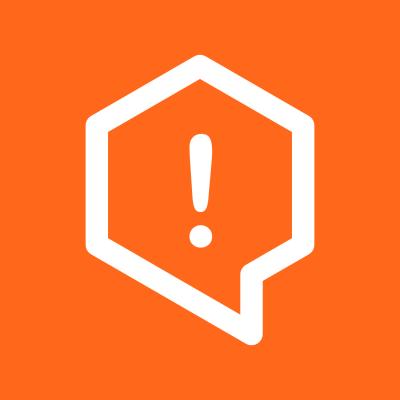
Security News
Fluent Assertions Faces Backlash After Abandoning Open Source Licensing
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
wiremock-rest-client
Advanced tools
Lightweight REST client to interact with a running WireMock server
The WireMock REST client is a lightweight module to interact with a running WireMock server based on the OpenAPI 3.0 spec via REST.
npm install wiremock-rest-client
The WireMockRestClient
has 5 services which correspond with request paths as specified in the OpenAPI spec.
global
- Global operationsmappings
- Operations on stub mappingsrecordings
- Stub mapping record and snapshot functionsrequests
- Logged requests and responses receivedscenarios
- Scenarios support modeling of stateful behaviorSee the API for the available methods. All methods are related to available operations on the WireMock server endpoints.
import { WireMockRestClient } from 'wiremock-rest-client';
const wireMock = new WireMockRestClient('http://localhost:8080');
const stubMappings = await wireMock.mappings.getAllMappings();
console.log(stubMappings);
await wireMock.global.shutdown();
updateGlobalSettings(delayDefinition: DelayDefinition): Promise<void>
resetAll(): Promise<void>
shutdown(): Promise<void>
Example:
await wireMockRestClient.global.resetAll();
getAllMappings(): Promise<StubMappings>
createMapping(stubMapping: StubMapping): Promise<StubMapping>
createMappingFromFile(fileName: string): Promise<StubMapping>
createMappingsFromDir(directoryName: string): Promise<any>
deleteAllMappings(): Promise<void>
resetAllMappings(): Promise<void>
getMapping(uuid: string): Promise<StubMapping>
updateMapping(uuid: string, stubMapping: StubMapping): Promise<StubMapping>
deleteMapping(uuid: string): Promise<void>
saveAllMappings(): Promise<void>
findByMetaData(contentPattern: ContentPattern): Promise<StubMappings>
removeByMetaData(contentPattern: ContentPattern): Promise<void>
Example:
await wireMockRestClient.mappings.resetAllMappings();
const stubMapping = {
"request": {
"method": "GET",
"urlPathPattern": "/api/helloworld"
},
"response": {
"status": 200,
"jsonBody": {"hello": "world"},
"headers": {
"Content-Type": "application/json"
}
}
};
const response = await wireMock.mappings.createMapping(stubMapping);
// Create mapping from current working directory
await wireMock.mappings.createMappingFromFile('stubs/hello-world.json');
// Create mappings from a directory recursively (based on current working directory)
await wireMock.mappings.createMappingsFromDir('stubs');
startRecording(recordSpec: RecordSpec): Promise<void>
stopRecording(): Promise<StubMappings>
getRecordingStatus(): Promise<any>
takeSnapshotRecording(snapshotSpec: RecordSpec): Promise<StubMappings>
Example:
const recordingStatus = wireMockRestClient.recordings.getRecordingStatus();
getAllRequests(): Promise<any>
deleteAllRequests(): Promise<void>
getRequest(uuid: string): Promise<any>
deleteRequest(uuid: string): Promise<void>
resetAllRequests(): Promise<void>
getCount(requestPattern: RequestPattern): Promise<any>
removeRequests(requestPattern: RequestPattern): Promise<any>
removeRequestsByMetadata(contentPattern: ContentPattern): Promise<any>
findRequests(requestPattern: RequestPattern): Promise<any>
getUnmatchedRequests(): Promise<any>
getUnmatchedNearMisses(): Promise<LoggedRequest[]>
getNearMissesByRequest(loggedRequest: LoggedRequest): Promise<any>
getNearMissesByRequestPattern(requestPattern: RequestPattern): Promise<any>
Example:
const requests = await wireMockRestClient.requests.getAllRequests();
getAllScenarios(): Promise<Scenario[]>
resetAllScenarios(): Promise<void>
resetScenario(scenarioId: string): Promise<void>
setScenarioState(scenarioId: string, state: string): Promise<void>
Example:
await wireMockRestClient.scenarios.resetAllScenarios();
The following optional configuration options are available, to be set via options or environment variables.
