module popularity was classified as popular.
We found that which-module demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Research
Security News
Socket researchers uncover a malicious npm package posing as a tool for detecting vulnerabilities in Etherium smart contracts.
Security News
Research
A supply chain attack on Rspack's npm packages injected cryptomining malware, potentially impacting thousands of developers.
Research
Security News
Socket researchers discovered a malware campaign on npm delivering the Skuld infostealer via typosquatted packages, exposing sensitive data.