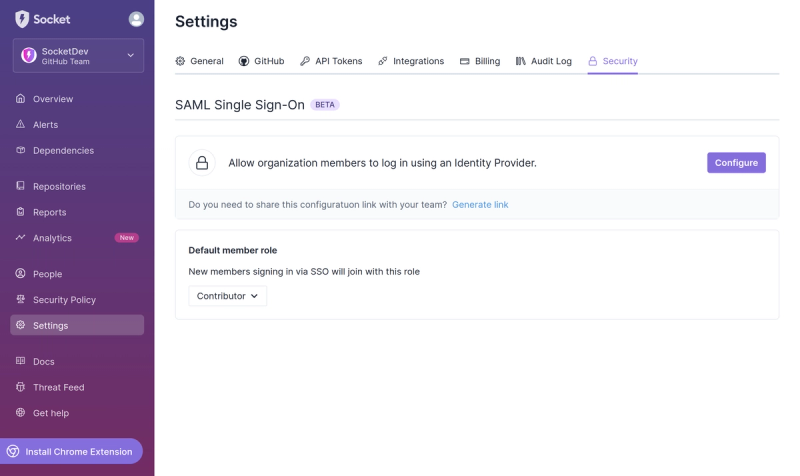
Product
Introducing SSO
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
ws-dependency-mock
Advanced tools
Readme
##ws-dependency-mock
npm i ws-dependency-mock --save
import { IsInt, IsEmail, Min, IsNotEmpty, IsString, MinLength, MaxLength } from 'class-validator';
export class UserModel {
@IsNotEmpty()
@IsInt()
@Min(1)
id: number;
@IsNotEmpty()
@IsString()
@MinLength(2)
@MaxLength(255)
first: string;
@IsNotEmpty()
@IsString()
@MinLength(2)
@MaxLength(255)
last: string;
@IsNotEmpty()
@IsString()
@IsEmail()
email: number;
}
import { AbstractContract } from '../../../src/abstract-contract';
import { UserModel } from '../../../test/src/models/user.model';
export class UserContract extends AbstractContract {
init(): void {
this
.add({
validation: UserModel, // Check validation
payload: { // Your mocked payload
test: 'success',
},
repeat: 1, // Count of repeat. Default: 1
timeout: 500 // Timeout return mock payload. Default: 0
});
}
}
import { ContractInterface } from '../../src/interfaces/contract.interface';
import { ContractNotFoundException } from '../../src/exceptions/contract-not-found.exception';
import { UserContract } from '../../test/src/contracts/user.contract';
export class ContractFactory {
create(key: string): ContractInterface {
switch (key) {
case 'user': return new UserContract();
default: throw new ContractNotFoundException(key);
}
}
}
const wsm = new WebSocketMock({
host: 'localhost',
port: 8080,
});
wsm.run(new ContractFactory());
Enjoy!
FAQs
Library for mock web socket server.
The npm package ws-dependency-mock receives a total of 2 weekly downloads. As such, ws-dependency-mock popularity was classified as not popular.
We found that ws-dependency-mock demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Product
Streamline your login process and enhance security by enabling Single Sign-On (SSO) on the Socket platform, now available for all customers on the Enterprise plan, supporting 20+ identity providers.
Security News
Tea.xyz, a crypto project aimed at rewarding open source contributions, is once again facing backlash due to an influx of spam packages flooding public package registries.
Security News
As cyber threats become more autonomous, AI-powered defenses are crucial for businesses to stay ahead of attackers who can exploit software vulnerabilities at scale.