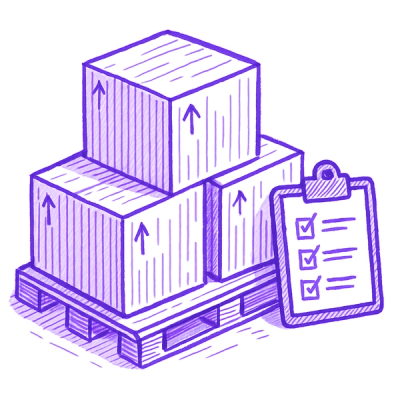
Security News
Open Source Maintainers Feeling the Weight of the EU’s Cyber Resilience Act
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Simple and lightweight Node.js task runner includes source processor and some utilities, which is useful for Front-end developments or Web productions
[klɔ'ks]
Simple and lightweight Node.js task runner includes source processor (SASS, SCSS, Babel, Imagemin etc.), and some utilities (watcher, BrowserSync, FTP client, etc.),
which makes more easy for you to Front-end developments or Web productions,
aiming to be an alternative of laravelmix, gulp, grunt,... and so on.
- Watching file change to call Processors, browserSync, FTP client, etc.
- SASS, SCSS Compile
- postcss
- babel
- Imagemin ( png, jpg, gif, svg, webp)
- BrowserSync
- FTP Client
- Users can register tasks and can call these with CLI command
Option 1. Yarn
$ yarn add -D xocs
Option 2. NPM
$ npm install -D xocs
[npm root]
|-- .env // Append setting templates includes FTP and BrowserSync to '.env'
| // ( will genereate unless already exists )
|-- .browserslistrc // Generate unless '.browserslistrc' already exists
|-- babel.config.js // Generate unless 'babel.config.js' already exists
|-- imagemin.config.js // Generate unless 'imagemin.config.js' already exists
|-- postcss.config.js // Generate unless 'postcss.config.js' already exists
|-- xocs.mix.js // Procedure file ( will genereate unless already exists )
Procedure file's name rule is xocs.***.js
or xocs.***.ts
.
If mutliple procedure files exist, these are prioritized in alphabetical order.
Edit xocs.mix.js
to define source directory root (srcRoot) and build directory root (publicRoot)
const xocs = require('xocs').default;
// Define source directory root (srcRoot) and build directory root (publicRoot)
xocs.init({
srcRoot: 'src',
publicRoot: 'public',
});
/** Add some additional procedures below
* (ex. Start watcher, sass compile, ... etc.)
* .
* .
*/
See below section for how to write additional procedures.
$ npx xocs
Option: Global Install
If you need run CLI command without npx
, install globally in advance
$ npm install -g xocs
$ xocs
const xocs = require('xocs').default;
xocs.init({
env: 'env.production', // Specify your '.env' file name ( Default is '.env')
srcRoot: 'src', // Specify your source directory root ( Default is 'src')
publicRoot: 'public', // Specify your build directory root ( Default is 'public')
});
// Compile scss files specified with 'glob pattern' (!no 'regex pattern')
xocs.sass('src/**/*.scss');
// Proccess with postcss based on options in 'postcss.config.js'
xocs.sass('src/**/*.scss').postcss();
// Proccess with babel based on options in 'babel.config.js'
xocs.babel('src/**/*.js');
// Proccess with postcss based on options in 'postcss.config.js'
xocs.imagemin('src/**/*.@(jpg|jpeg|png|gif|svg|webp)');
xocs.exec('rm -rf .cache');
// Proccess with postcss based on options in 'postcss.config.js'
xocs.watch(['src/**/*.sass', 'src/**/*.scss'], ($) => {
$.sass().postcss();
});
Hear, $
which is a argument of watch property method, has type named Thread
.
Thread
have same functions of xocs
itself except for it includes the information of target files of watcher.
// Init BrowserSync
const browser = xocs.browser();
// Watch files to trigger css compile and browser reload
xocs.watch(['src/**/*.sass', 'src/**/*.scss'], ($) => {
$.sass().postcss();
browser.reload();
});
You can also write as below
// Init BrowserSync
xocs.browser();
// Watch files to trigger css compile and browser reload
xocs.watch('src/**/*.@(sass|scss)', ($) => {
$.sass().postcss().reload();
});
Edit .env
file to path values to process.env
and ignore git staging of .env
with .gitignore
(recommend)
xocs
already includes dotenv module as dependincies
// Create FTP connection
xocs.remote({
host: process.env.FTP_HOST,
port: process.env.FTP_PORT,
user: process.env.FTP_USER,
password: process.env.FTP_PASS,
localRoot: process.env.FTP_LOCAL_ROOT,
remoteRoot: process.env.FTP_REMOTE_ROOT,
});
// Simply copy file from 'src' directory to 'public' directory and upload remote directory
xocs.watch('src/**/*.@(html|php)', ($) => {
$.copy().upload();
});
// Compile css from 'src' directory to 'public' directory and upload remote directory
xocs.watch(['src/**/*.sass', 'src/**/*.scss'], ($) => {
$.sass().postcss().upload();
});
You can also write as below, watching public directory insted of src directory.
