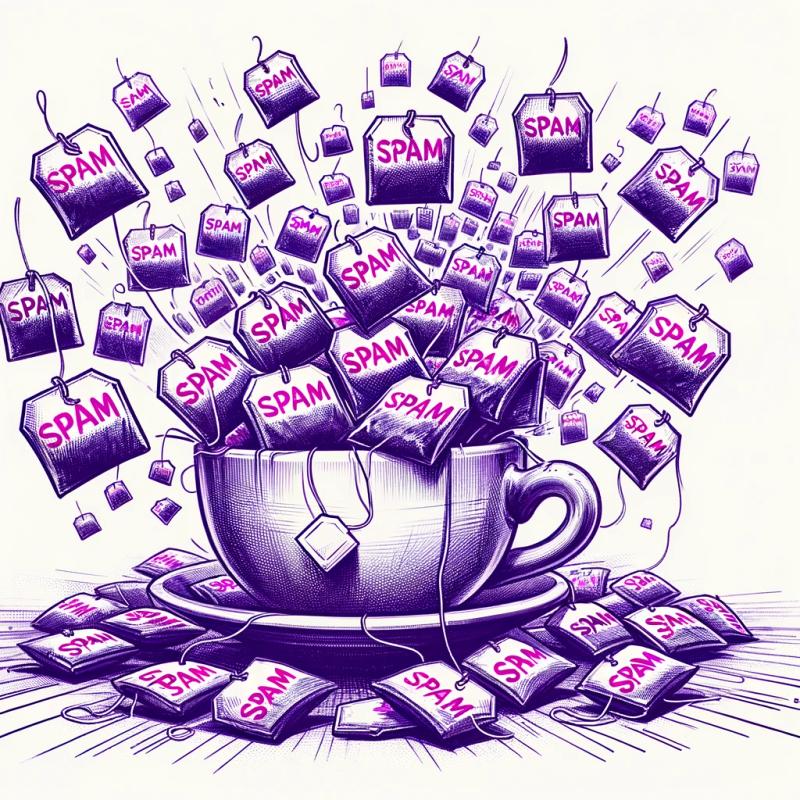
Security News
tea.xyz Spam Plagues npm and RubyGems Package Registries
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
wise-form
Advanced tools
`wise-form` is a React library designed to simplify the creation and management of forms in React applications. By utilizing JSON objects for form configuration, it offers a dynamic approach to form generation, including customizable structures, field o
Readme
wise-form
Librarywise-form
is a React library designed to simplify the creation and management of forms in React applications. By
utilizing JSON objects for form configuration, it offers a dynamic approach to form generation, including customizable
structures, field ordering, and comprehensive validation mechanisms. The library now includes the WrappedForm
component, enabling developers to organize form content within a WiseForm
without using the traditional <form>
tag,
providing greater flexibility in form design and integration.
To add wise-form
to your project, install it via npm:
npm install wise-form
Define a JSON object for your form's configuration. Below is an example structure for a contact form:
// Form structure example
export const form = {
name: 'Contact',
fields: [
{
name: 'email',
type: 'email',
required: true,
label: 'Email',
variant: 'floating',
},
// Add more fields as required
],
};
To render a form, import either WiseForm
or WrappedForm
from wise-form
and pass your form configuration to it:
import React from 'react';
import { WiseForm, WrappedForm } from 'wise-form';
const MyComponent = () => {
return <WiseForm settings={form} />;
// Or use WrappedForm for a form without the <form> tag
// return <WrappedForm settings={form} />;
};
export default MyComponent;
WrappedForm
WrappedForm
allows you to structure your form content within a WiseForm
but without wrapping it inside a <form>
element. This is particularly useful for integrating with other form management libraries or when the form tag is not
desired.
Both WiseForm
and WrappedForm
support a template logic to define the layout and grouping of form fields. This logic
uses a template setting to determine how fields are distributed and displayed. The template configuration can include
specific sizes, patterns, or distributions that are interpreted to organize the form fields into a coherent structure,
enhancing the form's usability and visual appeal.
template
System in wise-form
The template
property in wise-form
offers a flexible way to define the layout of your form. It controls how form
fields are grouped and arranged into rows, providing a straightforward method to customize your form's structure to fit
your UI requirements. This property can accept various configurations, including numbers, strings, and arrays, each
dictating the layout differently. Here's how each variation works:
Number: When the value is a simple number, it represents the number of fields or columns in that row. For
example, a value of 3
means the row will contain three fields.
String: A string value is used to represent a repeated row structure. The format follows the pattern
number x number
, where the first number specifies how many times the row structure will be repeated, and the second
number indicates the number of fields or columns in each of those rows. This allows for the easy repetition of
certain row structures across the form.
Array: An array value provides the most customization, where the first element can be either a number or a string (following the aforementioned logic), and the second element must be a string representing the CSS grid template for each column within that row. This allows for precise control over the layout of each row, including the ability to specify the size and distribution of fields or columns according to CSS grid conventions.
Let's look at a practical example to understand how these variations can be applied:
const formTemplate = [
2, // First row with 2 fields
'2x3', // Two rows, each with 3 fields
[3, '1fr 2fr 1fr'], // A single row with 3 fields, custom grid layout
['2x2', '1fr 1fr'], // Two rows, each with 2 fields, uniform grid layout
];
2
indicates a single row with two fields."2x3"
specifies that there will be two rows, each containing three fields.[3, "1fr 2fr 1fr"]
defines a single row with three fields, where the grid layout for each column
is specified as 1fr 2fr 1fr
, indicating the relative width of each field.["2x2", "1fr 1fr"]
represents two rows, each with two fields, both applying a grid layout of
1fr 1fr
, ensuring each field in the row takes up an equal amount of space.This template
system enables developers to craft forms that are not only functional but also visually aligned with the
application's design, ensuring a seamless user experience. By leveraging numbers, strings, and arrays, the template
property provides the flexibility needed to create diverse and dynamic form layouts.
Here is an example configuration for a login form:
export const loginForm = {
name: 'login',
fields: [
{
name: 'username',
type: 'text',
placeholder: 'Username',
required: true,
label: 'Username',
variant: 'floating',
},
{
name: 'password',
type: 'password',
placeholder: 'Enter your password',
required: true,
label: 'Password',
variant: 'floating',
},
],
};
WiseForm
or WrappedForm
Choose either WiseForm
or WrappedForm
for implementation, depending on your needs. Here's how to implement
WiseForm
:
import { WiseForm } from 'wise-form';
<WiseForm
types={{
select: ReactSelect,
// other custom field types
}}
settings={loginForm}
/>;
For WrappedForm
, the implementation is similar but without the <form>
tag encapsulation.
Contributions to wise-form
are highly appreciated. Please refer to our contribution guidelines for more information.
wise-form
is licensed under the MIT License. For more details, see the LICENSE file.
FAQs
`wise-form` is a React library designed to simplify the creation and management of forms in React applications. By utilizing JSON objects for form configuration, it offers a dynamic approach to form generation, including customizable structures, field o
The npm package wise-form receives a total of 24 weekly downloads. As such, wise-form popularity was classified as not popular.
We found that wise-form demonstrated a healthy version release cadence and project activity because the last version was released less than a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.
Security News
UnitedHealth Group disclosed that the ransomware attack on Change Healthcare compromised protected health information for millions in the U.S., with estimated costs to the company expected to reach $1 billion.