Configuration option | Default | Options property | Environment variable |
---|---|---|---|
Proxy | No proxy | proxy | WRC_HTTP_PROXY |
Headers | No additional headers | headers | WRC_HEADERS |
Log level | info | logLevel | WRC_LOG_LEVEL |
Continue on failure | false | continueOnFailure | WRC_CONTINUE_ON_FAILURE |
Example using options:
const wireMock = new WireMockRestClient('http://localhost:8080', {
proxy: 'http://mycorporateproxy.com',
headers: {Authorization: 'some-token'},
logLevel: 'debug',
continueOnFailure: true
});
Note: Environment variables have the highest priority
The proxy URL can be HTTP or HTTPS. Credentials for authentication can be passed in the URL.
Example:
WRC_HTTP_PROXY=http://username:secret@mycorporateproxy.com
Additional headers can be added to all HTTP requests.
Example:
WRC_HEADERS="{Authorization: 'some-token'}"
info
debug
will log the request body for each request.A different log level can be configured by setting the environment variable WRC_LOG_LEVEL
to specific a log level (trace
/debug
/info
/warn
/error
/silent
)
2019-12-11T20:43:18.157Z INFO wiremock-rest-client: [Uub8jVUBq91F3MIyKXrON] Request: [POST] http://localhost:8080/__admin/mappings
2019-12-11T20:43:18.157Z DEBUG wiremock-rest-client: [Uub8jVUBq91F3MIyKXrON] Request body: {"request":{"method":"GET","urlPathPattern":"/api/helloworld"},"response":{"status":200,"jsonBody":{"hello":"world"},"headers":{"Content-Type":"application/json"}}}
2019-12-11T20:43:18.158Z INFO wiremock-rest-client: [Uub8jVUBq91F3MIyKXrON] Response: [201] Created
2019-12-11T20:43:18.158Z INFO wiremock-rest-client: [EDYjmman5BLb7tgws-VGg] Request: [POST] http://localhost:8080/__admin/shutdown
2019-12-11T20:43:18.161Z INFO wiremock-rest-client: [EDYjmman5BLb7tgws-VGg] Response: [200] OK
true
A small CLI is available to load data from the command line by passing the folder which contains the stub mappings to be loaded. By default it will reset all stub mappings first (configurable).
$ wrc load --help
Usage: wrc load [options]
Options:
-f, --folders <folders> Comma separated list of folders containing stub mappings to be loaded
--no-reset Skip resetting all stub mappings
-u, --uri [uri] WireMock base URI (default: "http://localhost:8080")
-h, --help display help for command
FAQs
Lightweight REST client to interact with a running WireMock server
The npm package wiremock-rest-client receives a total of 12,442 weekly downloads. As such, wiremock-rest-client popularity was classified as popular.
We found that wiremock-rest-client demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Fluent Assertions is facing backlash after dropping the Apache license for a commercial model, leaving users blindsided and questioning contributor rights.
Research
Security News
Socket researchers uncover the risks of a malicious Python package targeting Discord developers.
Security News
The UK is proposing a bold ban on ransomware payments by public entities to disrupt cybercrime, protect critical services, and lead global cybersecurity efforts.