// Create FTP connection
xocs.remote({
host: process.env.FTP_HOST,
port: process.env.FTP_PORT,
user: process.env.FTP_USER,
password: process.env.FTP_PASS,
localRoot: process.env.FTP_LOCAL_ROOT,
remoteRoot: process.env.FTP_REMOTE_ROOT,
});
// Watching all files in public directory and upload remote directory
xocs.watch('public/**/*', ($) => {
$.upload();
});
Usage1: Specify tasks from CLI
Register tasks in xocs.mix.js
xocs.task('compile', () => {
xocs.sass('src/**/*.scss').postcss();
xocs.imagemin('src/**/*.@(jpg|jpeg|png|gif|svg|webp)');
});
Run task from CLI
$ npx xocs compile
Usage2: Specify tasks in Procedure
Register tasks in xocs.mix.js
xocs.task('compile:html', () => {
// For example, src/index.html => public/pages/index.html
xocs.watch('src/**/*.@(html|php)', ($, path) => {
$.copy(path, 'pages');
});
});
xocs.task('compile:js', () => {
xocs.watch('src/**/*.js', ($) => {
$.babel();
});
});
xocs.task('compile:css', () => {
xocs.watch('src/**/*.scss', ($) => {
$.sass().postcss();
});
});
xocs.task('compile:img', () => {
xocs.watch('src/**/*.@(jpg|jpeg|png|gif|svg|webp)', ($) => {
$.imagemin();
});
});
xocs.task('preview', () => {
// Start BrowserSync
xocs.browser({
proxy: process.env.PUBLIC_URL,
});
// Create FTP connection
xocs.remote({
host: process.env.FTP_HOST,
port: process.env.FTP_PORT,
user: process.env.FTP_USER,
password: process.env.FTP_PASS,
localRoot: process.env.FTP_LOCAL_ROOT,
remoteRoot: process.env.FTP_REMOTE_ROOT,
});
// Upload file to remote host when files in public directory are updated & run BrowserSync
xocs.watch(['public/assets/**/*', 'public/pages/**/*'], ($) => {
$.upload().reload();
});
});
xocs.run('compile:html', 'compile:js', 'compile:css', 'compile:img', 'preview');
Run task from CLI
$ npx xocs
You can write procudure file in TypeScript replacing extention from .js
to .ts
.
xocs.mix.ts
example is below.
import xocs, { Thread } from 'xocs';
xocs.init({
env: '.env.development', // default is s".env"
srcRoot: 'test/src', // default is "src"
publicRoot: 'test/public', // default is "public"
});
const { srcRoot, publicRoot } = xocs.config;
xocs.watch(srcRoot + '/assets/css/**/*.scss', ($: Thread) => {
$.sass().postcss();
});
xocs.watch(srcRoot + '/assets/img/**/*.@(jpg|jpeg|png|gif|svg|webp)', ($: Thread) => {
$.imagemin();
});
We manage the project version on VERSION.md The versioning scheme we refer is Semantic Versioning.
We always welcome your ideas and feedback. To contribute this project, please see CONTRIBUTING.md at first.
FAQs
Simple and lightweight Node.js task runner includes source processor and some utilities, which is useful for Front-end developments or Web productions
The npm package xocs receives a total of 1 weekly downloads. As such, xocs popularity was classified as not popular.
We found that xocs demonstrated a not healthy version release cadence and project activity because the last version was released a year ago. It has 1 open source maintainer collaborating on the project.
Did you know?
Socket for GitHub automatically highlights issues in each pull request and monitors the health of all your open source dependencies. Discover the contents of your packages and block harmful activity before you install or update your dependencies.
Security News
The EU Cyber Resilience Act is prompting compliance requests that open source maintainers may not be obligated or equipped to handle.
Security News
Crates.io adds Trusted Publishing support, enabling secure GitHub Actions-based crate releases without long-lived API tokens.
Research
/Security News
Undocumented protestware found in 28 npm packages disrupts UI for Russian-language users visiting Russian and Belarusian domains.