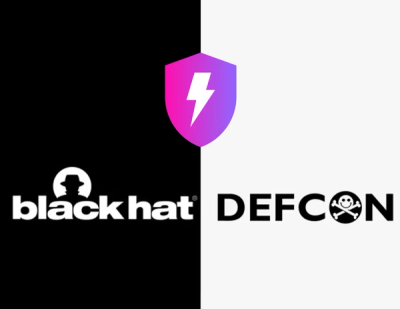
Security News
Meet Socket at Black Hat and DEF CON 2025 in Las Vegas
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Format url with query (string or object), simple and fast, with the power of qs
Supply Chain Security
Vulnerability
Quality
Maintenance
License
Format url with query (string or object), simple and fast, with the power of qs.
The typical usage of this library is building URL for Fetch API. It can be used both on server side and browser side.
const withQuery = require('with-query').default;
fetch(withQuery('https://api.github.com/search/repositories', {
q: 'query',
sort: 'stars',
order: 'asc',
}))
.then(res => res.json())
.then((json) => {
console.info(json);
})
.catch((err) => {
console.error(err);
});
npm install with-query --save
yarn add with-query
var withQuery = require('with-query').default;
var assert = require('assert');
const result1 = withQuery('http://example.com', {
a: 1,
b: 'hello',
});
assert.equal(result1, 'http://example.com?a=1&b=hello');
// Append and override the query in url
const result2 = withQuery('http://example.com?a=3&c=4&d=5', {
a: 1,
b: 'hello',
});
assert.equal(result2, 'http://example.com?a=1&c=4&d=5&b=hello');
// Hash is also supported
const result3 = withQuery('http://example.com?a=3&c=4&d=5#Append', {
a: 1,
b: 'hello',
});
assert.equal(result3, 'http://example.com?a=1&c=4&d=5&b=hello#Append');
// Remove hash
const result4 = withQuery('http://example.com?a=3&c=4&d=5#Append', {
a: 1,
b: 'hello',
}, { noHash: true });
assert.equal(result4, 'http://example.com?a=1&c=4&d=5&b=hello');
// with the power of qs
const result5 = withQuery('http://example.com?e[]=f', {
a: {
b: 'c',
},
});
assert.equal(result5, 'http://example.com?e%5B0%5D=f&a%5Bb%5D=c');
// parseOpt and stringifyOpt for qs.parse and qs.stringify
// see https://github.com/ljharb/qs
const result6 = withQuery('http://example.com&e[]=f', {
a: {
b: 'c',
},
}, {
stringifyOpt: {
encode: false,
},
parseOpt: {
parseArray: false,
},
});
assert.equal(result6, 'http://example.com&e[]=f?a[b]=c');
MIT
FAQs
Format url with query (string or object), simple and fast, with the power of qs
The npm package with-query receives a total of 3,280 weekly downloads. As such, with-query popularity was classified as popular.
We found that with-query demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
Meet Socket at Black Hat & DEF CON 2025 for 1:1s, insider security talks at Allegiant Stadium, and a private dinner with top minds in software supply chain security.
Security News
CAI is a new open source AI framework that automates penetration testing tasks like scanning and exploitation up to 3,600× faster than humans.
Security News
Deno 2.4 brings back bundling, improves dependency updates and telemetry, and makes the runtime more practical for real-world JavaScript projects.